The source code for these templates can be found here: https://github.com/gomobile/iotapp-local-temperature or download the Intel® XDK IoT Edition to check out all the node.js IoT application templates and samples plus HTML5 companion apps.
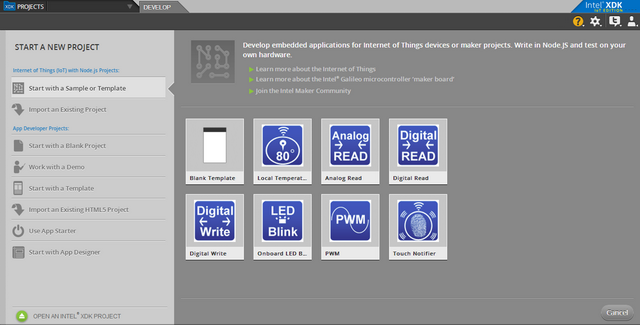
Introduction

Intel® XDK IoT Edition is a HTML5 hybrid and node.js application development environment that allow users to deploy, run, debug on various IoT platforms such as the Intel® Galileo and Edison board running the IoT Development Kit Linux Image and utilizes the Grover Starter Kit Plus – IoT Intel® Edition. With the starter kit and Linux* image installed, your development platform is ready to connect to Intel® XDK IoT Editon and run your node.js applications. Along with development features, this development environment provides various node.js templates and samples intended for running on Intel IoT platforms. For more information on getting started, go to https://software.intel.com/en-us/html5/documentation/getting-started-with-intel-xdk-iot-edition.
Purpose

The Local Temperature Node.js sample application distributed within Intel® XDK IoT Edition under the IoT with Node.js Projects project creation option showcases how to read analog data from a Grover Starter Kit Plus – IoT Intel® Edition Temperature Sensor, start a web server and communicate wirelessly using WebSockets. In order to communicate with sensors, this sample uses the MRAA Sensor Communication Library. The intent of this library is to make it easier for developers and sensor manufacturers to map their sensors & actuators on top of supported hardware and to allow control of low level communication protocol by high level languages & constructs.

The Local Temperature sample HTML5 companion application is also distributed under the Work with a Demo project creation option. This application communicates with node.js IoT application using WebSockets API and visualizes the received temperature data in a scatter plot using the D3 JavaScript Library.
Design Considerations
This sample requires the mraa library and xdk_daemon to be installed on your board. These two requirements are included in the IoT Development Kit Linux Image which enables communication between your board and Intel® XDK IoT Edition plus access to the IO pins. An active connection to your local network using an Ethernet cable or Wireless card such as the Intel 135N PCI Express WiFi/Bluetooth card is required for the WebSockets communication between the board and mobile device. The node.js application reads data from the temperature sensor then converts the value to degrees Fahrenheit. The collected temperature data is sent to the connected (mobile) clients using WebSockets.
[image of node.js application source & development board with sensors connected]
Node.js IoT application
Required npm packages:
These packages are listed in the package.json file to be built and installed on the connected development board. This is done by clicking the Install/Build button in the bottom toolbar. After installing the node modules, uploading (Click Upload button) and running (Click Run button) this project using Intel XDK IoT Edition will allow for evaluating the communication between the board and mobile application.
Note: You will need to wait for the upload process to complete before running your project.
Web Server
var app = require('express')();
var http = require('http').Server(app);
app.get('/', function (req, res) {
'use strict';
res.send('<h1>Hello world from Intel Galileo</h1>');
});
http.listen(1337, function () {
'use strict';
console.log('listening on *:1337');
});
Analog Read
var mraa = require("mraa");
var myAnalogPin = new mraa.Aio(0);
var a = myAnalogPin.read() >> 2;
WebSockets
var io = require('socket.io')(http);
io.on('connection', function (socket) {
'use strict';
console.log('a user connected');
socket.emit('connected', 'Welcome');
startSensorWatch(socket);
socket.on('disconnect', function () {
console.log('user disconnected');
});
});

The HTML5 mobile application requires for that the IP address of your board be inputted to connect to the necessary WebSockets connection for visualizing the temperature data on your mobile device which was collected by your development board.
HTML5 Companion application
WebSockets
try {
socket = io.connect("http://" + ip_addr + ":" + port);
socket.on("connected", function (message) {
navigator.notification.alert(
"Great Job!",
"",
'You are Connected!',
'Ok'
);
$.ui.showBackButton = false;
$.ui.loadContent("#main", false, false, "fade");
});
socket.on("message", function (message) {
chart_data.push(message);
plot();
$("#feedback_log").text("Last Updated at " + Date().substr(0, 21));
});
} catch (e) {
navigator.notification.alert(
"Server Not Available!",
"",
'Connection Error!',
'Ok'
);
}
D3.js Scatter plot
var chart_svg = d3.select("#chart").append("svg").attr("id", "container1").attr("width", window.innerWidth).attr("height", 0.6 * height).append("g");
chart_svg.attr("transform", "translate(25," + margin.top + ")");
var x1 = d3.scale.linear().domain([0, 5000]).range([0, 100000]);
var y1 = d3.scale.linear().domain([0, 200]).range([0.5 * height, 0]);
chart_svg.selectAll("line.y1")
.data(y1.ticks(10))
.enter().append("line")
.attr("class", "y")
.attr("x1", 0)
.attr("x2", 100000)
.attr("y1", y1)
.attr("y2", y1)
.style("stroke", "rgba(8, 16, 115, 0.2)");
chart_svg.append("text").attr("fill", "red").attr("text-anchor", "end").attr("x", 0.5 * window.innerWidth).attr("y", 0.55 * height).text("Periods");
var x1Axis = d3.svg.axis().scale(x1).orient("top").tickPadding(0).ticks(1000);
var y1Axis = d3.svg.axis().scale(y1).orient("left").tickPadding(0);
chart_svg.append("g").attr("class", "x axis").attr("transform", "translate(0," + y1.range()[0] + ")").call(x1Axis);
chart_svg.append("g").attr("class", "y axis").call(y1Axis);
var dottedline = d3.svg.line().x(function (d, i) {
'use strict';
return x1(i);
}).y(function (d, i) {
'use strict';
return y1(d);
});
......
Development /Testing
Each of the templates have been test on Intel® Galileo Generation 1 and 2 boards as well as the Intel® Edison board.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.