Get access to the new Intel® IoT Developer Kit, a complete hardware and software solution that allows developers to create exciting new solutions with the Intel® Galileo and Intel® Edison boards. Visit the Intel® Developer Zone for IoT.
This tutorial shows you how to create the hardware equivalent of “Hello World”: a blinking LED. This is a simple exercise to get you started using the Intel® Quartus® software for FPGA development. You’ll learn to compile Verilog code, make pin assignments, and then program the FPGA to blink one of the eight green user LEDs on the board. You'll use a 50 MHz clock input (there’s an oscillator on board that drives the counter in the FPGA) to create a counter and assign an on-board LED to the nth bit of the counter.
Level: beginner
Materials
Hardware
Terasic DE10-Nano kit
The Terasic DE10-Nano development board, based on an Intel® SoC FPGA, provides a reconfigurable hardware design platform for makers, IoT developers and educators. You can buy the kit here.
Software
Intel® Quartus® Prime Software Suite Lite Edition
The FPGA design software used here is ideal for beginners as it’s free to download and no license file is required. You can download the software here.
Note: The installation files are huge (several gigabytes). If you’re tight on disk space, we recommend you download only those items necessary for this exercise. When prompted which files to download. Select the individual files tab and check Quartus Prime and Cyclone V device support.
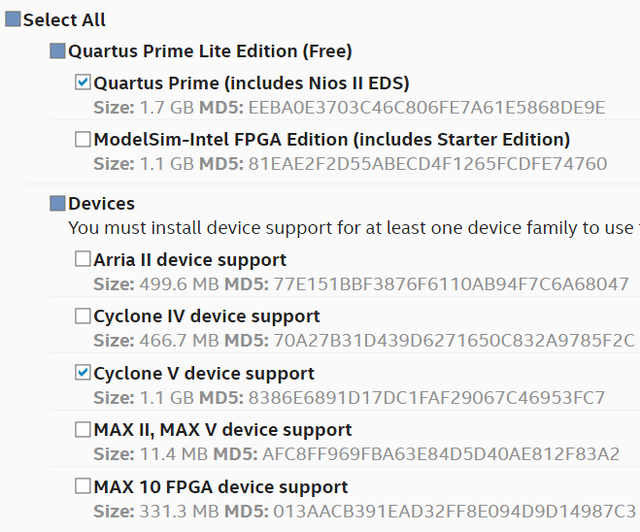
If you’ve downloaded and installed the Intel® Quartus® software, let’s get started creating a project!
Note: Screenshots are based on the latest release v16.1. User experience may vary when using earlier or later versions of Intel® Quartus® software.
Step 1: Create an Intel® Quartus® Software Project
Step 1.a: Open Intel® Quartus® Prime Software Suite Lite Edition.

Step 1.b: Open a New Project Wizard

Step 1.c: Select Next

Step 1.d: Directory, Name, Top-Level Entity
Choose a directory to put your project under. Here, we name our project “Blink” and place it under the intelFPGA_lite folder but you can place it wherever you want. Select Next.

When prompted to create the directory, choose Yes.

Step 1.e: Project Type
Select Empty Project, and then click Next.

Step 1.f: Add Files
You won’t be adding any files here. Click Next.

Step 1.g: Family, Device, and Board Settings
Select the following:
Family: Cyclone V
Device: Cyclone V SE Base
Device name: 5CSEBA6U23I7

Click Next.
Step 1.h: EDA Tool Settings
Skip this part. Click Next.

Step 1.i: Summary
Click Finish.

The following screen displays.

Step 2: Create an HDL File
Hardware Description Language (HDL)
We use Verilog as the HDL. If you are familiar with the C programming language but new to programming in an HDL, Verilog is like C in that you place a semicolon ‘;’ at the end of each statement.
Step 2.a: Navigate to the File tab (main window), and then select New.

Select Verilog HDL File, and then click OK.
Step 2.b: Choose File > Save As. Choose “blink” for the file name. This is your top-level file name and it must match the name of the project name (blink). Click Save.

Step 3: Create a Verilog Module
Step 3.a: Copy and paste this Verilog code into the blink.v window, and then save the file.
module blink(clk, LED);
input clk; output LED;
reg [31:0] cnt; initial cnt <= 0; always @(posedge clk) cnt <= cnt+1;
assign LED = cnt[24]; endmodule

Step 3.b: Analysis & Synthesis
Run the Analysis & Synthesis (right click, and then click Start) to perform a syntax check on the Verilog code.

If the process completes successfully, a green check mark displays next to Analysis & Synthesis. If you get an error, check your syntax and make sure it matches exactly the code block provided above. Remember that Verilog is like C in that a semicolon is used to end a statement. Make sure semicolons are placed appropriately.

Step 4: Choose Pin Assignments
Step 4.a: In the top navigation bar, select Assignments > Pin Planner.

