Introduction
Windows IPC is a communication mechanism which is responsible for sharing data among different applications or processes. According to the purpose, the technique provides data sharing among several processes and also among computers on network. Application can use IPC technique as client-server model. For example, a Windows Forms application wants data from an e-commerce web application that how many customers are visiting to purchase product on a specific moment. The web server is acting as a server to provide runtime information to the forms application. According to the requirement or purpose of IPC, a programmer can decide which type of IPC will be best fit for him. Several IPC mechanisms could be used in a single application to share the data. According to purpose or need, a programmer can choose the IPC process for development. Those indicators mostly depend on data sharing level, performance, nature of the application, etc. In Windows, we can consider a lot of techniques as IPC like Clipboard, COM, Data Copy DDE, File Mapping, Mailslots, Pipes, RPC, Windows Sockets and named shared memory.
Background
The .NET Framework has a lot of good ways to implement inter process communication (IPC) like Web services and Remoting, WCF, etc. The .NET framework also works with MSMQ which is managed by the operating system not the framework. So, MSMQ totally works outside the application’s current AppDomain
which could be a suitable alternative of IPC.
What is Pipe and Named Pipe?
Pipes provide a means for inter process communication. A process that connects to a pipe is a pipe client. One process writes information to the pipe, and then the other process reads the information from the pipe. There are two kinds of pipes and have their own advantages and purpose and those are Anonymous pipe and named pipe. So, we have to determine the type of pipe according to the requirement of the program. Anonymous pipes provide interprocess communication on the same machine using memory. It has less overhead than named pipe. Anonymous pipes are using for communication between threads or between parent and child processes. In this article, I am not going to elaborate anonymous pipes.
On the other hand, a named pipe is a named, one-way or duplex pipe for communication between the pipe server and one or more pipe clients. Every name pipe has its unique name over the network’s name pipe list. So that using the pipe server can be identified by the client to communicate. The named pipe is used for specially sharing data where there is a single server and multiple clients. All clients are using the same pipe name, but each instance has its own buffers.
A client-server mechanism universally makes a peer to peer connection between two processes. For example, a browser creates a connection with a web server to get data according to client’s request. In this article, the term pipe indicates to a process that creates a named pipe and the term pipe clients refers to a process that connects to an instance of a named pipe. Named pipes are used to provide communication channel between processes on the same computer or between processes on different computers across a network. When a server process is running, then all named pipes will be accessible from other computers on network remotely.
A client process will have to mention pipe name by the following way:
\\ServerName\pipe\NameOfThePipe
Server process cannot create a name pipe over the network or remote pc. So, there is no need to mention the server name. So the format of the name in server is
\\.\pipe\NameOfThePipe
Using the Code
The attached source code consists of four projects. One console application contains the server side code of named pipe server. The name of the console application is NamePipeServerTest
. The console server is responsible to run a process and waiting for a client connection. A client project of named pipe is NamedPipeClient
. It's a Windows Form based application, can send the instruction to server and display the processed result. A class library is added for utility type functionality or common functionality for server and client. The name of the utility class library is NamedPipe.Utility
.
After running of the NamePipeServerTest
, a process will run the server and be waiting for the client connection. After receiving and sending data with clients, then the server listen method will be called recursively to accept another connection. When a connection will be established with server, then server will process data. In server side processing, I used a Stream
reader class which reads the file content and sends back the content to client.
Now, we will go through few important classes that are used in this solution. In NamePipeServerTest, the below methods are used to create named pipe in server and process the requests from clients. In this example, I have created a name pipe as "MyTestPipe
" using NamedPipeServerStream
class. In constructor of this class, I pass PipeDirection
as InOut
and also mentioned how many server instances are allowed to wait for the client connection.
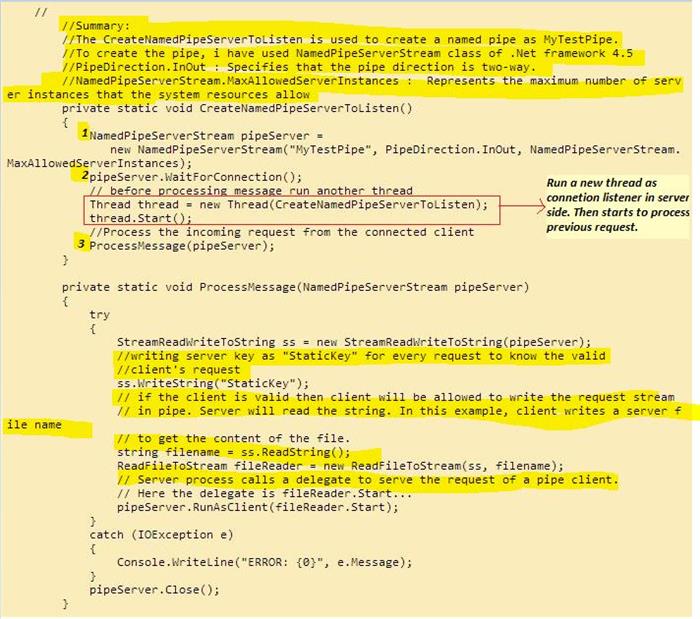
So, from the above comments of code, we know how to create a named pipe server and handle the request. Now, we will concentrate on client side code. To connect or communicate with pipe server, we need to use NamedPipeClientStream
class. The NamedPipeClientStream
class exposes a System.IO.Stream
around a named pipe, which supports both synchronous and asynchronous read
and write
operations. In this example, we are using synchronous read
and write
operations. StreamReadWriteToString
class is used to simply read and write stream
to pipe for both client and server. This class is a part of a common project NamedPipe.Utility
.
private void SubmitToProcessUsingClient(string serverFileName)
{
NamedPipeClientStream pipeClient =
new NamedPipeClientStream(".", "MyTestPipe",
PipeDirection.InOut, PipeOptions.None,
TokenImpersonationLevel.Impersonation);
lstLog.Items.Add("Connecting to server...\n");
pipeClient.Connect();
StreamReadWriteToString ss = new StreamReadWriteToString(pipeClient);
if (ss.ReadString() == "StaticKey")
{
ss.WriteString(serverFileName);
var serverMessage = ss.ReadString();
lstLog.Items.Add(serverMessage);
}
else
{
lstLog.Items.Add("Server could not be verified.");
}
pipeClient.Close();
}
The above code, if (ss.ReadString() == "StaticKey")
line is used to match the server key. Server sends the key to client side to validate the request. If it returns true/code>, then client can write the request <code>stream
to the pipe. After getting the response, the pipeClient
is being closed.
A named pipe server could be a single process instance to process the request. It is a very common scenario for a pipe server needs to communicate with multiple clients. To manage this kind of situation, we have three different approaches to implement. Those are Multithreaded Pipe Server, overlapped and Completion Routines. The most simple mechanism is Multithreaded Pipe Server. Impersonation is also important for named pipe ipc. The <code>ImpersonateNamedPipeClient
function allows the server end of a named pipe to impersonate the client.