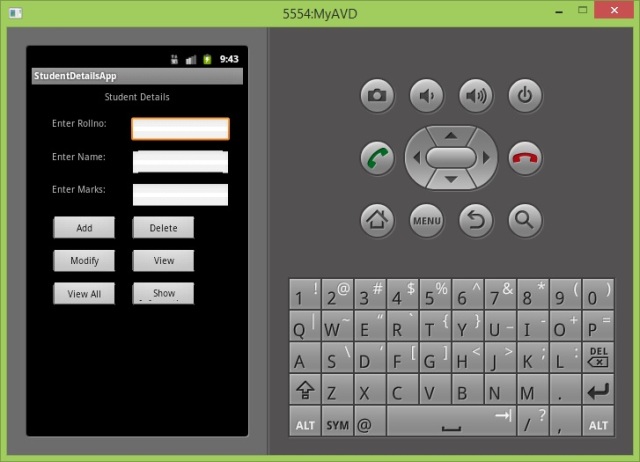

Introduction
In this article, I have attempted to demonstrate the use of SQLite database in Android in the simplest manner possible. Most of the articles and demos which I have seen on the net were not very simple for a layman to understand. Also most of the examples assume a deep knowledge of Android and SQL. This article assumes that the user has a working knowledge of Android and basic SQL commands. The example application shows how to perform basic DML and query operations on an SQLite table in Android.
Background
The example application which I have created is a simple Student Management System, which allows a user to add, delete, modify and view student details. The application accepts a student's roll number, name and marks and adds these details to a student
table. For simplicity, I have created all fields of VARCHAR
data type, which is a variable length character string
.
Using the Code
The SQLiteDatabase
class from the android.database.sqlite
package and the Cursor
class from the android.database
package provide all the functionality required for performing Data Manipulation Language (DML) and query operations on an SQLite table.
The following code shows how to create an SQLite database and a table in the database.
db=openOrCreateDatabase("StudentDB", Context.MODE_PRIVATE, null);
db.execSQL("CREATE TABLE IF NOT EXISTS student(rollno VARCHAR,name VARCHAR,marks VARCHAR);");
In the above code, the openOrCreateDatabase()
function is used to open the StudentDB
database if it exists or create a new one if it does not exist. The first parameter of this function specifies the name of the database to be opened or created. The second parameter, Context.MODE_PRIVATE
indicates that the database file can only be accessed by the calling application or all applications sharing the same user ID. The third parameter is a Cursor factory object which can be left null
if not required.
The db.execSQL()
function executes any SQL command. Here it is used to create the student
table if it does not already exist in the database.
Following is the full code of the onCreate()
method of the main activity.
public void onCreate(Bundle savedInstanceState)
{
<code>super.onCreate(savedInstanceState);
setContentView(R.layout.main);
editRollno=(EditText)findViewById(R.id.editRollno);
editName=(EditText)findViewById(R.id.editName);
editMarks=(EditText)findViewById(R.id.editMarks);
btnAdd=(Button)findViewById(R.id.btnAdd);
btnDelete=(Button)findViewById(R.id.btnDelete);
btnModify=(Button)findViewById(R.id.btnModify);
btnView=(Button)findViewById(R.id.btnView);
btnViewAll=(Button)findViewById(R.id.btnViewAll);
btnShowInfo=(Button)findViewById(R.id.btnShowInfo);
btnAdd.setOnClickListener(this);
btnDelete.setOnClickListener(this);
btnModify.setOnClickListener(this);
btnView.setOnClickListener(this);
btnViewAll.setOnClickListener(this);
btnShowInfo.setOnClickListener(this);
db=openOrCreateDatabase("StudentDB", Context.MODE_PRIVATE, null);
db.execSQL("CREATE TABLE IF NOT EXISTS student(rollno VARCHAR,name VARCHAR,marks VARCHAR);");
}
In the onClick()
event handler, we can write the code required to add, delete, modify and view records.
The following code uses the db.execSQL()
function to insert a student
record in the student
table.
db.execSQL("INSERT INTO student VALUES('"+editRollno.getText()+"','"+
editName.getText()+"','"+editMarks.getText()+"');");
The above code generates an INSERT
statement by appending the contents of the editable fields into a string
and executes the INSERT
statement.
In the same way, the DELETE
command can be executed as follows:
db.execSQL("DELETE FROM student WHERE rollno='"+editRollno.getText()+"'");
The above code deletes the record of the student whose roll number is entered in the editable field.
The UPDATE
command can be executed as follows:
db.execSQL("UPDATE student SET name='"+editName.getText()+"',marks='"+
editMarks.getText()+"' WHERE rollno='"+editRollno.getText()+"'");
The above code updates the record of the student whose roll number is entered in the editable field.
