Introduction
A while ago, I needed to implement a set of checkboxes that were organised in a hierarchical manner (in the form of a tree). i.e., the state of one checkbox affected its parent/child node checkbox.
The attached code is my way to simplify coding of such checkboxes.
Please see the comments in the code library file for details on all member functions and properties. The attachment contains the code library as well as some test files.
Let's see an example. Suppose we have to create checkboxes for the following tree:
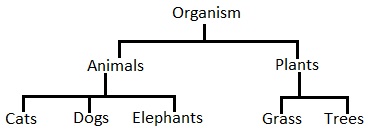
Let's start by creating the checkbox
es and collecting their references in JavaScript.
<!--
<label> <input type="checkbox" value="2310"> Organism </label>
<label> <input type="checkbox" value="30"> Animals </label>
<label> <input type="checkbox" value="2"> Cats </label>
<label> <input type="checkbox" value="3"> Dogs </label>
<label> <input type="checkbox" value="5"> Elephants </label>
<label> <input type="checkbox" value="77"> Plants </label>
<label> <input type="checkbox" value="7"> Grass </label>
<label> <input type="checkbox" value="11"> Trees </label>
<script>
var chkboxes = document.body.getElementByTagName("input");
</script>
Now, we can impose the hierarchical nature on these checkboxes using the library as follows:
<script>
(function() {
var chkbox_tree = CHK_TREE(chks[0], "my_tree", function(chkbox, tree) {
console.log("Checkbox clicked -->", chkbox);
console.log("All checked values -->", tree.getCheckedValue());
});
var animals = chkbox_tree.addCh(chkboxes[1]);
animals.addCh(chkboxes[2]);
animals.addCh(chkboxes[3]);
animals.addCh(chkboxes[4]);
var plants = chkbox_tree.addCh(chkboxes[5]);
plants.addCh(chkboxes[6]);
plants.addCh(chkboxes[7]);
chkbox_tree.log();
})();
</script>
Now if someone checks all of the {Cats
, Dogs
, Elephants
} checkboxes, then the Animals
checkbox will automatically be checked;
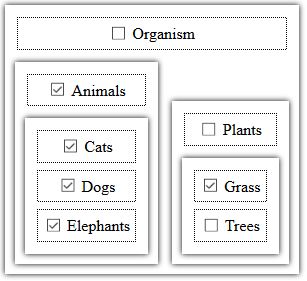
And if then you uncheck the Cats
checkbox, then the Animals
checkbox will automatically be unchecked. This works for all the parent/child relations in the tree.
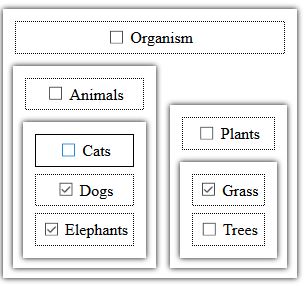
There is a single handler function for all the checkboxes which makes the handling of the result very easy.
I hope that you might find it useful too.
Languages that I work in: Python, JavaScript, C++, C, HTML, CSS, C#, PHP, SQL