Introduction
In most of the applications, we need authentication whether it is web based or mobile/window based. So AAD can be a good solution for authentication for your applications. It provides identity as a service with support of protocols such as OAuth, OpenID, and SAML. Before I start the implementation of our example, please read the basic introduction of these. If you are already aware about these, you can skip this part.
- AAD
- Azure B2C
- ADAL and its libraries: See its targeting v1.0
- MSAL and its libraries: it’s targeting v2.0
- ADAL vs MSAL / v1.0 vs v2.0
1. Configure Azure B2C
- Follow the steps mentioned in this link. You can follow the given easy steps and create all policies for registration/login/reset password, etc.
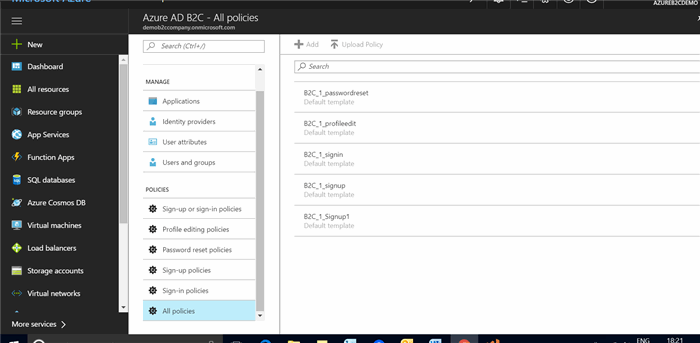
- Register your application: Use reply URL as http://localhost:4200
2. Setting Up the Application
- Download and install Node.js on your system.
- Download Visual Studio Code IDE.
- Check if npm command on command prompt is working on your system, if not, set the path of npm.
- Install CLI.
Angular CLI is an awesome command line tool through which we can do all tasks required for creating, building and deploying an Angular project.
npm install -g @angular/cli
If you have already installed CLI, then:
npm uninstall -g angular-cli
npm cache clean
npm install -g @angular/cli@latest
- Creating the Project using CLI and run the sample application using
ng serve
command.
ng new msalb2c-demo
cd msalb2c-demo
npm i
ng serve -o
ng serve -o
will open your default browser with http://localhost:4200
to view your application in your browser. Various CLI commands are available to ease project development. CLI commands
3. MSAL Implementation
As we are working on Angular2 project, we need JS library that is available on github. So install this npm package in your project.
npm install msal
Here, I am supposing your requirement is to authenticate your application on root level. So when a user hits your application:
- It redirects it to AAD B2C authentication page.
- From authentication page, user can register himself, reset his password and login.
- If user logged-in successfully, it will be redirected to your application URL that you had given in
reply URL
on Azure portal at the time of application registration as seen in the below screen.

- On the application redirection (after authentication), MSAL library stored the required parameters into
sessionstorage
that you can get using window.sessionStorage.getItem('msal.idtoken')
.
MSAL library stores token and other parameters in sessionstorage
by default. You can change this storage location sessionstorage
to localstorage
too if required.
The token is saved by name msal.idtoken
in sessionstorage
after successfully logged in. This token contains all the application claims defined in Sign-in Sign-up policy at Azure like the below image:

If you want to see this token content, then you can use JWT analyzer chrome extension.

Generally, this token is used for API authentication for data operations in client app. So you can authenticate your web API by sending it with request as Authorization header. Your API will validate this token and perform the operation accordingly.
You can get the demo code from my git repository. The brief of code is below:
login.component.ts: This component that will call login method.
ngOnInit() {
const token: string = this.authSandbox.getToken();
if (token === null || token === undefined || token === 'null') {
this.authSandbox.login();
}
auth.service.ts: This is the wrapper class over msal.service.ts
to provide all login, logout code that will be called in your component.
Note: Token has the expiration time so it may expire so we have to check whether the token is active or not. If not, it should acquire a new token by calling login method again.
import { Injectable } from '@angular/core';
import { tokenNotExpired } from 'angular2-jwt';
import { MSALService } from './msal.service';
import { HttpRequest } from '@angular/common/http';
@Injectable()
export class AuthService {
cachedRequests: Array<httprequest<any>> = [];
constructor(private msalService: MSALService) { }
public getToken(): string {
let token = window.sessionStorage.getItem('msal.idtoken');
if (this.isTokenExpired(token)) {
return token;
} else {
this.msalService.login();
}
}
public login() {
const token = this.getToken();
if (token == null || token === undefined || token === 'null') {
this.msalService.login();
}
}
private isTokenExpired(token: string): boolean {
if (token != null && token !== undefined && token !== 'null') {
return tokenNotExpired(null, token);
}
return true;
}
}
</httprequest<any>
msal.service.ts: It has the code that will call MSAL library API.
authentication.guard.ts: It is used to enable authentication at root level.
app-routing.module.ts: It is used to configure your route, it will go to authentication.gurad.ts and if already not authenticated, then redirected to LoginComponent
, canActivate: [AuthenticationGuard]
is guard deciding if a route can be activated.
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full', canActivate: [AuthenticationGuard] },
{ path: 'home', component: AppComponent, canActivate: [AuthenticationGuard] },
{ path: 'authentication', component: LoginComponent }
];
Using the Code
I have created a separate module for authentication in that you have to change your azure AAD B2C configuration in msal.service.ts
file.
private applicationConfig: any = {
clientID: 'paste your application id here',
authority: 'https://login.microsoftonline.com/tfp/demob2c.onmicrosoft.com/B2C_1_SignInPolicy',
b2cScopes: ['https://demob2c.onmicrosoft.com/user.read'],
redirectUrl: 'http://localhost:4200',
extraQueryParameter: 'p=B2C_1_SignInPolicy&scope=openid&nux=1'
};
demob2c
is Azure B2C tenant.
redirectUrl
should be the same as reply URL given at the time of Application registration.
To run the demo code:
- Download the code or clone git repository.
- If angular2 environment is not setup on your system, follow step above 2 "Setting up the application".
- Run command "
npm install
" on vs code terminal. - Run "
ng serve -o"
in vs code terminal.
Points of Interest
- MSAL can be considered as the next version of ADAL as many of the primitives remain the same (
AcquireTokenAsync
, AcquireTokenSilentAsync
, AuthenticationResults
, etc.) and the goal, making it easy to access API without becoming a protocol expert, remains the same. ADAL only works with work and school accounts via Azure AD and ADFS, MSAL works with work and school accounts, MSAs, Azure AD B2C and ASP.NET Core Identity, and eventually (in a future release) with ADFS… all in a single, consistent object model. So if you are using ADAL, plan to switch to MSAL. :) - Azure B2C is awesome. If you see the demo application at https://msalb2c-demo.azurewebsites.net, I haven't written code for login page, sign in page, signup page and other user profile related stuff, all are done by just setting up policies on Azure B2C. It provides a huge bunch of cool features from identity as a service to social login support. The login page with signin, signup and forget features look like below, you can also customize this page based on requirements.

The same azure b2c setup can be used with multiple applications.
History
Will be created soon... :)