Introduction
Sending SMS messages using Twilio is expected to work on any IoT device that supports Intel microcontrollers running on Ubuntu.
Twilio allows software developers to programmatically receive SMS and Multimedia Messaging Service (MMS) messages to their Twilio phone numbers and reply back with SMS and MMS messages. You can send SMS and MMS messages using Twilio’s REST API, track SMS conversations, and send SMS messages during phone calls.
About the UP Squared* Board
The UP Squared board is a low-power and high performance platform ideal for Internet of Things (IoT) applications. Depending on your performance requirements, you can purchase an UP Squared board based on either the Intel® Celeron® processor (N3350) or Intel® Pentium® processor (N4200). For more information, visit the official UP Squared site.
Gather Your Materials
Hardware
The hardware components used in this project are listed below:
For detailed assembly and instructions for powering on the UP Squared board, check out the UP Squared Get Started Guide at the Intel® Developer Zone.
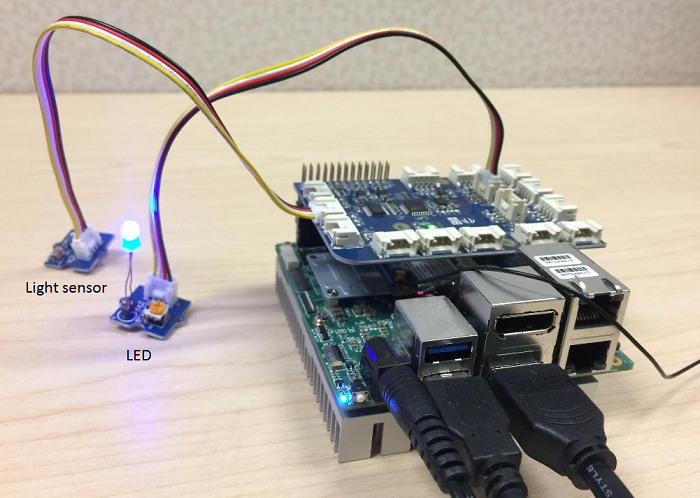
Figure 1: Hardware
Create a Twilio* Account
To receive SMS and MMS messages, you need an SMS-enabled Twilio phone number. To get your own dedicated phone number, sign up for a Twilio trial account. Once SMS-enabled Twilio access is granted, go to your account dashboard to find your Twilio Account SID and Twilio Auth Token.

Figure 2: Twilio Credentials from Account Dashboard
Install the Ubuntu* Operating System
Before you begin, the Ubuntu* operating system should be installed on the UP Squared platform. For this guide, the Ubuntu 16.04.1 LTS 64 bit desktop was used. For more information on how to install the Ubuntu operating system, visit https://wiki.up-community.org/Ubuntu.
When you boot the Up Squared board after Ubuntu desktop was installed, a menu will appear with options to boot either Ubuntu or other operating system. Ubuntu is highlighted and will be executed by default.
Install Twilio*
-
To ensure that the Ubuntu operating system is up to date and dependent Ubuntu packages are installed, open a command prompt (terminal) and type:
sudo apt-get update
-
Install the Node Version Manager (NPM) package:
sudo apt-get install npm
-
Install the Twilio node package from NPM:
npm install twilio
Install Ubuntu* Kernel
The default kernel in the Ubuntu Core doesn’t give access to the GPIO pins. Install the required kernel below to have access to the GPIO pins.
-
Before updating the kernel, use the following command to check whether you have the right kernel for Up Squared.
