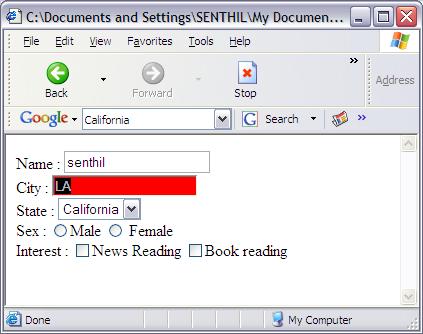
Introduction
When the user tabs off from an element, browsers by default just draw a border around the focused element. Sometimes it is hard to notice and if we want to help the user
to find this easily, we can change the background color of the currently focused element. It will help them easily identify the focused element.
How do we do this?
We need some JavaScript to set and reset the background color.
var bkColor = "red";
function getEvent(e){
if(window.event != null) {
return event;
}
return e;
}
function setBKColor(e){
e = getEvent(e);
var src = e.srcElement || e.target;
if(src != null) {
src.style.bkColor = src.style.backgroundColor;
src.style.backgroundColor = bkColor;
}
}
function reSetBKColor(e){
e = getEvent(e);
var src = e.srcElement || e.target;
if(src != null) {
src.style.backgroundColor = src.style.bkColor;
}
}
You can set any color for the global variable bkColor
which will be used for setting the background color. You can use an RGB color code or a color name such
as red, blue, green, etc. I have assigned red as my focus background color.
setBKColor
will be assigned to the onFocus
event. Whenever the element receives focus, the browser will call this method. In that method, we store the original
bkColor
in a temp variable and we just set the global bkColor
variable to the current element's background color.
reSetBKColor
will be assigned to the onBlur
event. Whenever the element loses focus, the browser will call this method. In that method, we just set
the temp bkColor
to the current element's background color, and it will reset to the original background color.
We can directly assign those JavaScripts to every HTML form element tag or write a global event handler to attach the event to all form elements.
I will show you both ways.
Adding to the HTML tag:
<input type="text" onfocus="setBkColor(event);" onblur="reSetBKColor(event);"
<input type="radio" id= "rd1" onfocus="setBkColor(event);" onblur="reSetBKColor(event);"
If you like to add a global handler:
<script language="javascript">
function attachEvent(name,element,callBack) {
if (element.addEventListener) {
element.addEventListener(name, callBack,false);
} else if (element.attachEvent) {
element.attachEvent('on' + name, callBack);
}
}
function setListner(eve,func) {
var ele = document.forms[0].elements;
for(var i = 0; i < ele.length;i++) {
element = ele[i];
if (element.type) {
switch (element.type) {
case 'checkbox':
case 'radio':
case 'password':
case 'text':
case 'textarea':
case 'select-one':
case 'select-multiple':
attachEvent(eve,element,func);
}
}
}
}
setListner("focus",setBKColor);
setListner("blur",reSetBKColor);
</script>
We need to call the setListner
function for the focus
and blur
functions. It will loop through all elements and attach the particular
function (second argument) to the event (first argument).
Now if you launch the HTML and tab off from one field to another or click inside a field, you will see the previous element's bkColor
reset to the original bkColor
and the focused element will change to the new bkColor
.