Uploading Files and Folders Recursively to a SharePoint Site






4.64/5 (10 votes)
This article demonstrates how to upload files and folders recursively from a local folder to Microsoft SharePoint Foundation site using the Object Model.
Introduction
You can upload documents to SharePoint libraries using the Object Model or SharePoint Webservices and this article about how to upload a file and folders recursively from a local folder to a folder on a Microsoft SharePoint Foundation site using the Object Model.

Background
I was assigned a project to Migrate DOORS (Dynamic Object-Oriented Requirements System) data into the TFS 2010 SharePoint portal server. The DXL script is take care of the extraction part and stored the files into the local folders. Then I Googled around to find a way to upload files recursively to SharePoint server, but no use. So I decided to do some research on it and came up with this code.
Microsoft.SharePoint
The Microsoft.SharePoint.dll is used to access the Sharepoint object model.
Code for Uploading File to Document Library
try
{
localPath = txtLocalPath.Text; //Local path.
sharePointSite = txtServerURL.Text; //SharePoint site URL.
documentLibraryName = txtLibrary.Text; // SharePoint library name.
// Make an object of your SharePoint site.
using (SPSite oSite = new SPSite(sharePointSite))
{
oWeb = oSite.OpenWeb();
CreateDirectories(localPath,
oWeb.Folders[documentLibraryName].SubFolders);
}
}
catch (Exception ex)
{
MessageBox.Show("Error:" + ex.Message );
}
CreateDirectories(string path, SPFolderCollection oFolderCollection)
The CreateDirectories
method is a recursive method which accepts two parameters. The path
parameter specifies the path of the source location in the file system of the local computer, and the oFolderCollection
parameter specifies the folder collection of the destination.
private void CreateDirectories(string path, SPFolderCollection oFolderCollection)
{
//Upload Multiple Files
foreach (FileInfo oFI in new DirectoryInfo(path).GetFiles())
{
FileStream fileStream = File.OpenRead(oFI.FullName);
SPFile spfile = oFolderCollection.Folder.Files.Add
(oFI.Name, fileStream, true);
spfile.Update();
}
//Upload Multiple Folders
foreach (DirectoryInfo oDI in new DirectoryInfo(path).GetDirectories())
{
string sFolderName = oDI.FullName.Split('\\')
[oDI.FullName.Split('\\').Length - 1];
SPFolder spNewFolder = oFolderCollection.Add(sFolderName);
spNewFolder.Update();
//Recursive call to create child folder
CreateDirectories(oDI.FullName, spNewFolder.SubFolders);
}
}
Local Folder

SharePoint Library
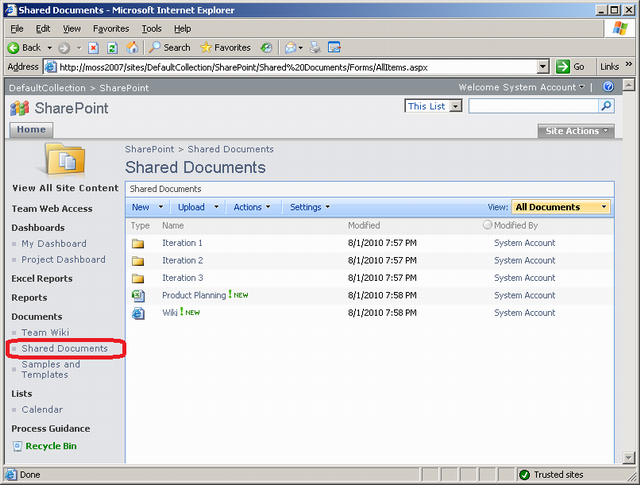
Output

Limitations
- The size of the file that is uploaded cannot exceed 2 GB.
- For all this code to execute, current logged in user should have create and upload, delete permissions at respective document library.
History
- 10th August, 2010: Initial post