Introduction
Getting a simple Angular 2 application up and running is fairly straight forward when following the 5 minute quickstart tutorial. To implement the same application in an ASP.NET 5 environment adds a lot of complexity not explained in the tutorial and therefore the purpose of this article is to explain the processes involved in setting up a basic Angular 2 application within an ASP.NET 5 environment.
IDE and Other Development Tool Versions
The versions of the IDE and other development tools used when writing this article is listed below:
- Visual Studio 2015 14.0.24720.00 Update 1
- Resharper Ultimate 2016.1.2
- TypeScript 1.8.6
- nodejs 4.4.5
Very Important
- It is of critical importance to update to the latest version of ReSharper, JetBrains fixed some bugs related to the TyeScript inspection settings.
- Visual Studio 2015 ships with an older version of Node.js, make sure that you update to the latest available release.
Setting Up An Empty ASP.NET 5 Web Project
From the File menu, select New > Project which will bring up the New Project dialog.
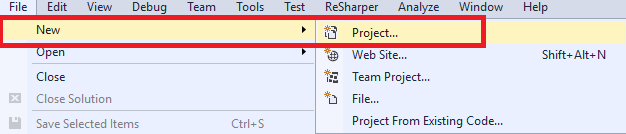
From the New Project dialog, select the ASP.NET Web Application template and name it Angular2QuickStart
.

Click the Ok button and select the Empty ASP.NET 5 template from the ASP.NET Project dialog.

Click the Ok button which will create the empty ASP.NET 5 project for you.
Configure the ASP.NET Application to Serve Static Files
Open the Startup.cs file and remove the following code from the Configure
method:
app.Run(async (context) =>
{
await context.Response.WriteAsync("Hello World!");
});
Now add the following to the Configure
method to enable the application to server default and static files such as index.html.
app.UseDefaultFiles();
app.UseStaticFiles();
The Configure
method should now look like this:
public void Configure(IApplicationBuilder app)
{
app.UseIISPlatformHandler();
app.UseDefaultFiles();
app.UseStaticFiles();
}
Create the Index.html File
Right click on the wwwroot folder in Solution Explorer and select Add > New Item ... which will bring up the Add New Item dialog.

Select the Text File template and name it Index.html.

Click on the Add button which will create the Index.html file and add the following HTML code to the file.

Update the project.json File
At the time of writing, the ASP.NET 5 application would end abrupty when running the project, this is because of the version used for the Microsoft.AspNet.StaticFiles
dependency in the project.json file. To fix this problem, make the following changes to the project.json file:
- Remove
"dnxcore50": { }
(The core framework is not used for this application) - Update the version of the
Microsoft.AspNet.StaticFiles
dependency to 1.0.0-rc1-final
The project.json should now look like this:
{
"version": "1.0.0-*",
"compilationOptions": {
"emitEntryPoint": true
},
"dependencies": {
"Microsoft.AspNet.IISPlatformHandler": "1.0.0-rc1-final",
"Microsoft.AspNet.Server.Kestrel": "1.0.0-rc1-final",
"Microsoft.AspNet.StaticFiles": "1.0.0-rc1-final"
},
"commands": {
"web": "Microsoft.AspNet.Server.Kestrel"
},
"frameworks": {
"dnx451": { }
},
"exclude": [
"wwwroot",
"node_modules"
],
"publishExclude": [
"**.user",
"**.vspscc"
]
}
Save the file which should in turn update the project dependencies. Now run the application by pressing F5.

Create the typings.json File
Up to this point in the article, we did the setups and configurations for a working ASP.NET 5 web application that is serving static files. The next section will focus on the setups and configurations needed to incorporate Angular 2. Create a typings.json file in the project root folder.

Select the Client-side > JSON File template and click on the Add button.

Add the following to typings.json and save the file.
{
"globalDependencies": {
"core-js": "registry:dt/core-js#0.0.0+20160317120654",
"jasmine": "registry:dt/jasmine#2.2.0+20160505161446",
"node": "registry:dt/node#4.0.0+20160509154515"
}
}
Create the package.json File
Create a package.json file in the project root folder. The package.json file is used to install Angular 2 and some other development dependencies. Right click on the project and select Add > New Item... which will bring up the Add New Item dialog.

