Updated December 22, 2015 — beta 0
Angular 2 is finally in beta! RxJS is no longer included, it must be installed manually.
You’ve already invested time – lots of time learning Angular 1. Controllers, services, directives, filters… All those terms finally make sense – it’s been quite a long road to get here.
And now, along comes Angular 2!
And they’re getting rid of controllers? Everything is a component now? What happened?!
“Looking at Angular 2 is like looking at a different programming language.”
Angular 2 looks all-new: new language, new syntax, new concepts. “This is gonna be like learning Angular 1 all over again, but worse!”
But it doesn’t have to be that way. Today, you will build a “hello world” app in Angular 2, using plain old JavaScript. No ES6, no TypeScript. And it’s gonna take about 5 minutes of your time.
You will laugh when you see how easy this is. Ready to get started?
Install angular2
Open up a Terminal. Create a new directory:
$ mkdir plain-js-ng2
$ cd plain-js-ng2
Install Angular 2:
$ npm install angular2@2.0.0-beta.0 rxjs@5.0.0-beta.0
(npm may complain. Ignore the errors as long as you end up with node_modules/angular2
and node_modules/rxjs
folders)
Note: If you have trouble installing angular2, make sure you're using the official NPM registry. Check the one you're using with npm config get registry
, and if it's not "https://registry.npmjs.org", run npm config set registry https://registry.npmjs.org
.
1. Create app.js
Create a new file called app.js
in your favorite text editor. Type this out:
Type it out by hand? Like a savage?
Typing it drills it into your brain much better than simply copying and pasting it. You're forming new neuron pathways. Those pathways are going to understand Angular 2 one day. Help 'em out.
<code class="language-javascript" data-lang="javascript">(function() {
var HelloApp =
ng.core
.Component({
selector: 'hello-app',
template: '<h1>Hello Angular 2!</h1>'
})
.Class({
constructor: function() {}
});
document.addEventListener('DOMContentLoaded', function() {
ng.platform.browser.bootstrap(HelloApp);
});
})();</code>
2. Create index.html
Create an index.html
file and type this in:
<code class="language-html" data-lang="html"><html>
<head>
<title>Plain JS Angular 2</title>
<script src="node_modules/rxjs/bundles/Rx.umd.js"></script>
<script src="node_modules/angular2/bundles/angular2-polyfills.js"></script>
<script src="node_modules/angular2/bundles/angular2.umd.dev.js"></script>
<script src="app.js"></script>
</head>
<body>
<hello-app></hello-app>
</body>
</html></code>
Type it out by hand? Like a savage?
We went over this already. It's like 292 characters. Type it in.
3. Open index.html
Load up index.html
however you like. I like running open index.html
in a Terminal (on a Mac).
So Easy! Such Angular!
That’s all there is to it. I told you it was easy!
By the way, now would be an excellent time to sign up for future Angular 2 articles! I’ll be going into more detail on Plain-JS Angular 2 as well as TypeScript and ES6, so if you want to stay ahead of the curve, put in your email below!
<form action="//binarynirvana.us5.list-manage.com/subscribe/post?u=caad576c33eb4a330b1dccd47&id=de622be6c5" method="post" id="mc-embedded-subscribe-form" name="mc-embedded-subscribe-form" class="ng-embedded-subscribe-form validate" target="_blank" >
</form>
If you’re curious about those script files being included in index.html
, check out my Angular 2 Dependencies Overview.
Let’s look at what’s going on in this little app.
