One of Code First main features is the Fluent API. This API can help you to configure the model in order to shape it (and the database) better. In this post, I’m going to show a simple example for how to use the Fluent API. Pay attention that the details I provide might change in the future since it's only a CTP and not a release.
The Example Model
In the example, I’m going to use the following model:
public class SchoolEntities : DbContext
{
#region Properties
public DbSet<Course> Courses { get; set; }
public DbSet<Department> Departments { get; set; }
#endregion
#region Methods
protected override void OnModelCreating(
System.Data.Entity.ModelConfiguration.ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
}
#endregion
}
public partial class Course
{
#region Properties
public int CourseID { get; set; }
public string Title { get; set; }
public string Days { get; set; }
public DateTime Time { get; set; }
public string Location { get; set; }
public int Credits { get; set; }
public int DepartmentID { get; set; }
public virtual Department Department { get; set; }
#endregion
}
public class Department
{
#region Properties
public int DepartmentID { get; set; }
public string Name { get; set; }
public decimal Budget { get; set; }
public DateTime StartDate { get; set; }
public int Administrator { get; set; }
public virtual ICollection<Course> Courses { get; set; }
#endregion
}
Fluent API Example
After you create your model, there are a lot of ways to configure the model using the Fluent API. The main place to do that is in the OnModelCreating
method which you override in the DbContext
. That method gets a ModelBuilder
instance which can be used to configure the model. The following example shows how to write some configuration with the API:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
modelBuilder.Entity<Department>().
Property(d => d.Name).
IsRequired().
HasMaxLength(50);
modelBuilder.Entity<Department>().
Property(d => d.DepartmentID).
HasDatabaseGenerationOption(DatabaseGenerationOption.None);
modelBuilder.Entity<Department>().
HasMany(d => d.Courses).
WithRequired(c => c.Department).
HasForeignKey(c => c.DepartmentID).
WillCascadeOnDelete();
modelBuilder.Entity<Department>().
Ignore(d => d.Administrator);
modelBuilder.Entity<Course>().
Property(c => c.Title).
IsRequired().
HasColumnName("Name");
}
What is Configured?
Let's explain what you see in the above code. When I want to configure an entity, I use the Entity method with the entity as a generic parameter. Then I’m exposed to the Fluent API and can start configuring my model. In the first line, I configure the Name
property of the department as required and with length of no more than 50 characters. In the second line, I configure the DepartmentID
as non database generated (since by default all the ID will be database generated). The third line creates a one to many relation between the department and its course collection. You first use the HasMany
to indicate the many side and then use the WithRequired
to indicate the one side of the relation. The HasForeignKey
indicates the foreign key on the many side. The last method, WillCascadeOnDelete
, will add cascade delete on the entity graph. The fourth line will force the model to ignore the administrator property of the department and therefore it won’t be generated in the database. The fifth line indicates that the Title
property is required and that in the database the column name will be Name
instead of Title
. Here is a simple example of creating data and inserting it to the database:
class Program
{
static void Main(string[] args)
{
using (SchoolEntities context = new SchoolEntities())
{
var department = new Department
{
DepartmentID = 1,
Administrator = 2,
Budget = 100000,
Name = "Data Access",
StartDate = DateTime.Now
};
var course = new Course
{
Credits = 2,
Days = "MF",
Location = "Class 1",
Time = DateTime.Now,
Title = "Entity Framework",
Department = department,
};
context.Departments.Add(department);
context.SaveChanges();
}
}
}
Running this example will generate a new database by the name ClassLibrary1.SchoolEntities
with all the configurations that were written in the OnModelCreating
method. If you want to change the generated database name (which is taken from the namespace
and context name by default), you need to create a constructor to the DbContext
with a call for the base constructor that gets a string
as parameter:
public SchoolEntities() :
base("MySchool")
{
}
The resulting database is as shown below:
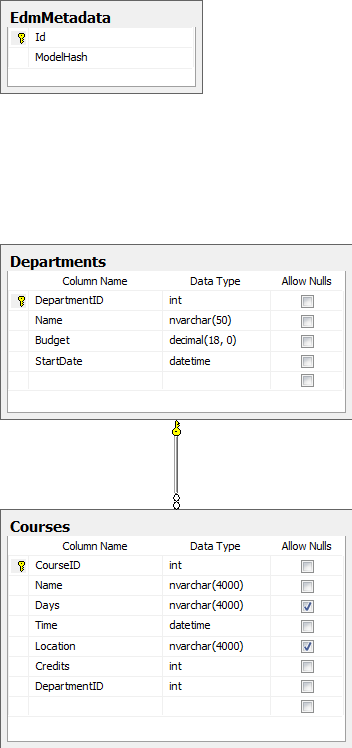
Summary
Let's sum up, Code First has a very interesting feature of fluent API. The fluent API for model configuration is easy to understand and use. In this post, I showed and explain a small portion of the API.
Gil Fink is a web development expert and ASP.Net/IIS Microsoft MVP. He is the founder and owner of sparXys. He is currently consulting for various enterprises and companies, where he helps to develop Web and RIA-based solutions. He conducts lectures and workshops for individuals and enterprises who want to specialize in infrastructure and web development. He is also co-author of several Microsoft Official Courses (MOCs) and training kits, co-author of "Pro Single Page Application Development" book (Apress) and the founder of Front-End.IL Meetup. You can read his publications at his website: http://www.gilfink.net