In this article we can learn how to file upload in asp.net mvc using Dropzone JS and HTML . For this we will create a default asp.net mvc web application. Before jumping to the project, first we will download the dropzone js files.
File upload part 1: Limit Number of files upload using Dropzonejs Options – Part 1
File upload part 2: Removing thumbnails from dropzone js– Part 2
DropzoneJS is an open source library that provides drag’n’drop file uploads with image previews.
It’s lightweight, doesn’t depend on any other library (like jQuery) and is highly customizable.
You can download the latest version from the official site here http://www.dropzonejs.com/ and also we can install using the nuget package manage console by the following command Package Manager Console
PM> Install-Package dropzone
File upload Implementation
After creating the default mvc project, install dropzone js using the nuget command.
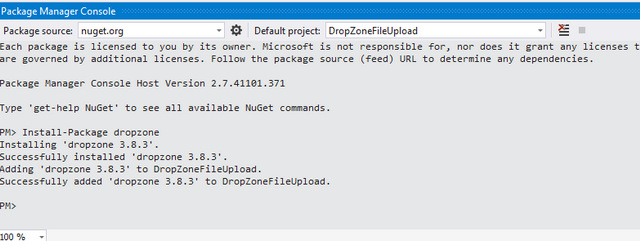
install dropzone using nuget package
Now create a bundle for your script file in BundleConfig.cs
bundles.Add(new ScriptBundle("~/bundles/dropzonescripts").Include(
"~/Scripts/dropzone/dropzone.js"));
Similarly add the dropzone stylesheet in the BundleConfig.cs
bundles.Add(new StyleBundle("~/Content/dropzonescss").Include(
"~/Scripts/dropzone/css/basic.css",
"~/Scripts/dropzone/css/dropzone.css"));
Now add the bundle reference in your _Layout page

dropzonelayout
Now every thing is fine now we can start writing up the application code. Now /Home/Index.cshtml file and Dropzone form in the page.
<div class="jumbotron">
<form action="~/Home/SaveUploadedFile" method="post" enctype="multipart/form-data" class="dropzone" id="dropzoneForm" style="width: 50px; background: none; border: none;">
<div class="fallback">
<input name="file" type="file" multiple />
<input type="submit" value="Upload" />
</div>
</form>
</div>
Now open the HomeController.cs and add the following code as follows
public ActionResult SaveUploadedFile()
{
bool isSavedSuccessfully = true;
string fName = "";
try{
foreach (string fileName in Request.Files)
{
HttpPostedFileBase file = Request.Files[fileName];
fName = file.FileName;
if (file != null && file.ContentLength > 0)
{
var originalDirectory = new DirectoryInfo(string.Format("{0}Images\\WallImages", Server.MapPath(@"\")));
string pathString = System.IO.Path.Combine(originalDirectory.ToString(), "imagepath");
var fileName1 = Path.GetFileName(file.FileName);
bool isExists = System.IO.Directory.Exists(pathString);
if (!isExists)
System.IO.Directory.CreateDirectory(pathString);
var path = string.Format("{0}\\{1}", pathString, file.FileName);
file.SaveAs(path);
}
}
}
catch(Exception ex)
{
isSavedSuccessfully = false;
}
if (isSavedSuccessfully)
{
return Json(new { Message = fName });
}
else
{
return Json(new { Message = "Error in saving file" });
}
}
Now add the following script to your Index.cshtml page
Dropzone.options.dropzoneForm = {
init: function () {
this.on("complete", function (data) {
var res = JSON.parse(data.xhr.responseText);
});
}
};
Also add the following css
#dropZone {
background: gray;
border: black dashed 3px;
width: 200px;
padding: 50px;
text-align: center;
color: white;
}
Source Code
You can download the source from the Github
The post File upload in ASP.NET MVC using Dropzone JS and HTML5 appeared first on Venkat Baggu Blog.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.