Background
Hello gamer! This is my 1st semester project for graduation level that was developed in the Turbo C/C++ graphics. As a member of The Code Project community, I seem to be able to share my project with the game world… :)
Introduction
TIC-TAC-TOE is the most popular and easiest game. It is a two players (X and O) game, where each player taking turns marks the spaces in a 3×3 grid. I have developed this game in TurboC graphics with interfacing keyboard event and have also shown some interactive animation. This tip will be helpful for anyone who wants to develop this type of game using TurboC graphics. So let’s get started…
Requirements
To run and develop TurboC graphics code, you need the following:
- TurboC compiler to execute and run the code.
- Graphic mode functions require a graphics monitor and adapter card such as CGA, EGA and VGA.
- To start graphics programming, you need two files which are GRAPHICS.H and GRAPHICS.LIB. Especially, these files are provided as part of TurboC compile.
Download & Install TurboC
You can download the TurboC++ for Window 7 or 8 in 64bit from the following link:-
After downloading and installing the TurboC++ compiler, you can automatically find the related header file GRAPHICS.H. And the GRAPHICS.LIB file can be found in the following path:
C:\TurboC++\Disk\TurboC3\LIB
It need not be set by you when you run a code. Only you need to set the initgraph()
3rd variable path as follows:
initgraph(&gd, &gm, "c:\\turboc3\\bgi " );
N.B.: Consider that your TurboC++ compiler is installed in the C:\\ TurboC++.
Now put the code \TurboC++\Disk\TurboC3\BIN folder and now open the TurboC++ Editor. Go to File>Open
and browser the path of TIC_TAC_TOE.CPP and open the file.
Start Game
Graphics Environment Starts with the graphics initializes function initgraph()
that loads a graphics driver from disk, then putting the system into graphics mode. It accepts three arguments, one is graphics driver(gd)
, the second is graphics mode(gm) and the third is path for bgi directory that specifies the directory path where initgraph looks for graphics drivers (*.BGI). Here, I have set path c:\\turboc3\\bgi.
Next, I have played an animation text that is write a string
“TIC TAC TOE
” where each character animatedly appears one by one. To animatedly write each letter, a function is called corresponding to the letter. After completing the write “TIC TAC TOE
” string two animations are sparking on two sides of the string
.
void start(){
int gdrive=DETECT,gmode;
initgraph(&gdrive,&gmode,"c:\\turboc3\\bgi");
delay(2000);
T(50,50); delay(100);
I(); delay(100);
C(160,50);delay(100);
T(300,50); delay(100);
A();delay(100);
C(455,50);delay(100);
T(160,200);delay(100);
O();delay(100);
C(250,200);delay(100);
E();delay(200);
for(int q=0;q<5;q++){
SCR(80,235);
SCR(470,235);
CIR(235);
}
closegraph();
}
To write animated letter T
a function T()
is calling that draws a horizontal line and then a vertical line by the circle()
function, where circle()
function draws a circle in x,y coordinate with radius r. If we increase the coordinate value of x and y is constant of parameter of circle()
function, then it will create a horizontal line. Same thing can be applied to draw a vertical line.
void T(int t1,int t2){
for(int i=0;i<70;i++){
setcolor(2);
circle(t1+i,t2,3);
delay(10);
}
for(i=0;i<70;i++){
setcolor(2);
circle(t1+35,t2+i,3);
delay(10);
}
}
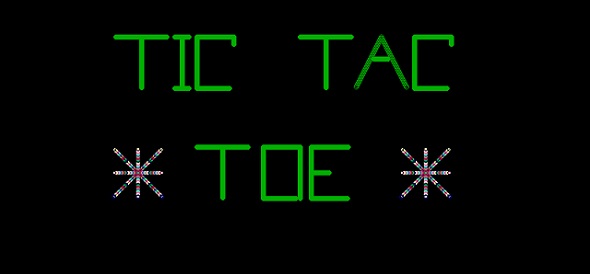
Menu Selection
Now you need to select your options that is 1 for Start the game and 2 for Quit the game. By entering your choice by the standard input/output method, the game will go for the further process. This input is taken by the simple standard I/O function scanf()
. According to the input value, the corresponding operation will be performed by the switch-case
statement.
printf("\n1.Start The Game");
printf("\n2.Quit The Game");
printf("\nEnter your choice(1-2) : ");
scanf("%d",&ch);
switch(ch)
{
case 1:
...
Case 2:
...
}

Enter Player
Enter the player name corresponding to the player X and Player O. Player name will be captured as string
by the gets()
function. Where gets()
function takes the string
input by the standard I/O method.
printf("\nEnter the name of the player playing for \'X\': ");
fflush(stdin);
gets(name_X);
printf("\nEnter the name of the player playing for \'O\': ");
fflush(stdin);
gets(name_O);

Display Board
In this game, two players alternate placing stones of their own color on an m×n board. Board is drawn by the simple printf()
function.
void Board()
{
int i,j;
clrscr();
printf("\n\t\t\t\tTIC TAC TOE BOARD");
printf("\n\t\t\t\t*****************");
printf("\n\n\n");
printf("\n\t\t\t 1\t 2\t 3");
for(i=1;i<=3;i++)
{
printf("\n \t\t\t _____________________________");
printf("\n \t\t\tº\t º\t º\t º");
printf("\n\t\t%d\t",i);
for(j=1;j<=3;j++)
{
if(pos_for_X[i][j]==1)
{
printf(" X");
printf(" ");
}
else if(pos_for_O[i][j]==1)
{
printf(" O");
printf(" ");
}
else
{
printf(" ");
continue;
}
}
printf("\n\t\t\tº\t º\t º\t º");
}
printf("\n\t\t\t------------------------------");
Player_win();
}

