.NET Assemblies can contain various types of resources like images, icons, files, etc. Such resources are mostly static, i.e.; do not keep changing during run time or application wise. Also, such resources are not executable items. So while deploying such assemblies, we need to make sure those resources are intact with the packaged assemblies. Else, assemblies may blow up while executing the resource dependent methods. So as part of deployment strategy, we should embed such resources into the assembly itself.
Today, we will see one such example of embedding XML file into assembly.
- Add one Class Library type project into the solution.
- Add one XML file as well. Fill XML file with few data that the class library may use to query.
- Right click on the XML file, and select Properties –> Build Action. Out of several Build Action options, select Embedded Resource.
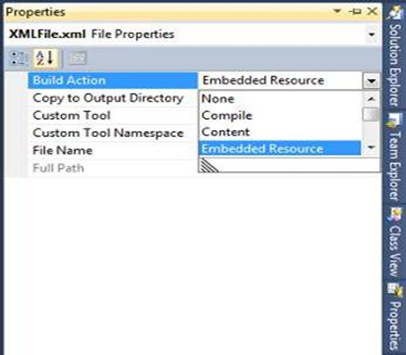
- There you may see other properties as well like Copy to Output Directory. It has options like this:

- If you select Do Not Copy, output of building the class library inside \bin\ folder will not have separate *.dll than embedded XML file.
- If you select Copy Always, output of building the class library inside \bin\ folder will always have *.dll and an embedded XML file.
However, I prefer the first option. By this, we make sure our distributable assembly is only one *.dll. This way, the embedded resource XML file cannot be modified, and our assembly can safely execute methods that depend upon this XML file. I like to suggest one more tip here - rename this XML file extension to *.config. Renaming XML file extension to *.config makes file not browsable by Browser in web applications.
Here, I have shown steps of embedding resource file into .NET assembly. This assembly can be either Class Library or Web application. We cannot embed resource file into web site type project as Web site does not produce assembly like Web application.
Now, let’s see how we can access embedded XML file from assembly during runtime.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Reflection;
using System.Xml;
using System.IO;
namespace MathLibrary
{
public class MathLibrary
{
private const string strFileName = "XMLFile.config";
public System.Xml.XmlDocument GetXMLDocument()
{
var assembly = Assembly.GetExecutingAssembly();
var stream = assembly.GetManifestResourceStreamthis.GetType(), strFileName);
var doc = new XmlDocument();
try
{
if (stream == null)
{
throw new FileNotFoundException("Couldnot find embedded mappings resource file.", strFileName);
}
doc.Load(stream);
}
catch (Exception ex)
{
throw ex;
}
return doc;
}
public System.IO.Stream GetXMLStream()
{
var assembly = Assembly.GetExecutingAssembly();
var stream = assembly.GetManifestResourceStream(this.GetType(), strFileName);
try
{
if (stream == null)
{
throw new FileNotFoundException("Couldnot find embedded mappings resource file.", strFileName);
}
}
catch (Exception ex)
{
throw ex;
}
return stream;
}
}
}
There are two methods shown above - one returning XmlDocument
object and other returning IO.Stream
. Either of the return types can be used to construct XmlDocument
object now at caller end of these methods.
Now let’s verify the assembly if it has any embedded resource or not. We can do this using either Reflector or Ildasm tool.
Reflector View

Ildasm View

See resource name is qualified with assembly namespace name prefixed to it - MathLibrary.XMLFile.config.
Happy coding!