Introduction
CKEditor
is a very popular text editor for most developers. It provides many ways to configure and customize to get a best fit editor for your application. What we often do is choose a best version of CKEditor
, then choose what feature to use by modifying its config.js file or use Online Toolbar Configurator. But don't you know you can do more with CKEditor
by adding your own button and handle your specific business. This article will show you how.
Background
Before we start, the following prerequisite knowledge is required for you:
- Basic knowledge about web development
- You are expected to know what the
CKEditor
is, how to download and embed it to your website - Basic command with
CKEditor
like: how to make the editor visible, how to modify config file
If you are not familiar with the editor, try reading the documentation first.
Example Project
This project is a demo version in which you can learn how to customize CKE to serve your business demand. It's not a professional real-life project so there is no data validation, we assume that every input would be in a correct format.
Imagine you are working for a daily news company and are responsible for posting daily weather forecast. Your partner company sends to you the data about weather information in your country. Their temperature data uses Fahrenheit, but in your country Celsius is more popular. Everytime you receive the data, you need to calculate and convert °F to °C using the formula: T(°C) = (T(°F) - 32) × 5/9 . The idea is creating a customized button on your editor, and easily you can highlight some text like "93°F" and click on the button to convert to °C. It's faster and more convenience than using copy and paste to another tool.
Getting Things Ready
You are expected to get the editor ready to work.
<textarea id="editor"></textarea>
<script src="~/Scripts/ckeditor/ckeditor.js"></script>
<script>
var editor = CKEDITOR.replace('editor');
</script>
Adding a Button
To add a new custom button to CKEditor
, use this code:
editor.ui.addButton('ConvertFtoC',
{
label: 'Convert Fahrenheit to Celsius',
command: 'cmd_convert_F_to_C',
toolbar: 'others',
icon: '/Content/images/convert.svg'
}
);
Command
can be declared before or after we add a button.
Toolbar
is the name of the toolbar group in which the button is inserted. You can check some toolbar names at this link.
Adding a Command
To add a new command to process when the button is clicked, use this code:
editor.addCommand("cmd_convert_F_to_C", {
exec: function (edt) {
}
});
The first parameter of addCommand
method is the command name. It must be the same as the command name we assign to the new button.
Now, when you click the button name ConvertFtoC
, the command cmd_convert_F_to_C
would be executed.
