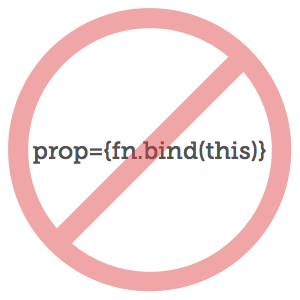
A couple weeks ago, I wrote a post that gave an overview of why calling .bind
inside the render
function is a bad idea, and showed a few better ways to do it (along with some of the bad ways).
This, here, is a TL;DR version of that post. If you want background on why calling .bind
inside render
is bad, and some of the common (bad) ways to do it, check out the original post.
Using React.createClass? No Need to Call Bind.
If you’re using React.createClass
, the member functions in your component are automatically bound to the component instance. You can freely pass them around without calling bind
. You’re all set.
Best, Easiest Way: Class Instance Field, Arrow Function
This creates and binds the function all at once. Easy. Inside render
(and elsewhere), the function is already bound because the arrow function preserves the this
binding.
The only downside: it’s not officially part of the standard yet. While it is supported by Babel, there’s the small risk that this feature could be taken out of the spec. However, many people are already using it, so it seems likely to stay.
class Button extends React.Component {
handleClick = () => {
console.log('clickity');
}
render() {
return (
<button onClick={this.handleClick}/>
);
}
}
Don’t like this way? Read the original post for other alternatives including binding in the constructor and using decorators.
Binding to Pass Arguments
Sometimes, you need to bind a function to its arguments, like if you’re rendering a list of items and you want to pass the item’s index to the onClick
handler.
You can avoid the bind
by using the method described in the original post.
Moving .bind
outside of render is a performance optimization that will only help if you’ve implemented shouldComponentUpdate
(or you’re using the Pure Render mixin, or the new PureComponent
base class).
The slowness is not caused by creating new functions each time (that’s pretty quick). Rather, creating a new function means that the prop’s value is different every time, and React will re-render that child component unnecessarily.
Best Alternative to Binding in Render was originally published by Dave Ceddia at Angularity on August 10, 2016.
CodeProject