In this article, you will find the internals, contents and code samples of using DataFrame Library for PPL.
Introduction
PPL is the Parenthesis Programming Language, in which all elements (statements, parameters, blocks) are enclosed in parentheses. PPL contains a preprocessor to simplify the writing programs and reduce the number of parentheses. PPL software includes console interpreter, WPPF graphic interpreter, utilities for creation code C# of user library, graphic Assistant, libraries for access to existing C# capabilities, tutorial and samples. PPL software can be connected to any user applications.
DataFrame
library is part of PPL software and includes different data processing methods for tables with named columns.
Software, similar DataFrame
library, are used in other languages, for example Pandas for Python.
Contents of DataFrame Library
DataFrame
library includes the following methods:
Create
SetWidthForAll
SetRow
SetColumn
Write
ClearColumns
Save
Read
InsertRows
AddRows
InsertColumns
AddColumns
RemoveRows
RemoveColumns
Sort
Reverse
SelectRows
UnSelectRows
Short help it is possible to get in interpreters CPPL.exe or WPPL.exe by command DataFrame.help(method_name)
or in Assistant.exe. For detailed explanations and samples, see in TutorialPPL.
Creation DataFrame Code
PPL Utility CreateULC.exe generates C# skeleton-code of Dataframe
project for subsequent filling. Library method names are defined in file config.cfg:
name = DataFrame
path =
methods = Create, SetRow, SetColumn, Write,
Save, Read,
InsertRows, AddRows,
InsertColumns, AddColumns,
RemoveRows, RemoveColumns,
ClearColumns, SetWidthForAll,
Sort, Reverse,
SelectRows, UnSelectRows
Generated code DataFrame.cs:
using System;
using System.Collections.Generic;
namespace PPLNS
{
public class DataFrame : AbstractClass
{
public DataFrame(PPL ppl)
{
this.ppl = ppl;
}
public void AddToKeywordDictionary()
{
keyword_dict = new Dictionary<string, PPL.OperatorDelegate>();
keyword_dict.Add("help", FuncHelp);
keyword_dict.Add("Create",FuncCreate);
keyword_dict.Add("SetRow",FuncSetRow);
keyword_dict.Add("SetColumn",FuncSetColumn);
keyword_dict.Add("Write",FuncWrite);
keyword_dict.Add("Save",FuncSave);
keyword_dict.Add("Read",FuncRead);
keyword_dict.Add("InsertRows",FuncInsertRows);
keyword_dict.Add("AddRows",FuncAddRows);
keyword_dict.Add("InsertColumns",FuncInsertColumns);
keyword_dict.Add("AddColumns",FuncAddColumns);
keyword_dict.Add("RemoveRows",FuncRemoveRows);
keyword_dict.Add("RemoveColumns",FuncRemoveColumns);
keyword_dict.Add("ClearColumns",FuncClearColumns);
keyword_dict.Add("SetWidthForAll",FuncSetWidthForAll);
keyword_dict.Add("Sort",FuncSort);
keyword_dict.Add("Reverse",FuncReverse);
keyword_dict.Add("SelectRows",FuncSelectRows);
keyword_dict.Add("UnSelectRows",FuncUnSelectRows);
help_dict.Add("help","DataFrame.help([name])");
help_dict.Add("Create","");
help_dict.Add("SetRow","");
help_dict.Add("SetColumn","");
help_dict.Add("Write","");
help_dict.Add("Save","");
help_dict.Add("Read","");
help_dict.Add("InsertRows","");
help_dict.Add("AddRows","");
help_dict.Add("InsertColumns","");
help_dict.Add("AddColumns","");
help_dict.Add("RemoveRows","");
help_dict.Add("RemoveColumns","");
help_dict.Add("ClearColumns","");
help_dict.Add("SetWidthForAll","");
help_dict.Add("Sort","");
help_dict.Add("Reverse","");
help_dict.Add("SelectRows","");
help_dict.Add("UnSelectRows","");
try
{
if (ppl.ImportList.ContainsKey("DataFrame") == false)
{
foreach (KeyValuePair<string, PPL.OperatorDelegate> pair in keyword_dict)
{
ppl.processing.keyword_dict.Add("DataFrame." + pair.Key, pair.Value);