Select the Client-side > NPM Configuration File template and click on the Add button.

Add the following to package.json and save the file which will in turn install the specified dependencies. The file is similair to the package.json file provided by the 5 minute quickstart tutorial.
Please note: The only difference is the "gulp": "3.9.1" development dependency needed to copy files to the wwwroot folder.
{
"name": "angular2-quickstart",
"version": "1.0.0",
"scripts": {
"start": "tsc &&
concurrently \"npm run tsc:w\" \"npm run lite\" ",
"lite": "lite-server",
"postinstall": "typings install",
"tsc": "tsc",
"tsc:w": "tsc -w",
"typings": "typings"
},
"license": "ISC",
"dependencies": {
"@angular/common": "2.0.0-rc.1",
"@angular/compiler": "2.0.0-rc.1",
"@angular/core": "2.0.0-rc.1",
"@angular/http": "2.0.0-rc.1",
"@angular/platform-browser": "2.0.0-rc.1",
"@angular/platform-browser-dynamic": "2.0.0-rc.1",
"@angular/router": "2.0.0-rc.1",
"@angular/router-deprecated": "2.0.0-rc.1",
"@angular/upgrade": "2.0.0-rc.1",
"systemjs": "0.19.27",
"core-js": "^2.4.0",
"reflect-metadata": "^0.1.3",
"rxjs": "5.0.0-beta.6",
"zone.js": "^0.6.12",
"angular2-in-memory-web-api": "0.0.11",
"bootstrap": "^3.3.6"
},
"devDependencies": {
"gulp": "3.9.1",
"concurrently": "^2.0.0",
"lite-server": "^2.2.0",
"typescript": "^1.8.10",
"typings": "^1.0.4"
}
}
Create the gulpfile.js File
One of the major differences when working with the basic Angular 2 application as explained in the 5 minute quickstart tutorial and an Angular 2 application within an ASP.NET 5 environment is that the files in the node_modules folder is not accessible from an ASP.NET 5 application. We therefore have to copy the files that we need from the node_modules folder to the wwwroot folder. We will do this using gulp.
Right click on the project and select Add > New Item... which will bring up the Add New Item dialog.

Select the Client-side > Gulp Configuration File template and click on the Add button.

Add the following to gulpfile.js to create the tasks that will copy the Angular 2 dependencies to the wwwroot folder.
var gulp = require('gulp');
var rimraf = require('rimraf');
var paths = {
npm: './node_modules/',
angular: './wwwroot/lib/@angular/',
angular2InMemoryWebApi: './wwwroot/lib/angular2-in-memory-web-api/',
rxjs: './wwwroot/lib/rxjs/',
lib: './wwwroot/lib/'
};
var angular = [
paths.npm + '@angular/**/*'
];
var angular2InMemoryWebApi = [
paths.npm + 'angular2-in-memory-web-api/**/*'
];
var rxjs = [
paths.npm + 'rxjs/**/*'
];
var libs = [
paths.npm + 'core-js/client/shim.min.js',
paths.npm + 'zone.js/dist/zone.js',
paths.npm + 'reflect-metadata/Reflect.js',
paths.npm + 'systemjs/dist/system.src.js'
];
gulp.task('angular', function() {
return gulp.src(angular).pipe(gulp.dest(paths.angular));
});
gulp.task('angular2InMemoryWebApi', function () {
return gulp.src(angular2InMemoryWebApi).pipe(gulp.dest(paths.angular2InMemoryWebApi));
});
gulp.task('rxjs', function () {
return gulp.src(rxjs).pipe(gulp.dest(paths.rxjs));
});
gulp.task('libs',function() {
return gulp.src(libs).pipe(gulp.dest(paths.lib));
});
gulp.task('clean', function(callback) {
rimraf(paths.lib, callback);
});
Bind the gulp Tasks to Visual Studio Events
To execute the gulp tasks, we have to bind them to Visual Studio events. Right click on gulpfile.js in Solution Explorer and select Task Runner Explorer.