uname -srv
The kernel for Up Squared should look like this:
Linux 4.10.0-42-generic #5000~upboard9-Ubuntu SMP Tue Dec 12 11:46:16 UTC 2017
-
Add the repository:
sudo add-apt-repository ppa:ubilinux/up
-
Update the repository list:
sudo apt update
-
Remove the generic installed kernel:
sudo apt-get autoremove -purge ‘linux-.*generic’
-
Install the kernel for Up Squared:
sudo apt-get install linux-image-generic-hwe-16.04-upboard
-
Reboot:
sudo reboot
-
Verify that the kernel was installed:
uname -srv
Install MRAA
Install the MRAA package to manage IO communications:
sudo npm install -g mraa
Read Light Sensor
To interface with the Grove shield, add this line into your code:
mraa.addSubplatform(mraa.GROVEPI, "0 ")
Using GROVEPI will shift all the pin numbers by 512, therefore, pin A1 for the light sensor will be pin 512 + 1:
var OFFSET = 512
var light = new groveSensor.GroveLight(OFFSET + 1);
Read the light sensor value from the analog pin:
var groveSensor = require('jsupm_grove');
var light = new groveSensor.GroveLight(OFFSET + 1);
var lightValue = light.value();
If the light sensor indicates it is getting dark, this will turn on the LED and send an SMS message:
if(light.value() < 10) {
ledPin.write(1);
…
}
And finally, use the following code to read and write to digital pin D5 to turn on the LED:
var ledPin = new mraa
ledPin.dir(mraa.DIR_OUT);
ledPin.write(1);
Send an Outbound SMS Message
To send a SMS message, we make a request to Twilio and specify the “to”, “from”, and “body”. The “to” is your mobile phone number, the “from” is the SMS-enabled Twilio phone number, and the “body” is the SMS message being sent. See the Twilio API documentation for Twilio client error codes.
var ACCOUNT_SID[] = "ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
var ACCOUNT_TOKEN[] = " xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ";
var twilio_number = "1480405xxxx";
var your_number = "1480xxxxxx";
var twilio = require('twilio');
var client = new twilio(ACCOUNT_SID, ACCOUNT_TOKEN);
message = client.messages.create({from: TWILIO_NUMBER,
to: YOUR_NUMBER,
body: "Hello World!!!"},
function(error, message) {
if (!error) {
console.log("The request was successful.");
console.log(message.Sid);
} else {
console.log("The request was failed. Error code:");
console.log(message.ErrorCode);
console.log(message.sid);
}
});
Example Sketch
Following is an example of sending an SMS message to your cell phone when the light sensor indicates it’s getting dark.
Code Example: send an outbound SMS message example (send_sms_msg.js)
var ACCOUNT_SID[] = "ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
var ACCOUNT_TOKEN[] = " xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ";
var twilio_number = "1480405xxxx";
var your_number = "1480xxxxxx";
var twilio = require('twilio');
var client = new twilio(ACCOUNT_SID, ACCOUNT_TOKEN);
var mraa = require('mraa');
mraa.addSubplatform(mraa.GROVEPI, "0");
console.log("MRAA version: " + mraa.getVersion());
var OFFSET = 512
var ledPin = new mraa.Gpio(OFFSET + 5);
var ledState = true;
ledPin.dir(mraa.DIR_OUT);
var groveSensor = require('jsupm_grove');
var light = new groveSensor.GroveLight(OFFSET + 1);
var sleep = require('sleep');
activity();
function activity() {
console.log("Enter activity()");
console.log("ledState: " + ledState);
var lightValue = light.value();
if(light.value() < 10) {
ledPin.write(1);
message = client.messages.create({from: twilio_number,
to: your_number,
body: "Hello World!!!"},
function(error, message) {
if(!error) {
console.log(message.Sid);
console.log(message.ErrorCode);
} else {
console.log("Error!");
}
});
}
ledState = !ledState;
sleep.sleep(1);
ledPin.write(0);
}
Run the Node Example
Export the MRAA dependent modules:
export NODE_PATH=/usr/local/lib/node_modules
Note that you need to be root user to access the GPIOs, so be sure to include “sudo”. To run the example, type the following command in a terminal:
sudo -E node send_sms_msg.js
When the light sensor indicates it’s getting dark, the LED will be blink and the SMS message will be sent. If your phone receives the message below, the SMS message was successfully sent.
Sent from your Twilio trial account - Hello World!!!
Summary
In this article, we have experimented with sending SMS messages using a Twilio account when the light sensor indicates dark conditions on the UP Squared board on Ubuntu. This experiment is designed to work on any IoT device that supports Intel microcontrollers running on Ubuntu such as Intel® IoT Gateway GB-BXTB-3825. Now try creating your own experiments with different sensors on the UP Squared board and other Intel® IoT devices.
UP Squared
References
About the Author
Nancy Le is a software engineer at Intel Corporation working to enable scalable IoT projects.