Introduction
Google maps are great. Making them easier to work with for developers is the jQuery gomap plugin.
Using this, and a nice list of the central latitudes / longitudes for each USA State from here, I was able to create an HTML page that allows the user to select any of the 50 U.S. States and have that State centered in the browser.
Note: An example of a live web site that uses this functionality is my site USA Map-O-Rama, which allows the user to populate a map with markers showing the locations of NFL and MLB teams, State capitals, National Parks, as well as the hometowns of many authors and musicians / bands.
The Setup
After downloading the gomap file, and saving it to the same location as the .html file I was building, I added this code to the head
section to access jQuery, Google Maps, and the gomap plugin:
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<script src="http://maps.google.com/maps/api/js?sensor=false"></script>
<script src="gomap.js"></script>
The HTML
I then added input selections like so (the "50+" refers to the fact that at that zoom level (4), other U.S. territories can be seen in addition to "just" the 50 states):
<select id="stateSelector">
<option id="USA48">USA (48)</option>
<option id="USA50">USA (50+)</option>
<option id="Alabama">Alabama</option>
<option id="Alaska">Alaska</option>
. . .
<option id="Wisconsin">Wisconsin</option>
<option id="Wyoming">Wyoming</option>
</select>
...and the div
for the map:
<div id="map"></div>
The jQuery
Then I added the gomap initialization inside the jQuery ready handler (which the user can revert back to by selecting "USA (48)"):
$("#map").goMap({
latitude: 36.75,
longitude: -100,
maptype: 'ROADMAP',
zoom: 5
});
If you prefer to use a different type of map (other than ROADMAP
, shown above), you could replace that value with SATELLITE
, HYBRID
, or TERRAIN
. Experimentation is your friend!
Then the change handler for the input selection:
$('#stateSelector').on("change",function() {
var $this = $(this).val();
if ($this == "Alabama")
{
LoadAlabama();
}
else if ($this == "USA (48)")
{
LoadUSA48();
}
else if ($this == "USA (50+)")
{
LoadUSA50();
}
else if ($this == "Alaska")
{
LoadAlaska();
}
. . .
else if ($this == "Wisconsin")
{
LoadWisconsin();
}
else if ($this == "Wyoming")
{
LoadWyoming();
}
});
...and finally the functions called by the change handler; one example should suffice:
function LoadAlaska() {
$("#map").removeData();
$("#map").goMap({
latitude: 61.370717,
longitude: -152.404420,
zoom: 5
});
}
Here is an example, showing what displays when the user selects the Aloha State from the input selector:
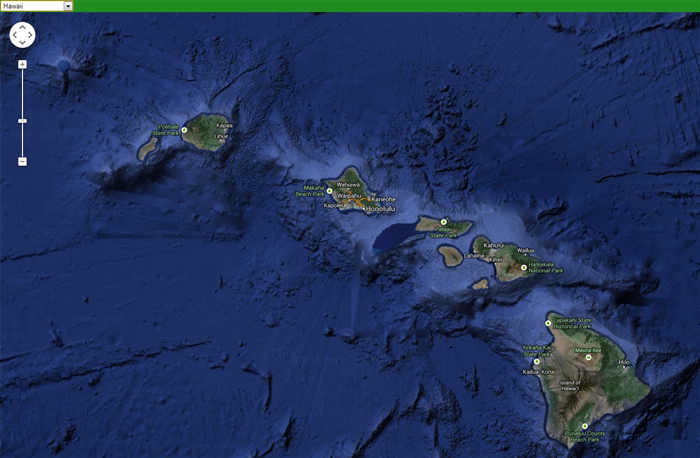
All the code is provided in the file for download, along with the gomap file; those two files are all you need - just double-click the HTML file, and voila! You will see this idea realized in all its presumptive glory.
This is a simple example of programmatically moving around a map; much more exciting things could be done by dynamically loading data each time the map is redrawn, such as to draw markers on the map, which can be done this way:
$.goMap.createMarker({
address: 'Green Bay, Wisconsin',
title: 'Lambeau Field, Green Bay, WI',
html: '<h1>Green Bay Packers [add any html you want here]</h1>'
});
So Long, It's Been Good to Know Ya! (Don't forget Woody!)
You could initiate the map showing a State, and allow the user to focus in on Counties; or you could start with the USA (as in this example) and, on selecting a State, load markers for all State Parks - or for the locations of all your businesses customers, or your contacts, etc.; you could also begin with a world map, allow the user to select first the continent they are interested in, then the country, and from there possibly a State, Province, or Region. The possibilities are virtually unlimited.
Data to use to populate the markers could come from a database, a json file, a web service/REST method, etc. Again, the possibilities that this technique hint at are almost mind-boggling to contemplate.
If your data contains latitudes and longitudes, you could query it (via LINQ or whatever works in your situation) to return just those records that fall within the boundaries of the map currently being displayed. By taking into account the longitude and latitude* being used to set the center of the map, along with the zoom level, you can determine the East/West (longitudinal) and North/South (latitudinal) limits of the world map subset currently being shown and only render the markers that will actually be seen at that projection.
Here's what I mean about only creating markers for addresses which are currently within the area the browser is displaying: You cannot know programmatically whether "Mokelumne Hill, California" is within the confines of the map as it is currently centered and scaled; however, you can calculate whether a latitude of 38.3004709 and longitude of -120.7063219 are within the bounds.
Once you know how many degrees of longitude and meridians of latitude a map displays at a given zoom factor, you can "do the math" to see whether a given long/lat pair should be represented on the map with a marker.
The methodology to making this happen, provided you have data with addresses in it, is to convert the addresses to their lat/long equivalents, store them in a separate table (related to the first one, perhaps with the original table's primary key value as a foreign key in your LatLong lookup table), and then query that table as the map is redrawn.
* As Jimmy Buffett sang, changes in latitudes can bring about changes in attitudes.
I am in the process of morphing from a software developer into a portrayer of Mark Twain. My monologue (or one-man play, entitled "The Adventures of Mark Twain: As Told By Himself" and set in 1896) features Twain giving an overview of his life up till then. The performance includes the relating of interesting experiences and humorous anecdotes from Twain's boyhood and youth, his time as a riverboat pilot, his wild and woolly adventures in the Territory of Nevada and California, and experiences as a writer and world traveler, including recollections of meetings with many of the famous and powerful of the 19th century - royalty, business magnates, fellow authors, as well as intimate glimpses into his home life (his parents, siblings, wife, and children).
Peripatetic and picaresque, I have lived in eight states; specifically, besides my native California (where I was born and where I now again reside) in chronological order: New York, Montana, Alaska, Oklahoma, Wisconsin, Idaho, and Missouri.
I am also a writer of both fiction (for which I use a nom de plume, "Blackbird Crow Raven", as a nod to my Native American heritage - I am "½ Cowboy, ½ Indian") and nonfiction, including a two-volume social and cultural history of the U.S. which covers important events from 1620-2006: http://www.lulu.com/spotlight/blackbirdcraven