If your code works, you should see the new button appear, otherwise check your code and fix error.
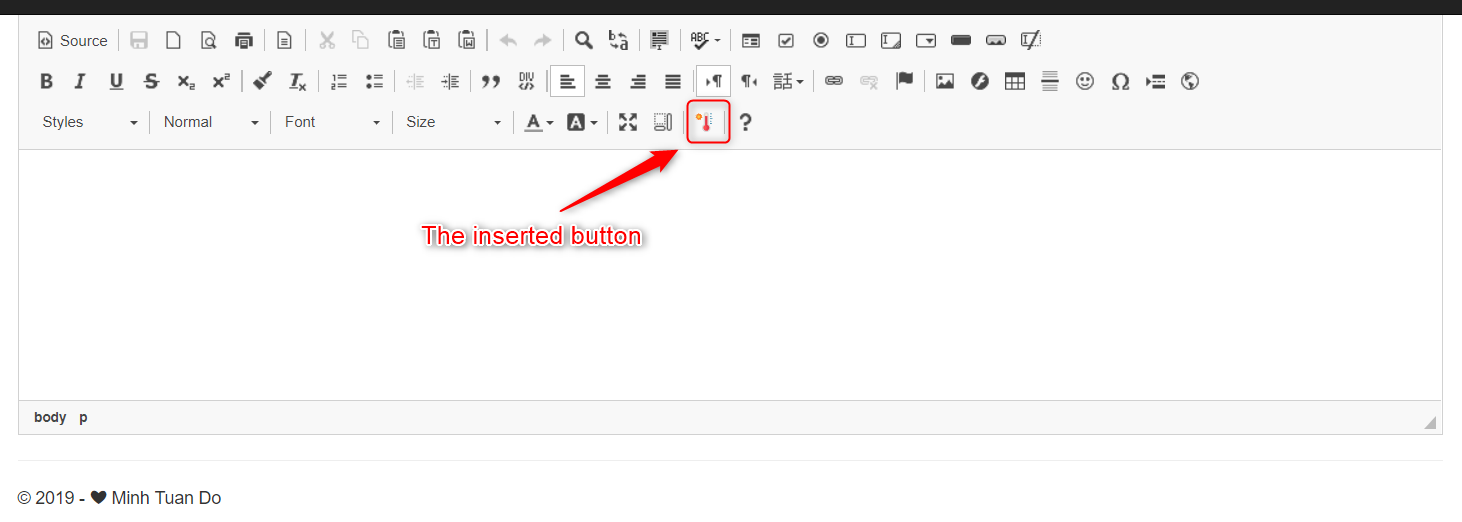
Get Highlighted Text
To get the highlighted text, use this code:
var mySelection = editor.getSelection();
var selectedText;
if (mySelection.getType() == CKEDITOR.SELECTION_TEXT) {
if (CKEDITOR.env.ie) {
mySelection.unlock(true);
selectedText = mySelection.getNative().createRange().text;
} else {
selectedText = mySelection.getNative();
}
}
var plainSelectedText = selectedText.toString();
Please notice that...
selectedText = mySelection.getNative();
...returns a HTML DOM element. It means we can treat it like an element and modify it normally such as adding an attribute or change its CSS style properties. To get the plain text of the selection, use the ToString
method.
Replace Highlighted Text
To replace the highlighted text by another text, simply insert the new text to our editor. If there is some text highlighted, it will be replaced by the new one, just like we highlight text and start typing.
You can also create a new HTML element to insert, not only plain text. HTML element allows us to work more creative, means we can add CSS style, give it some attribute such as an id
to retrive, and anything you need to modify your element. In this case, I create a span
tag and insert it to replace the old highlighted text.
var insertedElement = editor.document.createElement('span');
insertedElement.setAttribute('style', 'color: red');
insertedElement.appendText("Hello world!");
editor.insertElement(insertedElement);
Adding some calculating logic code to convert Fahrenheit to Celsius, finally your command should be like this:
editor.addCommand("cmd_convert_F_to_C", {
exec: function (edt) {
var mySelection = editor.getSelection();
var selectedText;
if (mySelection.getType() == CKEDITOR.SELECTION_TEXT) {
if (CKEDITOR.env.ie) {
mySelection.unlock(true);
selectedText = mySelection.getNative().createRange().text;
} else {
selectedText = mySelection.getNative();
}
}
var plainSelectedText = selectedText.toString();
var regex = /[+-]?\d+(\.\d+)?/g;
if (plainSelectedText.match(regex) != null) {
var fahrenheit = plainSelectedText.match(regex).map(function (v)
{ return parseFloat(v); });
if (!isNaN(fahrenheit)) {
var insertedElement = editor.document.createElement('span');
var result = (fahrenheit - 32) * (5 / 9);
if (result > 37) {
insertedElement.setAttribute('style', 'color: red');
}
insertedElement.appendText(result + " °C");
editor.insertElement(insertedElement);
}
}
}
});
And because there is all written in JavaScript, means you can do more with this command, like contacting a DOM element or sending an AJAX request.
See Your Result
Copy some data and try to select the ...°F data, then click the button to see the result. The new °C value will be calculated and then replace the old °F value.
