These posts focus on controlling intelligent precision electric motors from Windows applications written in C#. Specifically, we will be controlling a Schneider Electric M-Drive stepper motor with an integrated encoder and controller.
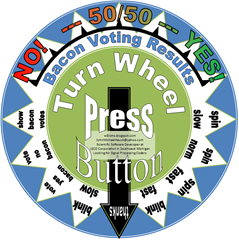
Posts in This Series
Concept
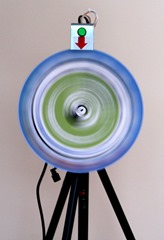
To demonstrate many of the programming concepts of the MDrive motor, I put together a tripod-mounted “Bacon Wheel”. The Bacon Wheel consists of a 10" inch paper disk, an Mdrive 17 motor, a power supply, a lighted button, some sheet metal, wires, and a camera tripod. Note the absence of a computer. The Bacon Wheel will be set in a hallway, kiosk style, at CodeMash 2013 (a conference for weird software developers).
After reading the instructions, the walk-up visitor should be able to manually rotate the paper disk to select one of several actions and then press the green flashing button. Yeah, this is kind of like the Fisher Price See ‘N Say:

The motor should then take some appropriate action, and then reset itself for the next iteration. The most unique feature allows the visitor to vote for (or against) the consumption of bacon by humans. The Bacon Wheel will indicate the current vote tally ratio by rotating the wheel to a spot along the No-Yes arc.
From just the above description, it should be evident that the MDrive has these capabilities:
- Ability to drive digital output signals (for controlling the light on the button)
- Ability to detect the rotational position of the motor shaft using an encoder
- Ability to read digital input signals (for detecting a button press)
- Ability to rotate the motor shaft at various speeds to various locations
- Ability to record events (votes) and perform mathematics on the results
- Ability to implement complex and arbitrary logic
The Hardware
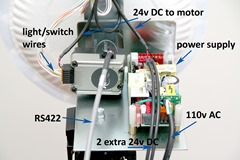
The two major electronic components shown are the MDrive motor and the uncovered 110VAC to 24VDC power supply. Two of the wires between the MDrive and the green button connect the LED, and two other wires lead to the contact closure of the switch. The fifth wire shown connected to the MDrive is for analog input and is not used. The power supply is connected to three sets of wires, one set runs to the MDrive motor and the other two are unused in this configuration. The RS422 cable is used for programming the MDrive logic from the computer, but is disconnected when the Bacon Wheel is being demonstrated in kiosk mode. Industrial grade double-stick foam tape is used to connect the motor shaft to the paper wheel.
The Kiosk Experience
If the visitor rotates the disk to:
- Spin Slow – The disk will rotate at 0.3 rpm through 22.5 degrees counter clockwise, and then return to the start position.
- Spin Norm – The disk will accelerate and rotate at about 60 rpm through two revolutions and then return to the start position.
- Spin Fast – The disk will accelerate and rotate at an alarming 300 rpm through 20 revolutions and then return to the start position.
- Blink Fast – The disk will return to the start position while the LED flashes at 50 Hz.
- Thanks – The disk will osculate +/- 22.5 degrees a few times and then return to the start position.
- Blink Slow – The disk will return to the start position while the LED flashes at 0.5 Hz.
- Vote Yes Bacon – A yes vote will be recorded. The disk will rotate to the thanks position and then will return to the start position.
- Vote No Bacon – A no vote will be recorded. The disk will rotate to the thanks position and then will return to the start position.
- Show Bacon Votes – The disk will rotate between the No and Yes positions, corresponding to the number of No and Yes votes recorded so far.
The Program
The code below is pretty much self-documenting. Refer to the invaluable MCode document to better understand each instruction.
