Introduction
Data Grid is most important part in web application. Most of the time we need to add the more value in data grid, again its also need to update/delete those data which are already stores in grid. But problem is postback. In every cases when we want to insert/update/delete from the grid, the page call the postback event and as a result it becomes to slow. In this article I developed a datagrid using Web Service and JSON. The JSON I used with Ajax. As a result I could avoid the postback event and also fast insert/delete/update with grid. But First I am discussing about some topic related the article.
- What is JSON
- JSON with Ajax
- XML
- How Web Service Work
- How JSON connect with Web Service
- How My Created Client Grid is Working
- Importance/Limitation
What is JSON
JSON means Java Script Object Notation is lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
JSON is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
These are universal data structures. Virtually all modern programming languages support them in one form or another. It makes sense that a data format that is interchangeable with programming languages also be based on these structures.
JSON with Ajax
We often use JSON with in Ajax. Ajax is a term for the ability of a webpage to request new data after it has loaded into the web browser, usually in response to user actions on the displayed webpage.
As part of the Ajax model, the new data is usually incorporated into the user interface display dynamically as it arrives back from the server. An example of this in practice might be that while the user is typing into a search box, client-side code sends what they have typed so far to a server that responds with a list of possible complete search terms from its database. These may be displayed in a drop-down list beneath the search, so that the user may stop typing and select a complete and commonly used search string directly. When it was originally described in the mid-2000s, Ajax commonly used XML as the data interchange format but many developers have also used JSON to pass Ajax updates between the server and the client
XML
XML is very familiar data storing format for any operating system. XML stores its data in structured and serialize way. Various XML-based protocols exist to represent the same kind of data structures as JSON for the same kind of data interchange purposes. When data is encoded in XML, the result is typically larger than an equivalent encoding in JSON, mainly because of XML's closing tags. In XML (as with JSON) there are alternative ways to encode the same information because some values can be represented both as child nodes and attributes. Sample data format in XML:
<Country>
<name>Bangladesh</name>
<capitalName>Dhaka</capitalName>
<shortDetails>
<Independence>1971</Independence>
<Language>Bangla</Language>
<popularSports> Cricket </popularSports>
</shortDetails>
</Country>
How Web Service Work
Web service is a technology framework, which allows machine-to-machine (M2M) interaction over the network. It can call application component. This protocol communicates with XML. It is not tied to any one operating system or programming language. It mainly works with:
- SOAP (Simple Object Access Protocol)
- UDDI (Universal Description, Discovery and Integration)
- WSDL (Web Services Description Language)
for more knowledge about web service please read those article
How JSON connect with Web Service
First JSON send a request to web server with the reference of web service. Web Server executes
the web service according to parameter. After execution the web service send SOAP message (XML formatted serialize data) to browser (via web server). JSON then fetch the xml formatted data use it.
The below figure will clear the communication between JSON and web service.
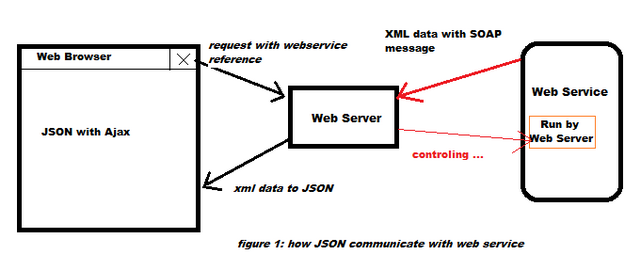
First create a web application and add a web service (.asmx) file. Now create a Get method to fetch the value form database.
[WebMethod]
public List<Employee> GetEmployeeDetails()
{
string conStr = WebConfigurationManager.ConnectionStrings["TESTDB"].ConnectionString;
DataTable dt = new DataTable();
SqlDataReader dr = null;
using (SqlConnection conn = new SqlConnection(conStr))
{
string sql = string.Format(@"Select * from [SampleInfoTable]");
SqlCommand cmd = new SqlCommand();
cmd.Connection = conn;
cmd.CommandText = sql;
cmd.CommandType = CommandType.Text;
conn.Open();
dr = cmd.ExecuteReader();
dt.Load(dr);
conn.Close();
cmd = null;
}
int countRow = dt.Rows.Count;
foreach (DataRow drEmp in dt.Rows)
{
Employee objemployee = new Employee();
objemployee.id = Convert.ToInt32(drEmp["id"].ToString());
objemployee.Name = drEmp["Name"].ToString();
objemployee.Email = drEmp["Email"].ToString();
objemployee.Phone = drEmp["Phone"].ToString();
objemployee.Address = drEmp["Address"].ToString();
list.Add(objemployee);
}
return list;
}
this method will send the data with SOAP message to web server.
Now add a web page and create the method of use JSON with Ajax in javascript region. For checking browse the webservice, you will get the XML data which actually need for JSON. Look on the
reference of Ajax url.
function GetEmployeeDetails() {
$.ajax({
type: "POST",
url: "hr_webservice.asmx/GetEmployeeDetails",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
}
url: "hr_webservice.asmx/GetEmployeeDetails",
the web service make ready the data in that link
this is the real communication between JSON and Web service. after getting the data I bind a table with javascript. also added a edit and delete control with event.
The output getting from web service which coming with xml serialization, When the success property of JSON getting the data, it calls another method with result object. Actually the whole table considered as a object Employee.
I have created a table for header value and another div for generate the table from javascript.