Click on the refresh button in Task Runner Explorer to update the gulp tasks created.

To bind the gulp task to a Visual Studio event, right click [task] > Bindings > [Visual Studio Event]

Bind the gulp tasks to the Visual Studio events as specified below:
- angular -> After Build
- angular2InMemoryWebApi -> After Build
- clean -> Clean
- libs -> After Build
- rxjs -> After Build
Build the project which should now create the lib folder within the wwwroot folder.
Please note: The initial copy might take a while to complete.
The wwwroot folder will look like this after the build.

Creating the Angular 2 TypeScript Files
The purpose of this article is to explain the processes involved in setting up Angular 2 within an ASP.NET 5 environment and therefore I will not explain the content of the TypeScript files we will be creating in this section.
Also take note that you do not have to use TypeScript, it is simply my personal preference. You can also write your Angular 2 applications using the following:
Right click on the project and select Add > New Folder.

Rename the folder to scripts:

Right click the scripts and select Add > New Item... which will bring up the Add New Item dialog.

Add an app.component.ts file by selecting the Client-side > TypeScript File template and clicking on the Add button.

Add the following code to the app.component.ts file:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: Congratulations on your fisrt Angular 2 application in an ASP.NET 5 environment!'
})
export class AppComponent { }
Right click the scripts folder and select Add > New Item... which will bring up the Add New Item dialog.

Add a main.ts file by selecting the Client-side > TypeScript File template and clicking on the Add button.

Add the following code to the main.ts file:
import { bootstrap } from '@angular/platform-browser-dynamic';
import { AppComponent } from './app.component';
bootstrap(AppComponent);
Create the tsconfig.json File
We have to configure the TypeScript compiler options, this is done by creating a tsconfig.json file.
Right click the project and select Add > New Item... which will bring up the Add New Item dialog.

Add a tsconfig.json file by selecting the Client-side > TypeScript JSON Configuration File template and clicking on the Add button.

Add the following to the tsconfig.json file:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"moduleResolution": "node",
"sourceMap": true,
"emitDecoratorMetadata": true,
"experimentalDecorators": true,
"removeComments": false,
"noImplicitAny": false,
"outDir": "wwwroot/app/"
},
"exclude": [
"node_modules",
"wwwroot"
]
}
Build the project which should create the wwwroot/app folder containing main.js and app.component.js.

Create the systemjs.config.js File
This file was adopted from the one provided by the 5 minute quickstart tutorial.
Right click the wwwroot folder and select Add > New Item... which will bring up the Add New Item dialog.

Add a systemjs.config.js file by selecting the Client-side > JavaScript File template and clicking on the Add button.

Add the following to the systemjs.config.js file:
(function (global) {
var map = {
'app': 'app',
'@angular': 'lib/@angular',
'angular2-in-memory-web-api': 'lib/angular2-in-memory-web-api',
'rxjs': 'lib/rxjs'
};
var packages = {
'app': { main: 'main.js', defaultExtension: 'js' },
'rxjs': { defaultExtension: 'js' },
'angular2-in-memory-web-api': { main: 'index.js', defaultExtension: 'js' },
};
var ngPackageNames = [
'common',
'compiler',
'core',
'http',
'platform-browser',
'platform-browser-dynamic',
'router',
'router-deprecated',
'upgrade',
];
function packIndex(pkgName) {
packages['@angular/' + pkgName] = { main: 'index.js', defaultExtension: 'js' };
}
function packUmd(pkgName) {
packages['@angular/' + pkgName] = { main: pkgName + '.umd.js', defaultExtension: 'js' };
};
var setPackageConfig = System.packageWithIndex ? packIndex : packUmd;
ngPackageNames.forEach(setPackageConfig);
var config = {
map: map,
packages: packages
}
System.config(config);
})(this);
Update the Index.html File
Update the Index.html file as depicted in the following image to setup the Angular 2 application.

Build and run the application to see the Angular 2 application in action.

Congratulations! You just created your first Angular 2 application in an ASP.NET 5 environment.

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.