Introduction
This tip provides a brief outline of developing a web application in three layer architecture programming style. Three layer architecture is divided into 3 partitions explained below.
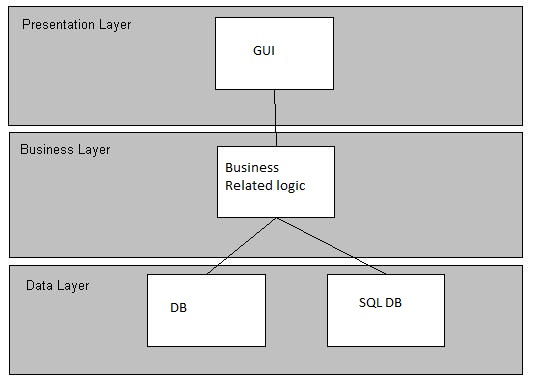
Background
DataAccessLayer
: That is also called DAL layer of the application and provides the performance of all database related activity like Insert Record, Delete Record, Edit Record, and Update Record. This layer provides accessing your data from your database. the Dataset is passed from DAL Layer to Business layer. BusinessLogicLayer
: This layer provides how to add your business related logic in your web application. the business related logic contains the logic of your looping structure (for
, while
, etc.) that perform the All Project Logic in single class file. BL Layer gets the Dataset
which was returned by DAL layer, then converts it into equivalent List (li
) object of that particular file. PresentationLayer
: This layer provides the design at the client side and takes the List which was returned from the business layer, then implements display function, insert function, edit function, etc. which you want to perform. In the image shown below, the three layers are displayed.
Using the Code
- To make application more understandable.
- Easy to maintain, easy to modify application and we can maintain good look of architecture.
Using 3-layer architecture development style, your web application expansion will be easy to implement new changes. In three layer, there are following class files needed to develop the application. The below snapshot displays all three files which you have to add in your application.

Step 1
Add Connection File for creating connection with SQL database. FileName is ClassMain.cs.
Declare two namespaces:
Using system.data;
Using system,data.sqlclient;
Class_Main.CS
SqlConnection cn = new SqlConnection(@"Data Source=JATINKHIMANI-PC\JATINKHIMANI;
Initial Catalog=mydatabase;Integrated Security=True");
Initial Catalog=SaffronyFeedback;Integrated Security=True");
public Class_Main()
{
//
// TODO: Add constructor logic here
//
}
#region QueryFunctions
public void insert(string que)
{
cn.Open();
SqlCommand cmd = new SqlCommand(que,cn);
cmd.ExecuteNonQuery();
cmd.Dispose();
cn.Close();
}
public void delete(string que)
{
cn.Open();
SqlCommand cmd = new SqlCommand(que, cn);
cmd.ExecuteNonQuery();
cmd.Dispose();
cn.Close();
}
public void update(string que)
{
cn.Open();
SqlCommand cmd = new SqlCommand(que, cn);
cmd.ExecuteNonQuery();
cmd.Dispose();
cn.Close();
}
public DataSet select(string que)
{
SqlDataAdapter ad = new SqlDataAdapter(que,cn);
DataSet ds = new DataSet();
ad.Fill(ds);
return ds;
}
#endregion
Step 2
Add another file for DataAccess from Connection file. This is provided to perform database related functions such as insert, update, delete and select.
DL_Marksheet.cs
public DL_Marksheet()
{
}
public static void MarksheetInsert(BL_Marksheet blm)
{
Class_Main cm = new Class_Main();
cm.insert("insert into marksheet(rollno,name,maths,science,english) values
("+blm.Rollno+",'"+blm.Name+"',
"+blm.Maths+","+blm.Science+","+blm.English+")");
}
public static DataSet MarksheetSelect()
{
Class_Main cm = new Class_Main();
DataSet ds = cm.select("select * from marksheet");
return ds;
}
public static DataSet MarksheetSelect(string rollno)
{
Class_Main cm = new Class_Main();
DataSet ds = cm.select("select * from marksheet where rollno="+rollno+"");
return ds;
}
public static void MarksheetDelete(string rollno)
{
Class_Main cm = new Class_Main();
cm.delete("delete from marksheet where rollno=" + rollno + "");
}
Step 3
Add your business related logic for displaying records from database here dataset
converting to List of valid object and then that list is passed to the presentation layer for displaying records.