Components over Directives
Angular 2 gets rid of directives in favor of components, but conceptually they’re very similar. Even their code is not that different. Have a look at this diagram:
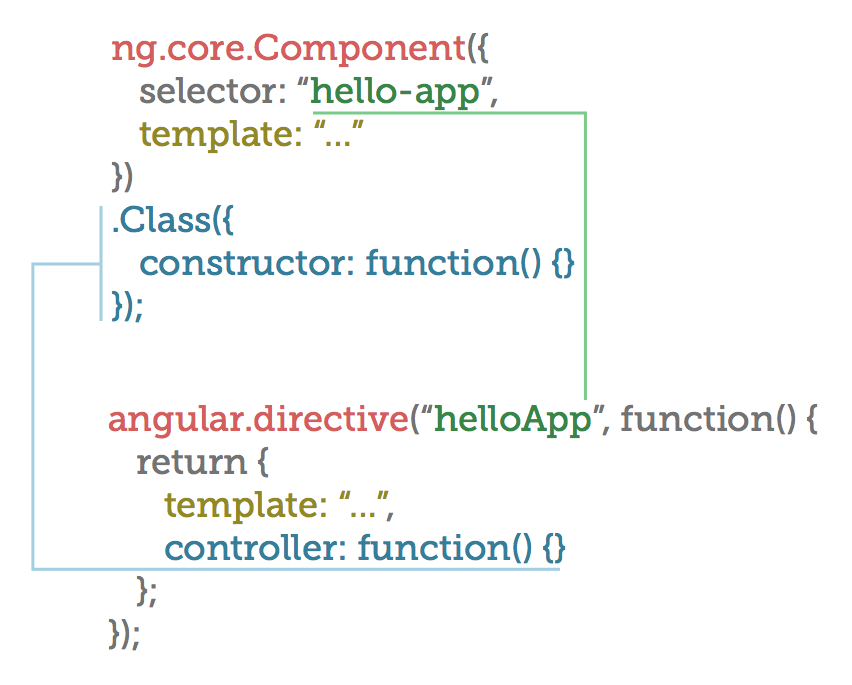
Both specify an HTML tag name (selector
vs inferring it from the directive’s name).
Both have associated behavior (Class
vs controller
).
Both have a template.
Even the chaining syntax isn’t that foreign, coming from Angular 1.
Explicit bootstrap
Angular 2 comes with a function called bootstrap
that initializes the app. This bit of code from above starts everything up:
<code class="language-javascript" data-lang="javascript">document.addEventListener('DOMContentLoaded', function() {
ng.platform.browser.bootstrap(HelloApp);
});</code>
It listens for a DOMContentLoaded
event using plain-old JavaScript, then calls ng.platform.browser.bootstrap
with the root component.
This is a conceptual shift from Angular 1: Apps are composed of nested components all the way down. Everything is nested inside the root.
Your Turn
Now that you have a (super-basic) Angular 2 app running, you can begin to play around with it. Here are some things to try:
Add a second component
Create a new component that contains your name and render it after the h1
tag.
Some hints:
-
Every component needs to be explicitly required in order to use it. The property belongs inside Component and is called directives
(oddly enough). It contains an array of components (which are just Javascript objects). e.g. directives: [MyName]
would require the MyName
component and let you use its tag.
-
Templates don’t appear to be limited to a single root node like with Angular 1 directives.
Add a list of names
Add a ul
tag with a nested li
for each name.
-
You’ll need the new ngFor
syntax. It replaces ng-repeat
, and the attribute looks like *ngFor="#name of names"
(there’s a *
in front, it’s important).
-
Variables declared on the constructor’s this
object will be available from within the template using ngFor
and the familiar {{ curly_braces }}
syntax.
Give Up?
Try to figure out the exercises on your own first. Refer to this example from the official Angular 2 docs - their syntax doesn’t look the same, but I bet you can work it out.
But if you’re truly stuck, the answers are below (hidden). Click to reveal.
<script>
function showAnswer(e) {
$('.click-to-show .highlight').css('display', 'block');
console.log('shown!!', e);
e.preventDefault();
return false;
}
</script>
[answer] Show your name
<code class="language-javascript" data-lang="javascript">var MyName = ng.core
.Component({
selector: 'my-name',
template: '<span>Dave</span>'
})
.Class({
constructor: function() {}
});
var HelloApp =
ng.core.Component({
selector: 'hello-app',
template: '<h1>Hello Angular 2!</h1><my-name></my-name>',
directives: [MyName]
})
.Class({
constructor: function() {}
});</code>
[answer] List of friends
<code class="language-javascript" data-lang="javascript">var FriendsList = ng.core
.Component({
selector: 'friends',
template: '<ul><li *ngFor="#friend of friends">{{ friend }}</li></ul>',
directives: [ng.common.NgFor]
})
.Class({
constructor: function() {
this.friends = ["Alice", "Bob", "James", "Aaron"];
}
});
var HelloApp =
ng.core.Component({
selector: 'hello-app',
template: '<h1>Hello Angular 2!</h1><friends></friends>',
directives: [FriendsList]
})
.Class({
constructor: function() {}
});</code>
Angular 2 in Plain JS was originally published by Dave Ceddia at Angularity on October 20, 2015.
CodeProject