LB SU ' Label. Label "SU" will begin executing on power-up
' Call subroutines
CL KS ' General setup
CL KH ' Home the motor
' Main loop
LB G0 ' Label. Branch here to keep looping
H 10 ' Hold. Wait 10ms
CL KL ' Toggle the light (toggle O2) at the rate in Z4
BR G0, I4 = 0 ' Cond branch. While switch (I4) not pushed
' Prepare for selected action
O2 = 0 ' Drive Digital Output. Turn light (O2) off (0)
Z4 = 10 ' set normal blink rate
' Call subroutines
CL KV ' Set normal velocities
CL KZ ' Calc Z1 as index (0-15) based on current position
' Call selected subroutine based on value of Z1
CL K0, Z1 = 4 ' show bacon votes
CL K1, Z1 = 5 ' vote no bacon
CL K2, Z1 = 6 ' vote yes bacon
CL K3, Z1 = 7 ' blink slow
CL K4, Z1 = 8 ' thanks
CL K5, Z1 = 9 ' blink fast
CL K6, Z1 = 10 ' spin fast
CL K7, Z1 = 11 ' spin norm
CL K8, Z1 = 12 ' spin slow
BR G0 ' Unconditional branch. Return to main loop
' Show bacon votes
LB K0
R3 = 768 * R1 ' Compute R3 as vote % pos (-384 to +384)
R4 = R1 + R2
R3 = R3 / R4
R3 = R3 - 384
MA 0 ' Move Absolute, home position
H ' Hold until movement is done
H 500 ' Hold half a second
MA R3 ' Move to vote results
RT
' Vote no bacon
LB K1 ' Label
R1 = R1 + 1 ' Increment R1
MA 1024 ' Move Absolute to "thanks"
H ' Hold until movement is done
H 1000 ' Hold one second
MA 0 ' Move to home
RT ' Return
' Vote yes bacon
LB K2
R2 = R2 + 1 ' Increment R2
MA 1024 ' Move Absolute to "thanks"
H ' Hold until movement is done
H 1000 ' Hold one second
MA 0 ' Move to home
RT ' Return
' Blink slow
LB K3
Z4 = 100
Z3 = 100
MA 0 ' Move to home
RT ' Return
' Thanks
LB K4
MR -128
H ' Hold until movement is done
MR 256
H
MR -256
H
MR 256
H
MA 0 ' Move to home
RT ' Return
' Blink fast
LB K5
Z4 = 1
MA 0 ' Move to home
RT ' Return
' Spin fast
LB K6
RC = 100
A = 20000
D = 20000
VM = 40000
MR 40960
H ' Hold until movement is done
MR -40960
H
CL KV
MA 0 ' Move to home
RT ' Return
' Spin norm
LB K7
MR 4096
H ' Hold until movement is done
MR -4096
H
MA 0 ' Move to home
RT ' Return
' Spin slow
LB K8
VM = 10
MR -128
H ' Hold until movement is done
CL KV
MA 0 ' Move to home
RT ' Return
' Set normal velocities
LB KV
RC = 25
A = 1000
D = 1000
VI = 10
VM = 10000
RT ' Return
' Print debug variables
LB KD
PR "P=", P, "$"
PR "R1=", R1, "$"
PR "R2=", R2, "$"
PR "R3=", R3, "$"
PR "R4=", R4, "$"
PR "Z1=", Z1, "$"
PR "Z2=", Z2, "$"
PR "Z3=", Z3, "$"
PR "Z4=", Z4, "$"
RT ' Return
' Blink light
LB KL
Z3 = Z3 + 1
BR GL, Z3 < Z4
Z2 = Z2 ^ 1
O2 = Z2
Z3 = 0
LB GL
RT
' General setup
LB KS
EE = 1 ' Enable the encoder (2048 per rev)
ER = 0 ' Clear errors
ST = 0 ' Clear stall
SF = 0 ' Stall factor. 0 means 0 tolerance
SM = 1 ' Stall mode. 0 means keep moving
HC = 0 ' Hold current %
RC = 25 ' Run current %
S1 = 16, 1, 0 ' Setup pin 1. Output. Lamp(-)
S2 = 16, 1, 1 ' Setup pin 2. Output. Lamp(+)
S3 = 16, 1, 0 ' Setup pin 3. Output. Switch COM
S4 = 0, 0, 0 ' Setup pin 4. Input. Switch NO
O1 = 0 ' Drive output pin
O2 = 0 ' Drive output pin
O3 = 0 ' Drive output pin
' Variable definitions
VA Z1 ' The wheel selection index (0-15)
VA Z2 ' A blink variable used to toggle the output line O2
VA Z3 ' A blink duration counter (0-Z4) (units of ms*10)
VA Z4 ' The blink duration (units of ms*10)
Z1 = 0
Z2 = 0
Z3 = 0
Z4 = 10
RT
' Homing
LB KH
A = 1000
D = 100000 ' Stop fast
VI = 100 ' Return home speed is 1/10th
VM = 1000 ' Move moderate speed
SL 0 ' Stop
H ' Hold until movement is done
H 100 ' Wait a touch
ER = 0 ' Clear errors
HI 1 ' Do the home procedure
H ' Wait until movement is done
MR -40 ' Adjustment factor for disk mount
H ' Wait until movement is done
P = 0 ' Declare this as position 0
RT
' Compute Z1 from current position from 0 to 15
LB KZ
Z1 = P + 64 ' Since we divide by 128, this is for rounding
LB G2
BR G3, Z1 >= 0 ' Make sure we are >= 0
Z1 = Z1 + 2048 ' By adding a full revolution
BR G2
LB G3
BR G4, Z1 < 2048 ' Make sure we are < 2048
Z1 = Z1 - 2048 ' By subtracting a full revolution
BR G3
LB G4
Z1 = Z1 / 128 ' The great divide
RT
E
John Hauck has been developing software professionally since 1981, and focused on Windows-based development since 1988. For the past 17 years John has been working at LECO, a scientific laboratory instrument company, where he manages software development. John also served as the manager of software development at Zenith Data Systems, as the Vice President of software development at TechSmith, as the lead medical records developer at Instrument Makar, as the MSU student who developed the time and attendance system for Dart container, and as the high school kid who wrote the manufacturing control system at Wohlert. John loves the Lord, his wife, their three kids, and sailing on Lake Michigan.