BL_Marksheet.cs
private int rollno;
private string name;
private int maths;
private int science;
private int english;
public BL_Marksheet()
{
}
public int Rollno
{
get
{
return rollno;
}
set
{
this.rollno = value;
}
}
public string Name
{
get
{
return name;
}
set
{
this.name = value;
}
}
public int Maths
{
get
{
return maths;
}
set
{
this.maths = value;
}
}
public int Science
{
get
{
return science;
}
set
{
this.science = value;
}
}
public int English
{
get
{
return english;
}
set
{
this.english = value;
}
}
public static void MarksheetInsert(BL_Marksheet blm)
{
DL_Marksheet.MarksheetInsert(blm);
}
public static List<BL_Marksheet> MarksheetSelect()
{
List<BL_Marksheet> li = new List<BL_Marksheet>();
Class_Main cm = new Class_Main();
DataSet ds = DL_Marksheet.MarksheetSelect();
for (int i = 0; i <= ds.Tables[0].Rows.Count - 1; i++)
{
BL_Marksheet blm = new BL_Marksheet();
DataRow dr = ds.Tables[0].Rows[i];
blm.Rollno = int.Parse(dr["rollno"].ToString());
blm.Name = dr["name"].ToString();
blm.Maths = int.Parse(dr["maths"].ToString());
blm.Science = int.Parse(dr["science"].ToString());
blm.English = int.Parse(dr["english"].ToString());
li.Add(blm);
}
return li;
}
public static List<BL_Marksheet> MarksheetSelect(string rollno)
{
List<BL_Marksheet> li = new List<BL_Marksheet>();
Class_Main cm = new Class_Main();
DataSet ds=DL_Marksheet.MarksheetSelect(rollno);
for (int i = 0; i <= ds.Tables[0].Rows.Count - 1; i++)
{
BL_Marksheet blm = new BL_Marksheet();
blm.Rollno = int.Parse(ds.Tables[0].Rows[i]["rollno"].ToString());
blm.Name = ds.Tables[0].Rows[i]["name"].ToString();
blm.Maths = int.Parse(ds.Tables[0].Rows[i]["maths"].ToString());
blm.Science = int.Parse(ds.Tables[0].Rows[i]["science"].ToString());
blm.English = int.Parse(ds.Tables[0].Rows[i]["english"].ToString());
li.Add(blm);
}
return li;
}
public static void MarksheetDelete(string rollno)
{
DL_Marksheet.MarksheetDelete(rollno);
}
Step 4
The write code for adding record in presentation file for designing the form of insert record.

<table align="center" border="1" cellpadding="4" cellspacing="1">
<tr>
<td align="justify">
<asp:Label ID="Label1" runat="server" Text="RollNo"></asp:Label>
</td>
<td align="justify">
<asp:TextBox ID="txtrollno" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td align="justify">
<asp:Label ID="Label2" runat="server" Text="Name"></asp:Label>
</td>
<td align="justify">
<asp:TextBox ID="txtname" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td align="justify">
<asp:Label ID="Label3" runat="server" Text="MAths"></asp:Label>
</td>
<td align="justify">
<asp:TextBox ID="txtmaths" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td align="justify">
<asp:Label ID="Label4" runat="server" Text="Science"></asp:Label>
</td>
<td align="justify">
<asp:TextBox ID="txtscience" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td align="justify">
<asp:Label ID="Label5" runat="server" Text="English"></asp:Label>
</td>
<td align="justify">
<asp:TextBox ID="txtenglish" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td align="justify" colspan="2">
<asp:Button ID="btnsubmit" runat="server" onclick="btnsubmit_Click"
Text="SUBMIT" Width="100px" />
</td>
</tr>
<tr>
<td align="justify" colspan="2">
<asp:GridView ID="gridmarksheet" runat="server" AutoGenerateColumns="False"
BackColor="White" BorderColor="#DEDFDE" BorderStyle="None" BorderWidth="1px"
CellPadding="4" ForeColor="Black" GridLines="Vertical">
<AlternatingRowStyle BackColor="White" />
<Columns>
<asp:BoundField DataField="rollno" HeaderText="RollNo" />
<asp:BoundField DataField="name" HeaderText="Name" />
<asp:BoundField DataField="maths" HeaderText="Maths" />
<asp:BoundField DataField="science" HeaderText="Science" />
<asp:BoundField DataField="english" HeaderText="English" />
</Columns>
<FooterStyle BackColor="#CCCC99" />
<HeaderStyle BackColor="#6B696B" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#F7F7DE" ForeColor="Black" HorizontalAlign="Right" />
<RowStyle BackColor="#F7F7DE" />
<SelectedRowStyle BackColor="#CE5D5A" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FBFBF2" />
<SortedAscendingHeaderStyle BackColor="#848384" />
<SortedDescendingCellStyle BackColor="#EAEAD3" />
<SortedDescendingHeaderStyle BackColor="#575357" />
</asp:GridView>
</td>
</tr>
</table>
Step 5
Add below cs code to Presentation file .cs form. Here, I run the insert and display record data if you want to edit & delete that display data, just call function which I have already written into BLMarksheet.CS file.
Class_Main cm = new Class_Main();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
DisplayData();
}
}
public void DisplayData()
{
List<BL_Marksheet> li = new List<BL_Marksheet>();
li = BL_Marksheet.MarksheetSelect();
gridmarksheet.DataSource = li;
gridmarksheet.DataBind();
}
protected void btnsubmit_Click(object sender, EventArgs e)
{
try
{
BL_Marksheet blm = new BL_Marksheet();
blm.Rollno = int.Parse(txtrollno.Text);
blm.Name = txtname.Text;
blm.Maths = int.Parse(txtmaths.Text);
blm.Science = int.Parse(txtscience.Text);
blm.English = int.Parse(txtenglish.Text);
DL_Marksheet.MarksheetInsert(blm);
DisplayData();
Response.Write("<script>alert('Record Inserted...')</script>");
Clear();
}
catch (Exception)
{
Response.Write("<script>alert('Try Again...')</script>");
Clear();
}
}
public void Clear()
{
txtrollno.Text = "";
txtname.Text = "";
txtmaths.Text = "";
txtscience.Text = "";
txtenglish.Text = "";
txtrollno.Focus();
}
I have made changes in the above code and if you want to see, then download the demo code which I have uploaded.
Points of Interest
There are many types of other 3-layer code styles available in many articles, but I think that is very efficient and contains the least amount of code to implement 3-layer architecture. This is very useful for beginners who aren't aware about the new code style in ASP.NET. There are many new developers that are struggling to develop it so, I have done it and publish it for all.
History
- 19th January, 2015: First version
Thank you!!
Jatin Khimani
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.