Let's see how we can display the SharePoint list item permissions using REST API.
I am going to use the SharePoint hosted app and SharePoint online. You could use an on premises installation as well.
Let's start by creating a SharePoint hosted app. Update the App.js as follows:
'use strict';
var hostweburl;
var appweburl;
var executor;
$(document).ready(function () {
getCustomPermission();
});
function getCustomPermission() {
$(document).ready(function () {
hostweburl =
decodeURIComponent(
getQueryStringParameter("SPHostUrl")
);
appweburl =
decodeURIComponent(
getQueryStringParameter("SPAppWebUrl")
);
var scriptbase = hostweburl + "/_layouts/15/";
$.getScript(scriptbase + "SP.RequestExecutor.js", getRoleAssignments);
});
}
function getRoleAssignments() {
executor = new SP.RequestExecutor(appweburl);
executor.executeAsync(
{
url:
appweburl +
"/_api/SP.AppContextSite(@target)/web/lists/getbytitle('Test')/
items(1)?$expand=RoleAssignments/Member/Users&@target='" +
hostweburl + "'",
method: "GET",
headers: { "Accept": "application/json; odata=verbose" },
success: successHandler,
error: errorHandler
}
);
}
function successHandler(data) {
var jsonObject = JSON.parse(data.body);
var roleAssignmentHTML = "";
var results = jsonObject.d.RoleAssignments.results;
for (var i = 0; i < results.length; i++) {
roleAssignmentHTML = roleAssignmentHTML +
"<p><b>" + results[i].Member.OwnerTitle +
"</b></p>";
var users = results[i].Member.Users.results;
if (users) {
for (var u = 0; u < users.length; u++) {
roleAssignmentHTML = roleAssignmentHTML +
"<p>" + users[u].Title + "</p>";
}
}
}
document.getElementById("message").innerHTML =
roleAssignmentHTML;
}
function errorHandler(data, errorCode, errorMessage) {
document.getElementById("message").innerText =
"Could not complete cross-domain call: " + errorMessage;
}
function getQueryStringParameter(paramToRetrieve) {
var params =
document.URL.split("?")[1].split("&");
var strParams = "";
for (var i = 0; i < params.length; i = i + 1) {
var singleParam = params[i].split("=");
if (singleParam[0] == paramToRetrieve)
return singleParam[1];
}
}
Notice that we are using the $expand=RoleAssignments/Member/Users
to get the user information present in each assignment.
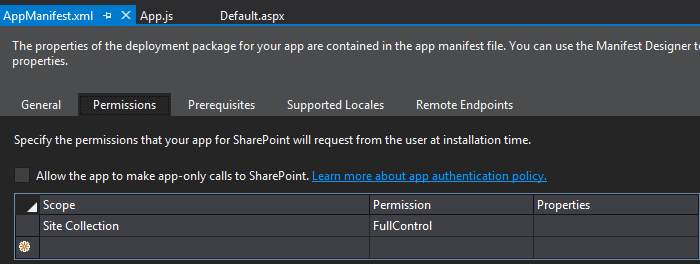
In deploying to production, you need to try out the different permission levels to ensure you are using the minimum required permissions.
This approach requires that we have a high enough permission level because we are listing the information of other users also.
When you build and execute the code, it should list the users and SharePoint groups which have the permission on the item.

I have uploaded the code for this solution in github at ItemLevelPermissions.
The post Display the SharePoint list item permissions using REST API appeared first on The SharePoint Guide.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.