To view a student record, we execute a query using the rawQuery()
method of the SQLiteDatabase
class as follows:
Cursor c=db.rawQuery("SELECT * FROM student WHERE rollno='"+editRollno.getText()+"'", null);
if(c.moveToFirst())
{
editName.setText(c.getString(1));
editMarks.setText(c.getString(2));
}
The above code uses the rawQuery()
method of the SQLiteDatabase
class to execute the SELECT
statement to select the record of the student, whose roll number is specified. It then checks if the record is found using the moveToFirst()
method of the Cursor
class and displays the name and marks in the respective editable fields.
To view all records, the following code can be used:
Cursor c=db.rawQuery("SELECT * FROM student", null);
if(c.getCount()==0)
{
showMessage("Error", "No records found");
return;
}
StringBuffer buffer=new StringBuffer();
while(c.moveToNext())
{
buffer.append("Rollno: "+c.getString(0)+"\n");
buffer.append("Name: "+c.getString(1)+"\n");
buffer.append("Marks: "+c.getString(2)+"\n\n");
}
showMessage("Student Details", buffer.toString());
The above code executes the SELECT
command to retrieve records of all students and appends them into a string
buffer. Finally, it displays the student details using the user-defined showMessage()
function.
Following is the full code of the onClick()
event handler:
public void onClick(View view)
{
if(view==btnAdd)
{
if(editRollno.getText().toString().trim().length()==0||
editName.getText().toString().trim().length()==0||
editMarks.getText().toString().trim().length()==0)
{
showMessage("Error", "Please enter all values");
return;
}
db.execSQL("INSERT INTO student VALUES('"+editRollno.getText()+"','"+editName.getText()+
"','"+editMarks.getText()+"');");
showMessage("Success", "Record added");
clearText();
}
if(view==btnDelete)
{
if(editRollno.getText().toString().trim().length()==0)
{
showMessage("Error", "Please enter Rollno");
return;
}
Cursor c=db.rawQuery("SELECT * FROM student WHERE rollno='"+editRollno.getText()+"'", null);
if(c.moveToFirst())
{
db.execSQL("DELETE FROM student WHERE rollno='"+editRollno.getText()+"'");
showMessage("Success", "Record Deleted");
}
else
{
showMessage("Error", "Invalid Rollno");
}
clearText();
}
if(view==btnModify)
{
if(editRollno.getText().toString().trim().length()==0)
{
showMessage("Error", "Please enter Rollno");
return;
}
Cursor c=db.rawQuery("SELECT * FROM student WHERE rollno='"+editRollno.getText()+"'", null);
if(c.moveToFirst())
{
db.execSQL("UPDATE student SET name='"+editName.getText()+"',marks='"+editMarks.getText()+
"' WHERE rollno='"+editRollno.getText()+"'");
showMessage("Success", "Record Modified");
}
else
{
showMessage("Error", "Invalid Rollno");
}
clearText();
}
if(view==btnView)
{
if(editRollno.getText().toString().trim().length()==0)
{
showMessage("Error", "Please enter Rollno");
return;
}
Cursor c=db.rawQuery("SELECT * FROM student WHERE rollno='"+editRollno.getText()+"'", null);
if(c.moveToFirst())
{
editName.setText(c.getString(1));
editMarks.setText(c.getString(2));
}
else
{
showMessage("Error", "Invalid Rollno");
clearText();
}
}
if(view==btnViewAll)
{
Cursor c=db.rawQuery("SELECT * FROM student", null);
if(c.getCount()==0)
{
showMessage("Error", "No records found");
return;
}
StringBuffer buffer=new StringBuffer();
while(c.moveToNext())
{
buffer.append("Rollno: "+c.getString(0)+"\n");
buffer.append("Name: "+c.getString(1)+"\n");
buffer.append("Marks: "+c.getString(2)+"\n\n");
}
showMessage("Student Details", buffer.toString());
}
if(view==btnShowInfo)
{
showMessage("Student Management Application", "Developed By Azim");
}
}
The following user-defined function is used to display message to the user:
public void showMessage(String title,String message)
{
Builder builder=new Builder(this);
builder.setCancelable(true);
builder.setTitle(title);
builder.setMessage(message);
builder.show();
}
The following user-defined function is used to clear edit fields:
public void clearText()
{
editRollno.setText("");
editName.setText("");
editMarks.setText("");
editRollno.requestFocus();
}
Points of Interest
I hope that this article will be helpful to people new to the Android platform to understand developing database applications for Android before starting to write more complex applications.