Here is the code for this initial release of the framework on GitHub.
1. Introduction
In a previous guide, we explored Playwright's Codegen tool to generate test cases for the NUnit framework using C#. The generated test cases were executed out of the box, but they lacked abstractions and presented some maintenance challenges due to hard-coded values that are present throughout the test cases. In this guide, we leverage Codegen again, but this time, we use ChatGPT to build a well-structured, readable, and maintainable testing framework using the Page Object Model (POM). While our examples focus on C# and NUnit, the principles discussed are adaptable to other technologies like Java and TestNG, ensuring flexibility and scalability in automation efforts
2. Manual Test Case
Before diving into automation, it is recommended to start with a manual test case. Let us consider a scenario where a user performs a quick smoke test to verify links on the home page of an ecommerce website. In this example, we use the following URL: “https://demo.nopcommerce.com”
3. Recording the test case
By analyzing the steps outlined in the manual test case, we can identify some of the pages for the application. Each user action described in the manual test case navigates the user to a different page, namely, “Homepage”, “Computers”, "Electronics", “Registration”, and “Login”.
Our first task towards building the framework is to identify the locators for elements on this page, leveraging Playwright's Codegen tool.
Codegen allows us to record user interactions and generates code snippets based on those interactions. To run Codegen from the command line, with the URL of the application under test, we can start the Playwright inspector tool with the following command.
This command opens the “Playwright inspector” and the browser side by side, as shown in Figure 1. and Figure 2.
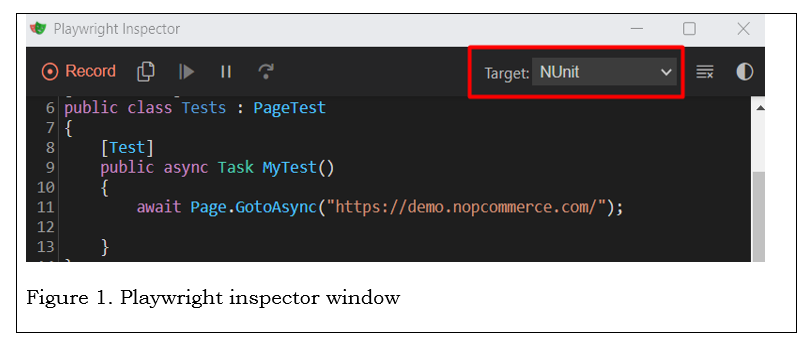

Our primary objective of using Codegen is to identify the locators for the pages under construction. By convention, we use “Page_<Name> for the names of the pages, so our home page is called “Page_Home”.
4. Identify home page locators
Here are the complete steps for identifying the locators for the home page using the functionality of Codegen. In this phase, we make use of the “Record Button” and the “Assert Visibility” button.

- Click the record button on the browser window
- Click in the “Assert Visibility” button
- Click on the URL (https://demo.nopcommerce.com/) in the address bar.
Notice how the state of the “Assert Visibility” button changes momentarily when we click on the element we are asserting, like the address bar in this case. If you have a fast computer, you may not see the change!

- Once the “Assert Visibility” button is back to its original state, click on it again, then click on “Registration”, then wait until the icon goes back to its original state.
- Click on “Log in”, then wait until the icon goes back to its original state.
- Click on “Computers”, then wait until the icon goes back to its original state.
- Finally, click on “Electronics”, then wait until the icon goes back to its original state.
Here are the results of our interaction with the page for the NUnit target settings.

5. Building our first page object
One of the challenges we face when creating an automation framework using POM is, ironically, the creation of the objects themselves. These challenges include building the initial set of objects, refining them iteratively, and continually expanding the collection of objects as more tests are integrated into the framework. To streamline this process, we have opted to leverage ChatGPT to help us accelerate this process.
Notice that the generated code, from Codegen, for this example has a couple of issues, which we need to fix before moving forward. (Author note: I am not sure if these are codegen bugs or just a display issue on my end.)
- Line 11 in Figure 5 is missing the statement to create a “page” instance. The line should have been:
var page = await context.NewPageAsync();
- The lines starting with “await Expect(page…” should have been
"await Assertions.Expect (page…"
Anyway, these issues present us with an opportunity to challenge ChatGPT.
To get started with “ChatGPT”, let us issue the following prompt. It is a bit long, but I wanted to investigate how far I can push ChatGPT.