In the BindTable first I am checking that the table which is generated dynamically is exist or not. if exist then removing the table as I will generate with updated data.
<span class="Apple-tab-span" style="white-space: pre;">
<span class="Apple-tab-span" style="white-space: pre;"> function BindTable(Employees) {
var root = document.getElementById('mydiv');
try {
var tblId = document.getElementById('tblGridValue');
if (tblId != null) {
root.removeChild(tblId);
}
}
catch (e)
{
}
Then A table is created inside of a div. Also added the id for the table, also width, style, others property.
var tab = document.createElement('table');
tab.setAttribute("id", "tblGridValue");
tab.setAttribute("class", "tableStyle");
tab.setAttribute("cellspacing", "3px");
var tbo = document.createElement('tbody');
Lets see how the grid row is generated: I applied a foreach loop to the LIST object to get every row of LIST, and
var row, cell;
$.each(Employees, function (index, employee) {
row = document.createElement('tr');
row.setAttribute("class", "tableRaw");
I created row for each row. As my each list cell contains 5 column for that I needed to split/detect and that why I again used a loop for each column. In the loop I am creating <td> for each column.

the row is binding form the getting object of result object, as a result the number of row is generated by the number of row of each row. the number of column is binding according to the values of object. the property also added for each column
for (var j = 0; j < 5; j++) {
cell = document.createElement('td');
cell.setAttribute("width", "200px");
var empId = employee.id;
var empName = employee.Name;
var empEmail = employee.Email;
var empPhone = employee.Phone;
var empAddress = employee.Address;
if (j == 0) {
var hiddenId = document.createElement("input");
hiddenId.setAttribute("type", "hidden");
hiddenId.setAttribute("id", "hfRow_" + employee.Id);
hiddenId.setAttribute("value", employee.Id);
cell.appendChild(hiddenId);
cell.appendChild(document.createTextNode(employee.Name));
}
else if (j == 1) {
var spanValue = document.createElement("span");
cell.setAttribute("width", "200px");
spanValue.setAttribute("display", "inline-block");
spanValue.appendChild(document.createTextNode(employee.Email));
cell.appendChild(spanValue);
}
else if (j == 2) {
cell.setAttribute("width", "200px");
cell.appendChild(document.createTextNode(employee.Phone));
}
else if (j == 3) {
cell.setAttribute("width", "200px");
cell.appendChild(document.createTextNode(employee.Address));
}
there used some hidden field to store the primary key and action mode of button. the primary key is used for update and delete. the action mode is used for tag that insert/update/delete which action request button is sending.
There also created the edit and delete button dynamically at last column
else if (j == 4) {
var element = document.createElement("img");
element.setAttribute("src", "images/edit-icon.gif");
element.setAttribute("width", "15px");
cell.setAttribute("width", "100px");
element.setAttribute("onclick", "EditMode('"
+ empId + "','"
+ empName + "','"
+ empEmail + "','"
+ empPhone + "','"
+ empAddress + "')");
cell.appendChild(element);
var elementDelete = document.createElement("img");
elementDelete.setAttribute("src", "images/DeleteRed.png");
elementDelete.setAttribute("width", "15px");
elementDelete.setAttribute("onclick", "return DeleteMode('" + empId + "')");
cell.appendChild(elementDelete);
}
the button also added the click event which will fire on click for edit mode.

