Here, I am going to show you a simple preferred time control, where you can select the day of the week and the time of the day. This can be used in lots of places where you may need to display the users' preferred times. A sample screenshot is attached below.
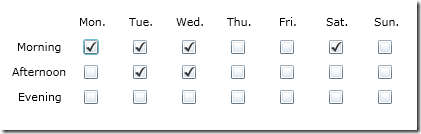
This control is developed using Silverlight 3 and VS2008. I am also attaching the source code with this post. This is a very basic example. You can download and customize it further according to your requirements.
I am trying to explain in a few words how this control works and what are the different ways in which you can customize it further.
File: PreferredTimeControl.xaml, in this file, I have just hardcoded the controls and their positions which you can see in the screenshot above. In this example, to change the start day of the week and time, you will have to go and change the design in XAML file. It's not controlled by your properties or implementation classes. You can also customize it to change the start day of the week, Language, Display format, styles, etc.
File: PreferredTimeControl.xaml.cs, in this control using the code below, first I am taking all the checkboxes from my form and storing it in the Global Variable, which I can use across my page.
List<CheckBox> checkBoxList;
#region Constructor
public PreferredTimeControl()
{
InitializeComponent();
GetCheckboxes();
}
#endregion
#region Helper Methods
private List<CheckBox> GetCheckboxes()
{
checkBoxList = new List<CheckBox>();
foreach (UIElement element in LayoutRoot.Children)
{
if (element.GetType().ToString() == "System.Windows.Controls.CheckBox")
{
checkBoxList.Add(element as CheckBox);
}
}
return checkBoxList;
}
Then I am exposing the two methods which you can use in the container form to get and set the values in these controls.
public void SetAvailibility(string selectedTimings)
{
foreach (CheckBox chk in checkBoxList)
{
chk.IsChecked = false;
}
if (!String.IsNullOrEmpty(selectedTimings))
{
string[] selectedString = selectedTimings.Split(',');
foreach (string selected in selectedString)
{
foreach (CheckBox chk in checkBoxList)
{
if (chk.Tag.ToString() == selected)
{
chk.IsChecked = true;
}
}
}
}
}
public string GetAvailibility()
{
string selectedText = string.Empty;
foreach (CheckBox chk in GetCheckboxes())
{
if (chk.IsChecked == true)
{
selectedText = chk.Tag.ToString() + "," + selectedText;
}
}
return selectedText;
}
In my example, I am using the matrix format for Day and Time, for example Monday=1, Tuesday=2, Wednesday=3, Thursday = 4, Friday = 5, Saturday = 6, Sunday=7. And Morning = 1, Afternoon =2, Evening = 3. So if I want to represent Morning-Monday, I will have to represent it as 11, Afternoon-Tuesday as 22, Morning-Wednesday as 13, etc. And in the other way, to set the values in the control, I am passing the values in the control in the same format as:
preferredTimeControl.SetAvailibility("11,12,13,16,23,22");
So this will set the checkbox value for Morning-Monday, Morning-Tuesday, Morning-Wednesday, Morning-Saturday, Afternoon of Tuesday and Afternoon of Wednesday.
To implement this control, first I have to import this control in xmlns
namespace as:
xmlns:controls="clr-namespace:PreferredTimeControlApp"
and finally put in your page wherever you want:
<Grid x:Name="LayoutRoot"
Style="{StaticResource LayoutRootGridStyle}">
<Border x:Name="ContentBorder"
Style="{StaticResource ContentBorderStyle}">
<controls:PreferredTimeControl
x:Name="preferredTimeControl">
</controls:PreferredTimeControl>
</Border>
</Grid>
And in the code behind, you can just include this code:
private void InitializeControl()
{
preferredTimeControl.SetAvailibility("11,12,13,16,23,22");
}
And you are ready to go. For more details, you can refer to my code attached.
I know there can be an even simpler and better way to do this. Let me know if you have any other ideas.
Sorry guys, I have used Silverlight 3 and VS2008, as from the system I am uploading, this is still not upgraded, but you can use the same code with Silverlight 4 and VS2010 without any changes. May be, it will just ask you to upgrade your project which will take care of the rest. You can also refer to a better formatted version of this post here.
Thanks for reading.
~Brij
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.