Introduction
Sometimes working with django admin interface requires some tool that allows one place to collect and analyze vital statistics. For example, in our case, we would like to aggregate all available information about users and put it into a speadsheet, which could be convenient to work with. Since the extension of the standard functionality, Django is very labor-intensive, and ready-made solutions have not been found, we decided to make a separate page on JavaScript.
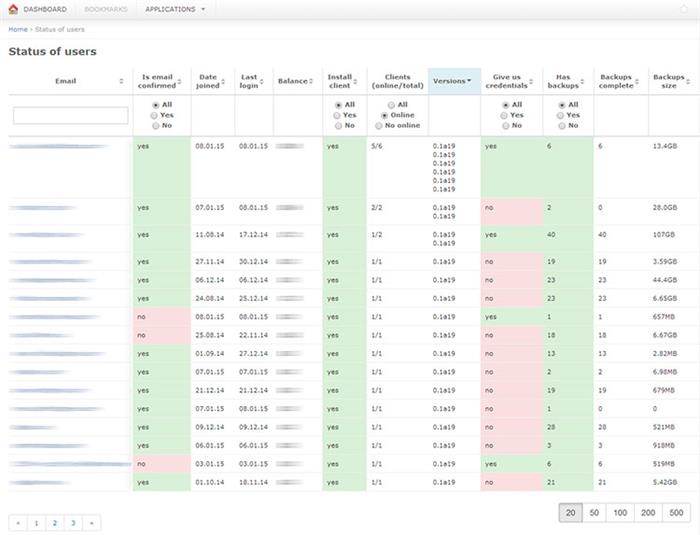
Create a New Page in the Django Admin Interface
To create a new page with reports by users, we need to use the ModelAdmin.get_urls()
method in the model UserAdmin
in admin.py:
def get_urls(self):
urls = super(UserAdmin, self).get_urls()
return patterns('', (r'report/,
admin.site.admin_view(self.report_view))) + urls
The report_view()
method in the same model collects a report on all users and places it in the context of a specific html-file (in this case 'admin/users_report.html'). Before it, all the data is converted to JSON.
def report_view(self, request):
data = (self.user_report(user) for user in User.objects.all().order_by('-date_joined'))
context = {'report': json.dumps(list(data))}
return HttpResponse(render(request,
'admin/users_report.html',
context),
content_type='text/html')
In the method user_report()
we create a regular dict for users, containing the necessary information to display.
Now, our report can be accessed at /admin/myapp/user/report/.
The Structure of the File with the Report
The report file is a standard html-file that has a JavaScript code in line:
{% extends 'admin/base_site.html' %}
{% block content %}
<style>
@import "{{ STATIC_URL }}admin/ng-table.min.css";
</style>
<div ng-app="Report">
...
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script>
<script src="{{ STATIC_URL }}admin/ng-table.min.js"></script>
<script type="text/javascript">
(function () {
});
</script>
{% endblock %}
To work with the spreadsheet, you just connect the two files and AngularJS ngTable
(more documentation).
Angular Application
The application itself in this case becomes quite small and consists of only one module. It describes the overall structure of the table and its logic.
A small offtop: it is common knowledge that in Angular and in Django, in order to access the data in the template, we use expressions such as {{ value }}
. We should somehow differentiate them to avoid conflicts. For this sake, we can replace the character sequences that would be used in Angular. For example, we can use {+ value +
}. This is done simply by interpolateProvider
:
.config(function($interpolateProvider) {
$interpolateProvider.startSymbol('{+');
$interpolateProvider.endSymbol('+}');
})
In report module, we connect ngTable
, and in the view we use ng-table
directive. The data previously placed in context is now available in the script. It can now be used via JSON string
parsing.
<table ng-table="tableParams"></table>
angular.module('Report', ['ngTable'])
.controller('ReportCtrl', [
'$scope', 'ngTableParams',
function ($scope, ngTableParams) {
var data = JSON.parse('{{ report|safe }}');
$scope.tableParams = new ngTableParams({
...
}, {
...
});
}])
That's it, our mini-application is ready for operation. In ngTableParams
, there are many ways to configure the table, thereby adding different functions. Full details of all the available features are accessible on the website ngTable and also GitHub. It really helps our project.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.