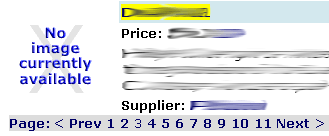
Introduction
If you use DataGrid
s on web pages that receive a lot of hits, and they only display information and are not to edit data, then high speed, small size might be what you need.
The standard DataGrid
is not optimised for search engine indexing as it uses JavaScript postbacks to jump to the next pages. There is also the slight possibility that your users might not have JavaScript enabled on their browser. We will have a look at implementing a custom DataGrid pager which incorporates "< Prev" and "Next >" links as well as replaces the postback buttons with standard anchor tags.
Background
As the majority of internet traffic is generated by search engines, having as many relevant pages spidered, and hopefully indexed, is the main concern for most webmasters. I consider the DataGrid
as one of the most powerful controls available to the ASP.NET developer. It does, however, have two disadvantages to its use:
- It creates a bloated Viewstate which could cause search engines to download unusable data and thereby skipping a page, as some spiders only read the top most part of a web page.
- The pager does not create search engine friendly links that are optimised for spiders due to the use of JavaScript postback.
It might be easier to implement custom paging than writing custom code yourself, but implementing a custom solution might just be the answer to getting more pages spidered as you will be using anchor tags instead of postbacks to handle the paging.
Using the code
There are two aspects to the code:
- The customized paging of the
DataGrid
. - The custom paging in the
Page_Load
event of the form.
All the work to customise the pager is done in the ItemCreated
event of the DataGrid
. The main thing to remember is that the pager is made out of a Label
control (the unlinked and therefore current page number) and several DataGridLinkButton
s, which are the active buttons. These DataGridLinkButton
s are the "culprits" that we want to replace with standard HTML anchor tags.
More descriptive comments are available inline.
The Datagrid_ItemCreated event
Private Sub CustomGrid_ItemCreated(ByVal sender As Object, _
ByVal e As System.Web.UI.WebControls.DataGridItemEventArgs) _
Handles CustomGrid.ItemCreated
If e.Item.ItemType = ListItemType.Pager Then
Dim Pager As TableCell = CType(e.Item.Controls(0), TableCell)
Dim myEnumerator As IEnumerator = Pager.Controls.GetEnumerator()
Dim labelPos As Int16
Dim blTag As Boolean = False
Dim strObj As String
Dim litTemp As Literal
Dim y As Int16
resumeHere:
y = 0
While (myEnumerator.MoveNext())
Dim myObject As Object = myEnumerator.Current
y += 1
If myObject.GetType().Equals(GetType(Label)) Then
If blTag = False Then
labelPos = CType(myObject, Label).Text - 1
blTag = True
End If
ElseIf myObject.GetType.ToString.Equals("System." & _
"Web.UI.WebControls.DataGridLinkButton") Then
Pager.Controls.Remove(myObject)
litTemp = New Literal
litTemp.Text = "<a href=" & chr(34) & _
"thispage.aspx?page=" & _
CStr(CInt(CType(myObject, LinkButton).CommandArgument) - 1) _
& chr(34) & ">" & _
CType(myObject, LinkButton).CommandArgument & "</a>"
Pager.Controls.AddAt(y - 1, litTemp)
myEnumerator = Pager.Controls.GetEnumerator()
GoTo resumeHere
End If
End While
Dim lbNext As New Literal
lbNext.Text = "<a href=" & chr(34) & _
"thispage.aspx?page=" & labelPos + 1 _
& chr(34) & "> Next ></a>"
Dim lbPrev As New Literal
lbPrev.Text = "<a href=" & chr(34) & _
"thispage.aspx?page=" & _
labelPos - 1 & chr(34) & ">< Prev </a>"
Dim endPagingIndex As Integer = (Pager.Controls.Count - 1)
If (labelPos > 0) Then
endPagingIndex += 1
Pager.Controls.AddAt(0, lbPrev)
End If
y = CType(sender, DataGrid).PageCount - 1
If (labelPos < y) Then
endPagingIndex += 1
Pager.Controls.AddAt(endPagingIndex, lbNext)
End If
Dim InfoText As Label = New Label
InfoText.ID = "it"
InfoText.Font.Bold = True
InfoText.Text = "Page: "
Pager.Controls.AddAt(0, InfoText)
End If
End Sub
The Page_Load
event is responsible for catching the page number passed to the form via the page
parameter in the querystring. You now have the opportunity to either let the DataGrid
do the data paging, or you can customise your database querystring to limit the returned results to only include the necessary records.
If using MySQL as your data engine, you can use the LIMIT
function to restrict the number of records returned. E.g., "SELECT * FROM SampleTable WHERE SampleField like ='sampleQuery%' LIMIT " & intP * 25 & ", 25"
. If using a similar query, you will only receive 25 records starting at intP * 25
. You can therefore limit the size of the data returned and speed up your page.
The Page_Load event
Private Sub Page_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
Dim intP as Integer
If Not Request.QueryString.Item("page") Is Nothing Then
intP = Request.QueryString.Item("page").ToString.Trim
CustomGrid.DataSource = YourDataSet
CustomGrid.DataBind()
CustomGrid.CurrentPageIndex = intP
End Sub
As paging is now manually handled, you can disable the Viewstate for the DataGrid
. Well, that is if you are only using the Viewstate so that you could page through the DataGrid
. If you need to be able to edit the grid, then you still need the Viewstate enabled.
By implementing custom paging, you can achieve three goals:
- Disable the Viewstate for the
DataGrid
and thereby save bandwidth. - Get more pages indexed by search engines.
- Implement custom paging using customised SQL queries and thereby lower the database time needed to page through the query.
Points of interest
I hate to use the Goto
function while coding, but this is the first time that I did not have an alternative than to use Goto
. The pager is not well documented, and I had to make due with the limited knowledge that was available. When changes are made to the enumerator, you have to reload the enumerator and therefore start from the beginning of the enumerator, therefore the use of the Goto
.
This article is based on the articles of Munsifali Rashid and Razi Bin Rais regarding customising the DataGrid pager.
Working sample
This article was born out of necessity as I had to find a way to limit database connection time and get more pages indexed. You can see a working example of the code on the search page of SearchForMore.Info when you do a search. View the links in the pager at the bottom of the DataGrid.