Introduction
This tip is a simple Facebook app in Windows 8.1 using free tools like Microsoft Visual Studio 2013 Community Edition. It will guide you from installing a Facebook app through programming the C# SDK for Facebook. It's a Windows Store style app.
Background
I checked some Facebook application examples but none satisfied what I wanted and that is the new Facebook asynchronous C# SDK. Knowledge of C# and XAML is required, in particular, the await async
keywords.
Using the Code
Download the source file and build it using MSVS 2013 community edition which is free. There is no binary file since it must be installed in the store or on your computer through MSVS.
Installing a Facebook Desktop App
- Go to Tools->nuget package manager -> nuget console
to install Facebook.dll and FacebookClient.dll type this: Install-Package Facebook.Client - Right click references to add a reference to facebook.dll and facebook.client.dll
- Go to developers.facebook.com-> myapps -> add a new app (like in figure 1)
- Select a name and click advanced
- Click settings
- Click advanced then native or desktop app (like figure 2)
- (very important) Select Embedded browser and OAuth Login (like in figure 3)
- Click yes, then save at the bottom
- Add an XML file named facebookconfig.xml to the main folder of your project, it looks like this:
="1.0"="utf-8"
<Extensions>
<Facebook AppId="your app id here" />
<Extensions>
<xml>
That's it. This is the only place we will need the AppID
, the AppSecret
will not be needed.
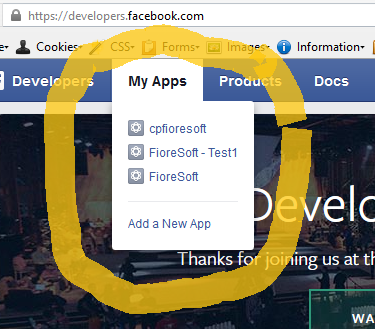
Fig 1

Fig 2

Fig 3
Updating the MainPage.cs and MainPage.xaml
Add to MainPage.cs:
using Facebook;
using Facebook.Client;
using System.Threading.Tasks;
using System.Diagnostics;
Here is the method to get user information:
private async static Task<dynamic> GetUser(String token)
{
var client = new FacebookClient();
dynamic user = await client.GetTaskAsync("/me",
new { fields = "first_name,last_name,gender,locale,id,name,email,picture,location",
access_token = token });
return user;
}
Add to your XAML file xmlns:fbc ="using:Facebook.Client.Controls”
. Between the grid, add this:
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel>
<fbc:LoginButton Name="fbclb" />
<TextBox Name="tbUser" />
<Button Name="btSend" Content="get user" Click="OnButtonUser" />
<Button Name="btPublish" Content="Publish" Click="OnButtonPublish" />
<fbc:FriendPicker Name="fbcfp" />
<fbc:ProfilePicture />
<fbc:PlacePicker />
</StackPanel>
</Grid>
We added a login button, a friend picker, a profile picture and a place picker control. The friend picker doesn't really work anymore, only users who are your friends and are connected to the same app as you, will be added. But we add it anyway so we can easily get the AccessToken
. The login button does all the hard work to authenticate into Facebook.
The functions GetUser
and PublishStream
were adapted from this article on MSDN magazine.
Additional Information
There is a very nice tool in Facebook to explore the so called "graph API" ( a series of get or post http elements). You can try it here.