In this post, I will talk about one of the patterns which I usually use while writing unit tests. Apart from MOQ library, I also use Fluent Assertions. You can search for the same in nuget package manager and install it in the test project. Out of the box, fluent assertions give tons of extensions method which help to easily write assertions on the actual like shown below. In the below example, I am just running the test against one sample string of my name.
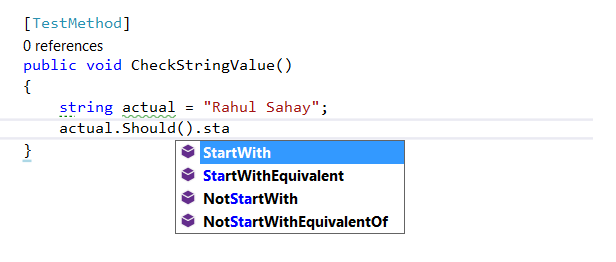
Now, when I complete this test, it will look like:
[TestMethod]
public void CheckStringValue()
{
string actual = "Rahul Sahay";
actual.Should().StartWith("Ra").And.EndWith("ay");
}
When I run the same, it will produce the below result.

Similarly, to verify that a collection contains a specified number of elements and that all elements match a predicate, you can test like shown below:
[TestMethod]
public void CheckCollection()
{
IEnumerable collection = new[] { 1, 2, 3,4,5 };
collection.Should().HaveCount(6, "because I thought I put 5 items in the collection");
}
Now, when I run the above test, it will fail. And this will fail for a valid reason. But, look at the error message returned by the same.

Let, me show the same against one of the infrastructure code where I am using the same.
[TestMethod]
public void MovieNameTest()
{
IEnumerable<Movie> movie;
var mockMovieRepository = MovieRepository(out movie);
mockMovieRepository.Setup(obj => obj.GetMovies()).Returns(movie);
IMovieService movieService = new MovieManager(mockMovieRepository.Object);
IEnumerable<MovieData> data = movieService.GetDirectorNames();
data.Should().HaveCount(4, "because we put these many values only");
}
Now, it has one dependency on MovieRepository(out movie)
. Basically, this is my arrange.
private static Mock<IMovieRepository> MovieRepository(out IEnumerable<Movie> movie)
{
Mock<IMovieRepository> mockMovieRepository = new Mock<IMovieRepository>();
movie = new Movie[]
{
new Movie()
{
MovieName = "Avatar",
DirectorName = "James Cameron",
ReleaseYear = "2009"
},
new Movie()
{
MovieName = "Titanic",
DirectorName = "James Cameron",
ReleaseYear = "1997"
}
};
return mockMovieRepository;
}
When I run the above test, it will again throw a similar error because count is 2
and I am checking for 4
.

Thanks for joining me. I hope you like it.
Happy coding!
Hey there, it's Rahul Sahay! I'm thrilled to be a platform specialist at Publicis Sapient, where I get to work on some exciting projects. I've been honing my skills in various aspects of the software development life cycle for more than 15 years, with a primary focus on web stack development. I've been fortunate to have contributed to numerous software development initiatives, ranging from client applications to web services and websites. Additionally, I enjoy crafting application architecture from scratch, and I've spent most of my time writing platform agnostic and cloud agnostic code. As a self-proclaimed code junkie, software development is more than just a job to me; it's a passion! And I consider myself lucky to have worked with an array of cutting-edge technologies, from .NetCore to SpringBoot 3, from Angular to React, and from Azure to AWS and many more cousin technologies...
- š Iām currently working @ below tech stacks
- Microservices,
- Distributed Systems,
- Spring Boot
- Spring Cloud
- System Design,
- Docker,
- Kubernetes,
- Message Queues,
- ELK Stack
- DotNetCore,
- Angular,
- Azure
- š¬ Ask me anything about my articles [My View](https://myview.rahulnivi.net/)
- š« How to reach me: [@rahulsahay19](https://twitter.com/rahulsahay19)
- š« Github: [@rahulsahay19](https://github.com/rahulsahay19)