So, when edit image button click the EditMode() method fire
function EditMode(empId, empName, empEmail, empPhone, empAddress) {
var hfEditedId = document.getElementById('hfEditedId');
var txEditedName = document.getElementById('txEditedName');
var txEditedEmail = document.getElementById('txEditedEmail');
var txEditedPhone = document.getElementById('txEditedPhone');
var txEditedAddress = document.getElementById('txEditedAddress');
var hfActionMode = document.getElementById('hfActionMode');
var btnEntry = document.getElementById('btnEntry');
hfEditedId.value = empId;
txEditedName.value = empName;
txEditedEmail.value = empEmail;
txEditedPhone.value = empPhone;
txEditedAddress.value = empAddress;
hfActionMode.value = "UPDATE";
btnEntry.value = "Update";
}
at the same time the the mode changed easy way. In this state the hidden field store the selected rows primary key.

after click the update button the edit method fire which is again sending the edited value to direct database
function EditEmployeeData() {
var editedName = document.getElementById('txEditedName');
var editedEmail = document.getElementById('txEditedEmail');
var editedPhone = document.getElementById('txEditedPhone');
var editedAddress =document.getElementById('txEditedAddress');
var hfEditedId = document.getElementById('hfEditedId');
var hfActionMode = document.getElementById('hfActionMode');
if (BlankValidation(editedName, editedEmail, editedPhone, editedAddress) == true) {
$.ajax({
type: "POST",
url: "hr_webservice.asmx/EditEmployeeData",
data: "{ 'employeeId': '" + hfEditedId.value
+ "','editedName': '" + editedName.value
+ "', 'editedEmail': '" + editedEmail.value
+ "','editedPhone':'" + editedPhone.value
+ "','editedAddress':'" + editedAddress.value
+ "','action_mode':'" + hfActionMode.value + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
ResetText();
}
}
and also delete method calls onclick event of generated image button
function DeleteMode(deleteEmpId) {
var agree = confirm("Are you sure you want to delete this information ?");
if (agree) {
$.ajax({
type: "POST",
url: "hr_webservice.asmx/DeleteEmployeeData",
data: "{ 'employeeId': '" + deleteEmpId + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
BindTable(response.d);
},
failure: function (msg) {
alert(msg);
}
});
}
}
when JSON calls the edit method to webservice, the web service method fires and the method directly working with database.
[WebMethod]
public List<Employee> EditEmployeeData(string employeeId, string editedName, string editedEmail, string editedPhone, string editedAddress, string action_mode)
{
string conStr = WebConfigurationManager.ConnectionStrings["TESTDB"].ConnectionString;
int rowsInserted = 0;
using (SqlConnection conn = new SqlConnection(conStr))
{
SqlCommand cmd = new SqlCommand();
cmd.Connection = conn;
cmd.CommandText = "sp_sample_info";
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add("@id", employeeId);
cmd.Parameters.Add("@Name", editedName);
cmd.Parameters.Add("@Email", editedEmail);
cmd.Parameters.Add("@Phone", editedPhone);
cmd.Parameters.Add("@Address", editedAddress);
cmd.Parameters.Add("@Action", action_mode);
conn.Open();
rowsInserted = cmd.ExecuteNonQuery();
conn.Close();
cmd = null;
}
list = GetEmployeeDetails();
return list;
}
delete action doing at the same way.
sample output of client side data grid:

Importance/Limitation
Importance
- When using JSON with Ajax, a web application can request only the grid command(insert/update/delete) that needs to be updated, thus drastically reducing bandwidth usage and specially load time.
- The web application will be operated faster or more responsive, even if the application has not changed on the server side.
- Ajax enable to reduce connections to the server, since scripts and style sheets only have to be requested once.
- State can be maintained throughout a Web site such as Javascript variables.
LimitationAny user whose browser does not support Ajax or JavaScript, or simply has JavaScript disabled, will not be able to use its functionality
History
25-November-2012