Introduction
In this article, you will learn how to populate menu items dynamically from a database. The dynamicity of the Menu
control is particularly useful
when you need to restrict users from using some specific menu items.
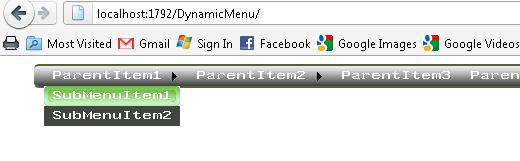
Creating the Database Tables
You will need a table in the database to store the MenuItem
s
.

Here, the MenuLocation column contains the URL of the target page when the corresponding menu item is clicked.
Sample data(For Reference):
Step 1: You need to specify some parent menu items with parentid = 0
Step 2: You can then assign submenuitems to these parents
For instance consider the following structure-
MenuID MenuName MenuLocation ParentID
1 ParentItem1 NULL 0
2 ParentItem2 NULL 0
3 ParentItem3 NULL 0
11 SubMenuItem1 NULL 1
12 SubMenuItem2 NULL 1
21 SubMenuItem3 NULL 2
Here ParentItem1,2,3 are Parents and SubMenuItem1,2,3 are their childs as specified.
As far as MenuLocation Column is conerned you can assign urls in it e.g Default.aspx
or User/NewUserAdd.aspx
etc. according to the directory structure of your application.
MasterPage
Step 1: Place the ASP.NET Menu
control in the MasterPage.
<div class="MenuBar">
<asp:Menu ID="menuBar" runat="server" Orientation="Horizontal" Width="100%">
<DynamicHoverStyle CssClass="DynamicHover" />
<DynamicMenuItemStyle CssClass="DynamicMenuItem" />
<DynamicSelectedStyle CssClass="DynamicHover" />
<StaticHoverStyle CssClass="staticHover" />
<StaticMenuItemStyle CssClass="StaticMenuItem" ItemSpacing="1px" />
<StaticSelectedStyle CssClass="staticHover" />
</asp:Menu>
</div>
MasterPage Code-Behind
Step 2: Using the following code, you are pulling the menu and submenu from the database in proper order. Call the following function from the Page_Load
event of the MasterPage when !IsPostBack
.
private void getMenu()
{
Connect();
con.Open();
DataSet ds = new DataSet();
DataTable dt = new DataTable();
string sql = "Select * from tbl_WebMenu";
SqlDataAdapter da = new SqlDataAdapter(sql, con);
da.Fill(ds);
dt = ds.Tables[0];
DataRow[] drowpar = dt.Select("ParentID=" + 0);
foreach (DataRow dr in drowpar)
{
menuBar.Items.Add(new MenuItem(dr["MenuName"].ToString(),
dr["MenuID"].ToString(), "",
dr["MenuLocation"].ToString()));
}
foreach (DataRow dr in dt.Select("ParentID >" + 0))
{
MenuItem mnu = new MenuItem(dr["MenuName"].ToString(),
dr["MenuID"].ToString(),
"", dr["MenuLocation"].ToString());
menuBar.FindItem(dr["ParentID"].ToString()).ChildItems.Add(mnu);
}
con.Close();
}
Points of Interest
This type of menu population is particularly useful when both the user count and menu item count are variable because you can set the access permissions (restricting users from
accessing some pages at runtime) which will be described in the next article.
Note
The menu control may not work as intended in some browsers such as chrome. So in that case you may have
to add a '.browser' file under 'App_Browsers' folder of your project.

Open file safari.browser from Solution Explorer and remove all the code clear and add the following:
<browsers>
<browser refID="safari1plus">
<controlAdapters>
<adapter controlType="System.Web.UI.WebControls.Menu" adapterType="" />
</controlAdapters>
</browser>
</browsers>
Save the safari.browser file and there you go..