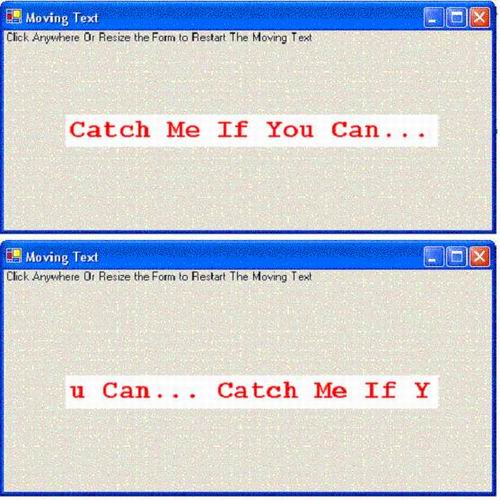
Overview
This application demonstrates how to create a "string loop" in C#. A common
use of "string loops" is in web advertisements, where the slogan or product name
appears to be revolving in a circle. The code creates a rectangle in the middle
of the client area that is just large enough to fit the entire message, minus
any trailing white space. Clicking on the form or resizing it will cause the
"string loop" to restart.
Points to Notice
Rather than use a string to represent the message ("Catch Me If You Can…" ) it
is far more efficient and intuitive to use the StringBuilder
class, found in the
System.Text
namespace. StringBuilder
allows you to directly manipulate a string,
rather than having to make copies of a regular string.
The manipulation of the StringBuilder
’s internal string is quite
straightforward:
private void DrawMovingText()
{
Graphics grfx = CreateGraphics();
Font font = new Font( "Courier New", 20, FontStyle.Bold );
string spaces = " ";
StringBuilder str =
new StringBuilder( "Catch Me If You Can..." + spaces );
Rectangle rect = CreateRect( grfx, str, font );
int numCycles = str.Length * 3 + 1;
for( int i = 0; i < numCycles; ++i )
{
grfx.FillRectangle( Brushes.White, rect );
grfx.DrawString( str.ToString(), font, Brushes.Red, rect );
str.Append( str[0] );
str.Remove( 0, 1 );
Thread.Sleep( 150 );
}
grfx.Dispose();
}
To avoid having DrawMovingText()
eat up all of the CPU's
attention it is placed on a worker thread. Every time the user clicks on the
form or resizes it, the thread is killed and reborn. Obviously, if you wanted to
alter the speed at which the letters "move" you would simply adjust the number
of milliseconds that the thread sleeps for.
private void Form_Load( object sender, System.EventArgs e )
{
StartThread();
}
private void Form_MouseUp(object sender,
System.Windows.Forms.MouseEventArgs e)
{
StartThread();
}
private void Form_Resize( object sender, System.EventArgs e )
{
StartThread();
}
private void label_Click(object sender, System.EventArgs e)
{
StartThread();
}
private void StartThread()
{
thread.Abort();
Thread.Sleep( 100 );
Invalidate();
thread = new Thread(new ThreadStart(DrawMovingText));
thread.Start();
}
The final point of interest is how the size and location of the rectangle that
contains the message are determined. The Graphics
class
has a MeasureString
method that will give you the width
or height of the string that you are passing in. Those are used for the width
and height of the rectangle. You then use those values in tandem with the
dimensions of the form to determine the coordinates of where the rectangle will
originate from.
private Rectangle CreateRect
( Graphics grfx, StringBuilder str, Font font )
{
int w = (int)grfx.MeasureString(str.ToString(), font).Width + 5;
int h = (int)grfx.MeasureString( str.ToString(), font ).Height;
int x = (int)this.Width / 2 - w / 2;
int y = (int)this.Height / 2 - h;
return new Rectangle( x, y, w, h );
}