Player X Turn
The first player who shall be designated "X
" needs to enter the row and column number. If column or row number is out of range of [1,3] like row<1 or row > 3 or column<1 or column>3
, then it would be a wrong position or else grid position array pos_marked[row][col]
will be assigned 1 according to the row and column number.
void PlayerX()
{
int row,col;
if(win==1)
return;
printf("\nEnter the row no. : ");
fflush(stdin);
scanf("%d",&row);
printf("Enter the column no. : ");
fflush(stdin);
scanf("%d",&col);
if(pos_marked[row][col]==1 || row<1 || row>3 || col<1 || col>3)
{
printf("\nWRONG POSITION!! Press any key.....");
wrong_X=1;
getch();
Board();
}
else
{
pos_for_X[row][col]=1;
pos_marked[row][col]=1;
Board();
}
}
Player O Turn
The second player who shall be designated "O" needs to enter the row and column number. If PlayerO
enters row or column position that is not valid, that means value is out of [1,3] like row<1 or row>3 or column<1 or column>3
then it will be warning through an message “WRONG POSITION!
” or else it will be marked the grid position array pos_marked[row][col]
assign 1 according to the value of row and column value.
void PlayerO()
{
int row,col;
if(win==1)
return;
printf("\nEnter the row no. : ");
scanf("%d",&row);
printf("Enter the column no. : ");
scanf("%d",&col);
if(pos_marked[row][col]==1 || row<1 || row>3 || col<1 || col>3)
{
printf("\nWRONG POSITION!! Press any key....");
wrong_O=1;
getch();
Board();
}
else
{
pos_for_O[row][col]=1;
pos_marked[row][col]=1;
Board();
}
}
Wrong Turn
Wrong turn can be made up of two ways:
- If player gives an entry that cell is already fill-up. That means
pos_marked[row][col]=1
then position will be wrong. - If player gives the row or column number that is out of 3x3 grid. To check if
row<1 or row>3 or col<1 or col>1
then wrong position will happen.
if(pos_marked[row][col]==1 || row<1 || row>3 || col<1 || col>3)
{
printf("\nWRONG POSITION!! Press any key.....");
wrong_X=1;
getch();
Board();
}

Result Check
After each turn of a player, check a calculation that the pos_marked[i][j]=1
where I and j is increment value from 1 to 3 and the value of check(chk
) is increased or else it would be continue
.
void check()
{
int i,j;
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
if(pos_marked[i][j]==1)
chk++;
else
continue;
}
}
}
Win Calculation
Now, it's time to check the result. The player who succeeds in placing three respective marks in a horizontal, vertical, or diagonal row wins the game.
To check the horizontal line for the player x if the pos_for_X[i][1]=1
and pos_for_X[i][2]=1
and pos_for_X[i][3]=1
then playerX
will win where I increment value from 1 to 3. In the same way, I have checked the vertical line and diagonal line. And the code segment is as follows:
void Player_win()
{
int i;
for(i=1;i<=3;i++)
{
if(pos_for_X[i][1]==1 && pos_for_X[i][2]==1 && pos_for_X[i][3]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_X);
printf("\nPress any key............");
return;
}
}
for(i=1;i<=3;i++)
{
if(pos_for_X[1][i]==1 && pos_for_X[2][i]==1 && pos_for_X[3][i]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_X);
printf("\nPress any key............");
return;
}
}
if(pos_for_X[1][1]==1 && pos_for_X[2][2]==1 && pos_for_X[3][3]==1)
{
win=1;
printf("\n\nRESULTL: %s wins!!",name_X);
printf("\nPress any key......");
return;
}
else if(pos_for_X[1][3]==1 && pos_for_X[2][2]==1 && pos_for_X[3][1]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_X);
printf("\nPress any key.....");
return;
}
for(i=1;i<=3;i++)
{
if(pos_for_O[i][1]==1 && pos_for_O[i][2]==1 && pos_for_O[i][3]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_O);
printf("\nPress any key.....");
return;
}
}
for(i=1;i<=3;i++)
{
if(pos_for_O[1][i]==1 && pos_for_O[2][i]==1 && pos_for_O[3][i]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_O);
printf("\nPress any key.....");
return;
}
}
if(pos_for_O[1][1]==1 && pos_for_O[2][2]==1 && pos_for_O[3][3]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_O);
printf("\nPress any key.....");
return;
}
else if(pos_for_O[1][3]==1 && pos_for_O[2][2]==1 && pos_for_O[3][1]==1)
{
win=1;
printf("\n\nRESULT: %s wins!!",name_O);
printf("\nPress any key.....");
return;
}
}

Match Draws
Game will be draws by the following condition:
- If no result is returned from the board against the player X or O, that means if
chk
variable value is 9
. - If no result is returned for the
win
variable, that means if win
variable is not equal to 1
.
if(chk==9)
{
printf("\n\t\t\tMATCH DRAWS");
printf("\nPress any key....");
break;
}
…
if(win!=1)
{
printf("\n\t\t\tMATCH DRAWS!!");
printf("\nPress any key.......");
}

Quit Game
After finishing the game, the following good bye message will be shown. This simple text is present by the printf()
function.

Conclusion
Tic-Tac-Toe is the most popular game. So download the source code and run it to play the game. Happy coding… :)