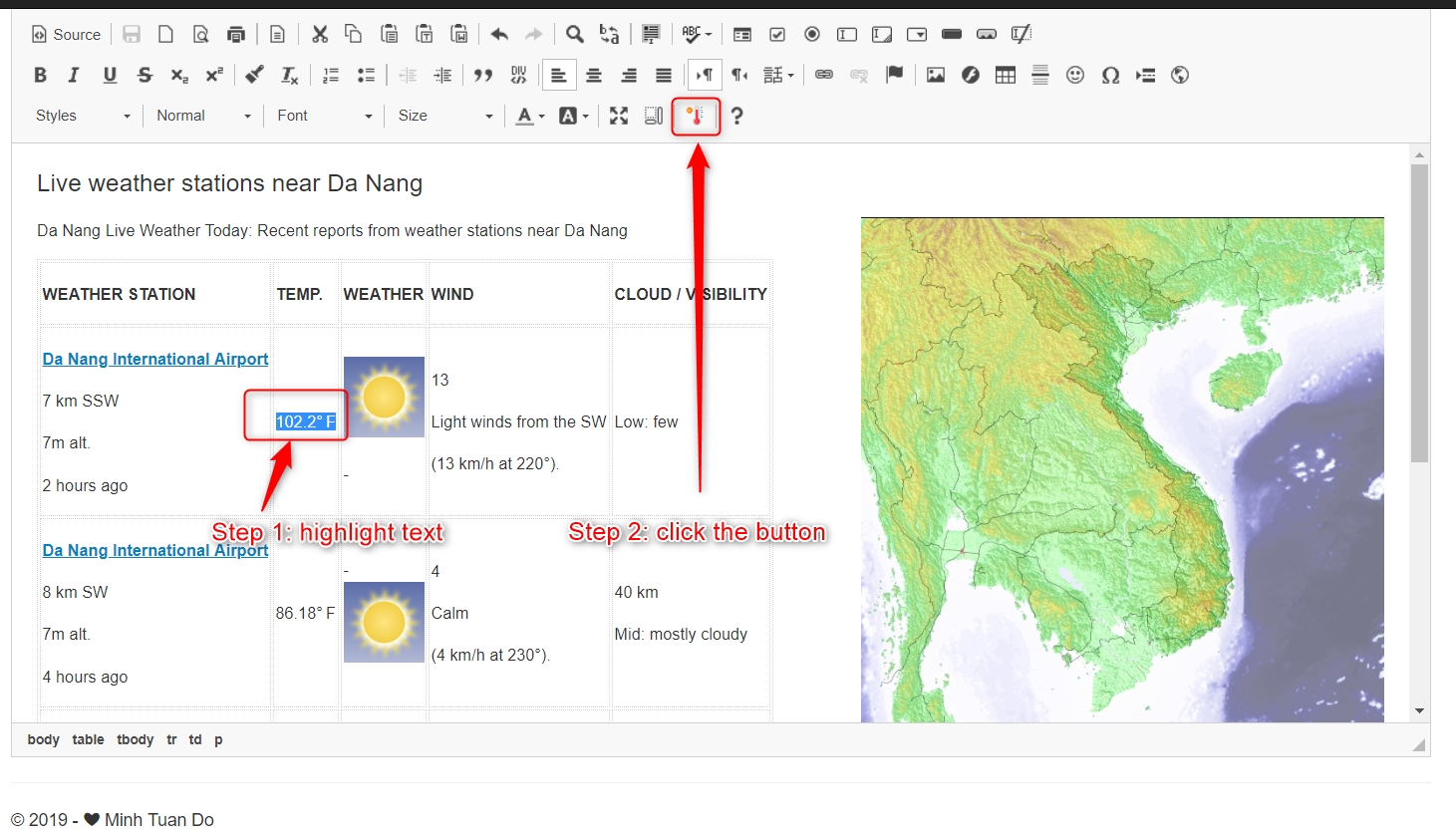
And here is the result we have achieved:
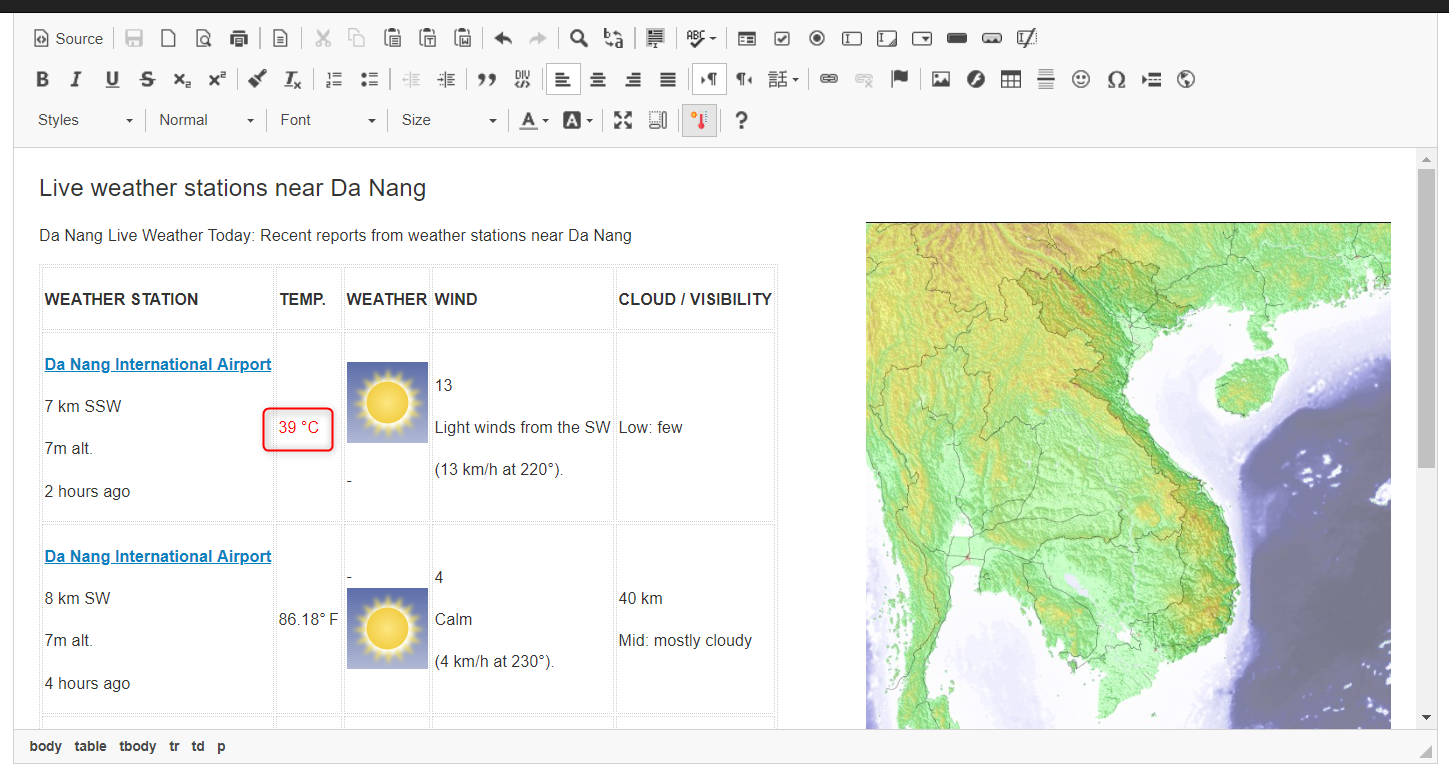
Points of Interest
CKEditor
is easy to use, provides many approaches to customize and supports a lot of features. By reading its document and research, you will find out that there is more than just a command button, but also many things we can do with it at a higher level. You can even replace its default event handler by your own (For example: you can change the way we made the text bolder when click the "B" button, by re-writing the default handler). My example project is just a little one to help you understand this, but by doing a deep research, we can optimize its features and help us a lot in editing text.
History
- 7th July, 2019: Initial post
I'm currently working in .NET technology, including Web and mobile app development.
My personal blog is at http://codingamazing.com