}
ppl.ImportList.Add("DataFrame", this);
}
}
catch (Exception io)
{ }
}
public bool FuncCreate(List<string> parameters, ref string result,
Composite node = null)
{
try
{
}
catch (Exception ex)
{
ppl.print("Error: ...");
return false;
}
return true;
}
public bool FuncSetRow(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncSetColumn(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncWrite(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncSave(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncRead(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncInsertRows(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncAddRows(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncInsertColumns(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncAddColumns(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncRemoveRows(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncRemoveColumns(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncClearColumns(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncSetWidthForAll(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncSort(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncReverse(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncSelectRows(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
public bool FuncUnSelectRows(List<string> parameters, ref string result,
Composite node = null)
{
return true;
}
}
}
Body of each of these methods uses PPL API, code is not so difficult for understanding, but long enough for presentation on this page, and you may find it in DataFrame source.
Additionally, utility Create ULC.exe creates file DataFrame.json for utility Assistent.exe and you can see part of this file here:
[
{
"cmd": "help",
"ppl": ["DataFrame.help([name])"]
},
{
"cmd": "Create",
"ppl": ["DataFrame.Create([df_name])[(number rows)(column1)(column2)(column3)…]"]
},
{
"cmd": "SetRow",
"ppl": ["DataFrame.SetRow(df_name)(row index)(value column1)( value column2)…"]
},
.............................
]
Samples of Code
Sample 1
Creation First DataFrame
By default:
DataFrame
name is DF
,
number of rows is 10
,
column names - AB
,C
,...X
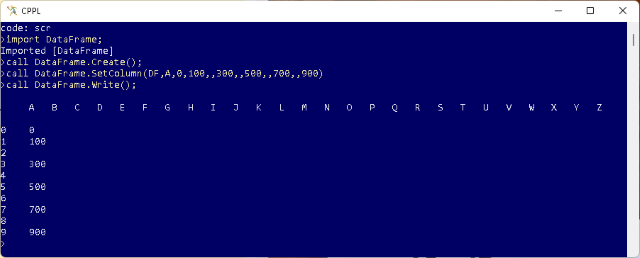
There are several ways to fill DataFrame
cells:
call DataFrame.SetColumn(dataframe name, column name, value row1, value row2,...)
call DataFrame.SetRow(dataframe name, row index, value column1, value column2,...)
- directly using operators PPL:
set dataframe_name.column_name[row index] = value
for example:
for above-mentioned sample set DF.B[0] = PPL
- read data from file in format csv or data(internal PPL format)
call DataFrame.Read(dataframe_name,filename)
Sample 2
File: examples\df\products.csv
Bagel,140,310,Medium
Buiscuit ,86,480,High
Jaffa cake,48,370,Med-High
Bread white,96,240,Medium
Bread wholemeal,88,220,LowMed
Chapatis,250,240,Medium
Cornflakes,130,300,Med-High
The folllowing PPL program creates DataFrame
, reads data from file csv, displays dataframe
, sorts by column per100gr ascending and displays it again:
Program: examples\df\df8.scr
import DataFrame;
DataFrame.Create(Products)(0)(Bread&Cereals)(Size)(per100grams)(energy);
set Products.Settings.Bread&CerealsWidth = 20;
DataFrame.Read(Products)(examples\df\products.csv);
DataFrame.Write(Products);
DataFrame.Sort(Products)(ascend)(per100grams);
DataFrame.Write(Products);

Conclusion
DataFrame
library contents will be expanded and any user suggestions to add will be accepted. As well, I am ready to help in creation of new PPL user libraries.
History
- 4th June, 2022: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.