Here we'll load pre-trained CNN to memory, and run age estimation.
In this series of articles, we’ll show you how to use a Deep Neural Network (DNN) to estimate a person’s age from an image.
In the previous article, we trained the CNN for age estimation. We reached an accuracy of 56% on the testing dataset. This means that our model can correctly predict the age group of a person in more than half of the cases. In this article – the last of the series – we will explain how to use the pre-trained CNN for estimating a person’s age from an image.
Load Pre-Trained CNN to Memory
To load the pre-trained CNN from the disk to memory, you use the following Python code:
from keras.models import load_model
netname = r"C:\Faces\age_class_net_16_256.cnn"
trained_net = load_model(netname)
Now our CNN is instantiated and ready to be used for age estimation. We need to get the image, preprocess it in the same way as we did for training, and feed the data to the model. The following Python class implements the estimation algorithm:
import numpy as np
from keras.preprocessing.image import img_to_array
class AgeEstimator:
def __init__(self, converters, net):
self.converters = converters
self.net = net
def estimate(self, image):
for (i, converter) in enumerate(self.converters):
image = converter.convert(image)
array = img_to_array(image, data_format="channels_last")
arrays = []
arrays.append(array)
data = np.array(arrays).astype("float")/255.0
prob = self.net.predict(data)
classes = prob.argmax(axis=1)
class_num = classes[0]
return class_num
The class constructor receives two parameters:
converters
– the list of converter instances for image preprocessing (the same converters we used for network training) net
– the pre-trained CNN
The estimate
method has a single parameter that refers to the image data. This method applies all conversions to the image data, converts the image to the special array format, and scales the values to the unit range [0.0, 1.0].
After conversion, we call the predict
method. Note that it receives an array of data. And what it returns is also an array, where each element is the array of the probabilities. We then use the argmax
function to get the age group that has the highest probability.
Run Age Estimation
Now we can create an instance of the class, get an image, and estimate the age of the person:
import cv2
import os
rc = ResizeConverter(128, 128)
gc = GrayConverter()
estimator = AgeEstimator([rc, gc], trained_net)
dataFolder = r"C:\Faces\Testing"
imageName = r"25_0_1_20170116002835308.jpg.chip.jpg"
imageFile = os.path.join(dataFolder, imageName)
image = cv2.imread(imageFile)
ageGroup = estimator.estimate(image)
Here we use the OpenCV cv2.imread
method to get the image from a file. Then we call the estimate
method and get the predicted age group number, ageGroup
. Knowing the age group number and the age group ranges, we can assign the group’s range to the person on the image.
The following code allows us to label the image with the age group and save it to a file:
ageranges = [1, 6, 11, 16, 19, 22, 31, 45, 61, 81, 101]
resultFolder = r"C:\Faces\Results"
cv2.putText(image, "["+str(ageranges[ageGroup])+"-"+str(ageranges[ageGroup+1]-1)+"]",
(4, 20), cv2.FONT_HERSHEY_SIMPLEX, 0.75, (255, 255, 255), 2, cv2.LINE_AA)
resultFile = os.path.join(resultFolder, imageName)
cv2.imwrite(resultFile, image)
We use the cv2.putText
method to draw the text on the image, then the cv2.imwrite
to save the annotated image to a file.
Here are some examples of the age-group-annotated images:
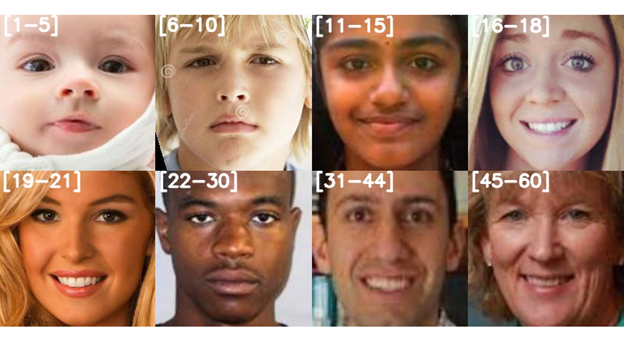
Note that you can get an image for the estimation function from the various sources: files, IP cameras, global or local networks, mobile devices, and so on. The estimated age can be then used for evaluating the demographics of retail customers or any other statistics.
Wrapping Up
In this article series, we discussed all the steps required to use modern DL algorithms for age estimation: selection of the training/testing dataset, design and implementation of the CNN, model training, and the actual estimation by the pre-trained CNN model. We hope this was useful. Good luck in your own DL exploits!