Introduction
We know that Dependency injection is a software design pattern which talks about the dependency between software modules. It’s everyone’s goal to implement de-couple and less dependency modules. Angular is a JavaScript framework where dependency injection is handled very smoothly and we can design each and every module in Angular without depending on other modules and this is the beauty of AngujarJS framework.
In this tip, we will discuss dependency injection in controller of AngularJS . If you are very new in AngularJS, then I will suggest you to go through the documentation of AngularJS before continuing with this tip.
We know that AnjularJS is great MVC framework in client which truly separates data, business logic and presentation logic into different modules. The controller is responsible for implementing business logic which often takes data from service (service in angular) and push to view. So, in general, Controller is the glue between model and view.
Those who are server side developers (like C# or Java programmers) might be aware of Dependency injection and the various ways to implement it. Yes, I am talking about controller injection, property injection and function injection but dependency injection in Angular is a bit different than this. We will see it in practice.
Dependency Injection in Controller of AngularJS
Let’s start with baby steps, we will implement the view at first, which is nothing but a simple HTML page like below and give reference of Angular library. In this example, Dependency.js is my external js file where I will define controller, you are free to define controller in the same page if you wish.
<html ng-app>
<head>
<script src="angular.min.js" type="text/javascript"></script>
<script src="Dependency.js" type="text/javascript"></script>
</head>
<body ng-controller="myController">
{{message}}
</body>
</html>
Let’s Implement Controller
Yes, as we know, controller in AngularJS is nothing but normal JavaScript function and here the controller is dependent on $scope
because within controller we are setting property of $scope
object. Now, the beauty of the controller is that we are injecting $scope
to the controller externally.
function myController($scope) {
$scope.message = 'Welcome in AngularJS';
}
Angular's Dependency Injection allows you to write code like the following without taking into account where $scope
comes from. In this case, $scope
gets injected by Angular whenever this controller is instantiated.
Angular uses this approach because it allows for a clear separation of concerns and for a higher degree of focused unit-testing. You can for example configure the DI framework to use mock-objects for underlying components instead of real services during your unit tests. This approach allows you to focus on testing only one particular piece of functionality - one unit - instead of testing all the underlying services as well.
Once we run the application , we will see the below output.
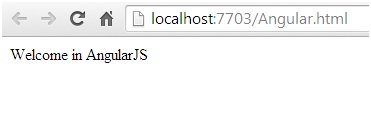
Create Controller and Attach it to Module
The previous controller implementation is much similar to the class JavaScript function, but there is an official way to define controller in AngularJS. In this example, we will create module at first and then we will attach controller to this module and we will inject same $scope
to the controller. Here is the view implementation.
We have just added ng –app directive in page which will bootstrap the Angular framework in document.

Here, we are creating module at first:
var app = angular.module('myApp', []);
and we are attaching ‘myController
’ controller with the module and injecting $scope
to the controller.
app.controller('myController',['$scope', function ($scope) {
$scope.message = 'Welcome in AngularJS';
}]);
Inject Angular Service to Controller
In this example, we will create one simple service in AngularJS and then we will inject the service to controller. So, let’s create service at first. Have a look at the below code.
var app = angular.module('myApp', []);
//Implement and attach service to module
app.service('dataService', function () {
var message = function () {
return 'Data from service';
};
return {
message:message
};
});
Now, we will create controller and we will inject service to the controller. Within controller , we are just retrieving message from service and setting to scope.
So, in this controller, we are injecting two objects; one is $scope
and another is dataService
.
//Controller implementation
//inject dataService to controller
app.controller('myController', ['$scope', 'dataService', function ($scope, dataService) {
$scope.message = dataService.message();
}]);
Once we run the application, we should see the below message:

Inject Factory to AngularJS Controller
This is very similar to service injection. In this example, we will inject factory to controller. Here is the implementation of factory. It’s very simple and we have added two message sending functions within messageFactory
.
var app = angular.module('myApp', []);
app.factory('messageFactory', function () {
var alretMessage = function () {
return 'Alert message';
};
var warningMessage = function () {
return 'Warning message';
};
return {
alretMessage : alretMessage,
warningMessage : warningMessage
};
});
In the same way, we can inject factory to controller. Have a look at the below code.
app.controller('myController', ['$scope', 'messageFactory', function ($scope, messageFactory) {
$scope.message = messageFactory.alretMessage() + ' , ' + messageFactory.warningMessage();
}]);
And here is the output message:

Border Line
In this example, we have seen how to implement dependency injection within controller of AngularJS. Normally, every component in AngularJS is very much de-coupled and pluggable. I can say that strong dependency injection made AngularJS one of the most popular front end frameworks.
I am software developer from INDIA. Beside my day to day development work, i like to learn new technologies to update myself. I am passionate blogger and author in various technical community including dotnetfunda.com , c-sharpcorner.com and codeproject. My area of interest is modern web technology in Microsoft stack. Visit to my personal blog here.
http://ctrlcvprogrammer.blogspot.in/