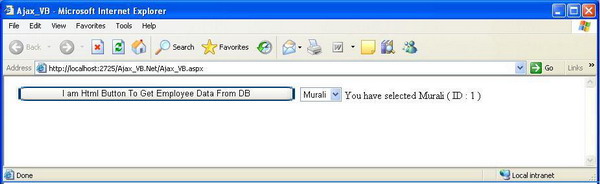
Introduction
AJAX stands for Asynchronous JavaScript and XML. Beginners may find it hard when they want to learn AJAX. They may get confused when they see samples using Atlas. This article is mainly for beginners of AJAX. The sample code is very easy and self-understandable. This code does not use Ajax.dll. It uses the XmlHttp
object to do the AJAX implementation.
Using the code
Download the source code, unzip it, and configure it in your IIS. The folder "Ajax_VB.Net" contains a sub-folder named "DB Table". This sub-folder contains a SQL file. Execute the SQL in your local SQL Server. A table with the name "employee" will be created. Add some more data into the table with an Insert
query in the SQL file.
Execute the project Ajax_VB.Net. Click the button. The button is an HTML button. It takes employee data from the table 'employee' without page post-back and populates the data in the dropdownlist. When a particular record is selected in the dropdownlist, the selected employee name and employee ID will be displayed in the label.
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML. It is a browser technology. It is a combination of JavaScript and XML. Data is transferred in the form of XML. The XMLHttp
object is used in AJAX for data transfer.
Why AJAX?
AJAX is used to eliminate round-trips to the server when a request is posted to the server. In simple terms, we can say that when we want to access a database without page refresh, we can use AJAX.
The GetData()
function calls the GetEmployeeDetails page. In that page, employee details are retrieved from the database and returned back to the calling JavaScript function. From the Response
object, the return value is evaluated and bound to the dropdownlist.
Sample source code
var xmlHttp
function GetData()
{
xmlHttp=GetXmlHttpObject()
if (xmlHttp==null)
{
alert ("Browser does not support HTTP Request")
return;
}
var url="GetEmployeeDetails.aspx"
xmlHttp.onreadystatechange=stateChanged
xmlHttp.open("GET",url,true)
xmlHttp.send(null)
}
function stateChanged()
{
if (xmlHttp.readyState==4 || xmlHttp.readyState=="complete")
{
var response=xmlHttp.responseText;
response=response.substring(0,response.indexOf("<!DOCTYPE")-4);
if(response=="Empty")
{
alert("No Record Found !!!");
}
else if(response=='Error')
{
alert("An Error occured in accessing the DataBase !!!");
}
else
{
var arr=response.split("~");
var empID=arr[0].split(",");
var empName=arr[1].split(",");
document.getElementById('dlistEmployee').length=0;
for(var i=0;i<empID.length;i++)
{
var o = document.createElement("option");
o.value = empID[i];
o.text = empName[i];
document.getElementById('dlistEmployee').add(o);
}
}
}
}
function GetXmlHttpObject()
{
var objXMLHttp=null
if (window.XMLHttpRequest)
{
objXMLHttp=new XMLHttpRequest()
}
else if (window.ActiveXObject)
{
objXMLHttp=new ActiveXObject("Microsoft.XMLHTTP")
}
return objXMLHttp
}
function display()
{
var selIndex=document.getElementById("dlistEmployee").selectedIndex;
var empName=document.getElementById("dlistEmployee").options(selIndex).text;
var empID=document.getElementById("dlistEmployee").options(selIndex).value;
document.getElementById("lblResult").innerHTML="You have selected " +
empName + " ( ID : " + empID + " )";
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.