Understanding AJAX






2.38/5 (7 votes)
Let's cook up a simple AJAX example
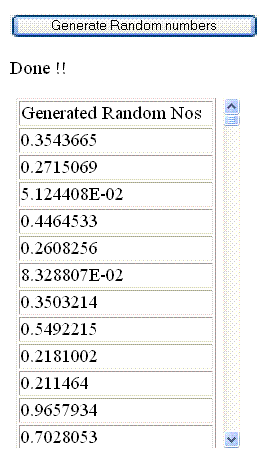
Introduction
Just imagine a very massive webpage providing a lot of functionalities with lot of data, all coming from the server. Now the user wants to get some information from the server that may affect only a small portion of this page but when he submits his request, the whole page renders again unnecessarily, taking lot of time and populating each and every thing again.
This has been a very irritating problem in the past but now, AJAX (Asynchronous JavaScript And XML) has come up as a solution of the whole page rendering. With the blessings of AJAX, we can update/refresh only the necessary part of our webpage instead of rendering the whole page itself.
Our GOAL in this Example
Our aim is to get familiar with AJAX. So here we are going to cook up a very simple example by focusing on the usage of AJAX rather than making the business logic and presentation complex by involving database connectivity or other complex logics. (Sure we all already know databases. Even if we don't know, we will learn that some other time.)
We'll generate random numbers on the Server (generateRandomNos.asp) and then we will call this server side code on our simple HTML page (showRandomNos.html) by means of AJAX. We'll notice that only the part of our HTML page (showRandomNos.html) will be updated instead of getting the whole page refreshed.
Using the Code
Our first page is just an HTML page that displays randomly generated numbers when user clicks on the button "Generate Random numbers" BUT the science used here is AJAX. Only a small division is updated instead of rendering the whole page to accomplish the user's request.
onclick='JavaScript:generateRandomNumbers("generateRandomNos.asp")'
On the button's Onclick
event, we call a JavaScript function and pass the URL of the *.asp page (that will generate the Random numbers at the server) like shown above:
function generateRandomNumbers(targetURL)
{
var xmlHttpReq = false;
// FOR IE
if (window.ActiveXObject) {
xmlHttpReq = new ActiveXObject("Microsoft.XMLHTTP");
}
// FOR OTHER BROWSERS
else if (window.XMLHttpRequest) {
xmlHttpReq = new XMLHttpRequest();
}
xmlHttpReq.open('POST', targetURL, true);
xmlHttpReq.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xmlHttpReq.onreadystatechange =
function() { // START OF Callback function
if (xmlHttpReq.readyState == 4) {
document.getElementById("result").innerHTML =
xmlHttpReq.responseText;
document.getElementById("generationStatus").innerHTML= "Done !!";
}
else
{
document.getElementById("generationStatus").innerHTML=
"Generating Random numbers...";
}
} // END OF Callback function
xmlHttpReq.send();
}
For processing the server side code, we need to send a request to the server. As AJAX is a browser specific technology, we've to make a browser specific object such as in case of Internet Explorer; we will create it as shown below:
new ActiveXObject("Microsoft.XMLHTTP");
Now we will call the server side code using the XMLHttpRequest
object:
xmlHttpReq.open('POST', targetURL, true);
Different states of our xmlHttpRequest
object will let us know how far we have reached. Its readyState
4 tells the browser that our request has successfully been completed and control has been returned to the browser. readyState
s can be of four different types as listed below:
- 0 = uninitialized
- 1 = loading
- 2 = loaded
- 3 = under process
- 4 = complete
if (xmlHttpReq.readyState == 4) {
document.getElementById("result").innerHTML = xmlHttpReq.responseText;
document.getElementById("generationStatus").innerHTML= "Done !!";
}
else
{
document.getElementById("generationStatus").innerHTML=
"Generating Random numbers...";
}
We can also get the server's response in the form of TEXT
or XML
such as:
// FOR TEXT Response
xmlHttpReq.responseText;
// FOR XML Response
xmlHttpReq.responseXML;
In the end, let's have a look at our server side code below. It just generates random numbers using rnd
method:
for i = 1 to 9999
Randomize()
response.Write(rnd)
next
We've successfully cooked our first AJAX example and to taste it, just place both the files on your web server and then run the web page showRandomNos.html...
Enjoy!
History
- 8th October, 2006: Initial post