Notice that the input (clk) and output (LED) are listed under Node Name. This is because you ran the Analysis & Synthesis.
Step 4.b: Table 3-8 of the Terasic DE10-Nano User Manual (page 26) shows the LED pin assignments. Choose one of the 8 green user LEDs. Table 3-5 shows the pin assignments for clock inputs. In this tutorial, you use a 50 MHz clock input from the FPGA.
Type the pin numbers into the Location column. The rest of the columns will auto populate with data (some with default values that we’ll need to set). The columns you will need to change are the I/O standard, Current Strength and Slew Rate. For I/O standard, the default is 2.5 volts. Change to 3.3-V LVTTL. The Current Strength will by default read 16ma(default) and we need to select 16ma. If we don’t specifically set them, then we get warning messages in our compilation. Also, change Slew Rate from 1(default) to 1. You don’t need to set a Slew Rate for the clk. Leave that blank.
Node Name | Location | I/O Standard | Current Strength | Slew Rate |
---|
LED | PIN_W15 | 3.3-V LVTTL | 16ma | 1 |
clk | PIN_V11 | 3.3-V LVTTL | 16ma | |
Set pin Location.

Set Current Strength to 16ma for both input (clk) and output (LED). Set Slew Rate to 1 (only for the LED).

Close out the Pin Planner.
Step 5: Create an SDC File
Before you compile the Verilog code, you need to provide timing constraints for the design. You'll create an SDC (Synopsis Design Constraints) file that contains commands to let the Intel® Quartus® software know how to close timing on the design. Without it, you will get warning messages in the compile flow because the Intel® Quartus® software has no idea how to close timing on the design.
To create a blink.sdc and add that to the blink file directory, do the following.
Open a text editor (notepad) and copy and paste the following content:
# this is a comment
# inform quartus that the clk port brings a 50MHz clock into our design so
# that timing closure on our design can be analyzed
create_clock -name clk -period "50MHz" [get_ports clk]
# inform quartus that the LED output port has no critical timing requirements
# its a single output port driving an LED, there are no timing relationships
# that are critical for this
set_false_path -from * -to [get_ports LED]
Now save as a .sdc file. Place in the blink file directory – same directory where our verilog file (.v file extension) lives.

Step 6: Compile the Verilog Code
Step 6.a:
Right click on Compile, and then click Start. Because there are only have a handful of code lines, the compilation should take about a minute.
Note: To compile the code you don’t need the Terasic DE10-Nano board to be connected to your computer just yet. That’s coming up soon! (Hint: get out the mini-b USB cable that came in the box).


After you compile the Verilog code, you can program the FPGA.
Step 7: Program the FPGA
The final step is to program the FPGA. To do that, you need to connect the board to your computer via the USB blaster port.
Note: Remove the microSD card before programming the FPGA.
Step 7.a: Use the USB cable (mini-b connector) that came with the Terasic DE10-Nano kit. Insert the mini-b connector into the USB Blaster port on the Terasic DE10-Nano board and the Type-A end into a standard USB port on your host computer.
Step 7.b: Turn on the board.
Step 7.c: Right click to open Program Device.

Step 7.d: Hardware Setup
Select Hardware Setup.
Set the Mode to JTAG.

Under the drop down for Currently selected hardware, choose DE-SoC.

Click Close.

Step 7.e: Click Auto Detect.

Select the device 5CSEBA6. This is the FPGA device.

Step 7.f: Add the .sof file.
Right click on the file column for the FPGA device and click Change File.


Step 7.g: Navigate to the output_files folder, select blink.sof, and then click Open.

Here, blink.sof is your programming file. SRAM Object Files (.sof files) are binary files containing data for configuring SRAM-based devices—our FPGA is based on SRAM—using the Intel® Quartus® software Program Device (also called Programmer). The programmer looks into the SOF file and gets the programming bit stream for the device.

Step 7.h: Check the Program/Configure column, and then click Start.

Step 8: Observe the blinking LED
If your progress bar is 100% (Successful), watch the board for the blinking LED.

Next Steps
Now that you’ve got one successful blinking LED under your belt, you can modify the blink rate of the LED by using a different bit of the counter. 1 Hertz is 1/second (cycles per second) and blinking at 1 Hz means the LED blinks once per second. For 2 Hz, the LED blinks twice per second. And 0.25 Hz would blink the LED once every 4 seconds (slow blink). For a slower blink, use a higher bit of the counter and for faster, use a smaller bit (e.g., cnt[22]). Test out different counter bits and see what you get.
Find below some quick clock and counter math to help you make sense of the blinks per second as it relates to the clock and counter bit selected.
Clock and Counter Math
cnt[n] where n = the counter bit
2^n; we've chosen n = 24 in our Verilog code sample
2^24 = 16,777,216
Our clock is 50 MHZ or 50,000,000
Clock / 2^n = blinks per second<br /> 50,000,000 / 16,777,216 = 2.9802
That’s about 3 blinks per second.
Additional Resources
You may know us for our processors. But we do so much more. Intel invents at the boundaries of technology to make amazing experiences possible for business and society, and for every person on Earth.
Harnessing the capability of the cloud, the ubiquity of the Internet of Things, the latest advances in memory and programmable solutions, and the promise of always-on 5G connectivity, Intel is disrupting industries and solving global challenges. Leading on policy, diversity, inclusion, education and sustainability, we create value for our stockholders, customers and society.