A few seconds later, we get the following response.

The test case generated by ChatGPT for this test case was correct and complete. I made a few formatting changes. Other than that, I copied it into Visual Studio and executed it.
Our next task is to generate a page object for this test case. Here is the ChatGPT prompt that I used.
- Create a page object for the test case "VerifyPageElementsAreVisible".
- Use “Page_Home” for the class name
- Use Pages_generated_chatgpt for the namespace
ChatGPT generated the desired, as expected. Overall, we can use ChatGPT as a senior consultant looking over our shoulders, as we iterate through the lifecycle of creating the framework.
6. Create an NUnit/C# project
At this point, we are ready to put our efforts into action.
- Start Visual Studio
- Create an NUnit project,
- Install the following NuGet packages.
- Microsoft.Playwright
- Microsoft.Playwright.NUnit
- Microsoft.Playwright.TestAdapter
Please note that I am using VS 2022, but any version should work just as well.
Next, create three folders to organize the automation framework
- WebElements_recorded_codegen
Create a class under this folder, then paste the generated code by codegen (from Figure 5) into it. After pasting it, comment out the code, since we only need this code to copy and paste into the ChatGPT prompt.
- Tests_generated_chatgpt
Create a class under this folder, then paste the code generated by ChatGPT (Figure 7) into it. This is a complete test case. Compile it and run it. Congratulations!
- Pages_generated_chatgpt
Create a class under this folder, then paste the code generated by ChatGPT (Figure 7) into it. This is a complete test case. Compile it and run it. Congratulations!
Here is the structure of the solution after completing the steps above.

This marks the completion of our minimal framework with the assistance of ChatGPT. As we proceed with the development of automation frameworks in our daily work, we will consistently utilize ChatGPT to create new test cases, integrate additional page objects to accommodate these cases, and refactor the code as necessary.
7. Points of Interest
ChatGPT is a great tool to use, and it is here to stay. Here are some of my thoughts on using it for this project.
- The shorter and the more specific the prompts, the better results I got.
For example, in my initial prompts, I was curious to see what the response would be, so I created a prompt with multiple objects. Here is an example, where I provided the code snippet in Figure 5.
The following code snippet represents an incomplete case.
- Generate a completed test case
- Use namespace “PlaywrightAutomationDemo”
- Use class name “Page_Home”
- Do not inherit from “PageTest”
- For each page object that “Page_Home” makes use of, create a separate page object called “Page_<Name>, where <Name> is the name of the object. For example, “Page_Computers” would be one such example.
- Create each class in its own file. The file name should be the same as the class name.
- Use lambda expressions to refactor all hard-coded values as class properties
(Note: I provided the code snippet from Figure 5 here)
8. GitHub Repo
Here is the code for this initial release of the framework on GitHub.
9. Conclusion
Leveraging ChatGPT and the Playwright's Codegen tool has proved to be an effective approach for rapidly developing automated testing frameworks. By generating well-structured code snippets and page objects, we were able to streamline and accelerate the process of building an initial version of the framework.
Through the combination of manual test case analysis, automated code generation, and iterative refinement, ChatGPT served as a valuable assistant throughout the development process, providing helpful suggestions and code snippets.
Moving forward, we will continue to use ChatGPT to iterate on our framework, incorporating additional test cases, refining existing code, and addressing any maintenance challenges that arise. With ChatGPT's assistance, we can ensure that our testing framework remains flexible, scalable, and aligned with best practices in software testing.
Test Automation Architect with a focus on designing and implementing automation frameworks that are extensible and modular, with re-usable components, using the Page Object Model (POM). Areas of interest include Azure DevOps, C#, Visual Studio (2022), Blazor, MVC, API testing, Selenium, and Playwright.