In this article, you will learn about a JavaScript object, FormGen, for creating and managing Forms.
Introduction
This article is about a JavaScript object (fGen ) for creating and managing Forms[1]; fGen is sufficiently generalized for creating a wide set of forms, from simple message box to relatively complex forms with widgets like buttons, graphic buttons, text fields, combos, radio buttons and images; moreover fGen supports event management, local processing and Ajax interaction.
In this article there is only an informal and not exhaustive presentation of the package, however, some particularities will be highlighted when commenting on the examples.
The package has been rebuild, in particular the functionalities are unchanged, apart from some minor implementations, but the syntax has been made more intuitive for to achieve simpler use, better security and greater maintainability.
| 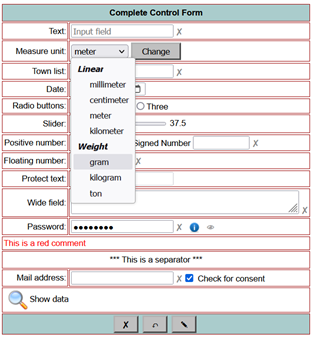 |
Using the Program
The form generator is in formgen.js script, which contains the object function fGen
; this function is used for create a new object:
fGenObject = new fGen(idContainer,control_list)
where idContainer
is the id of an HTML tag that will host the generated form and control_list
is the list of widgets with possibly other information (controls) for managing the form.
If the idContainer
is omitted, it is created with id fg_PopUp
and class fg_PopUp
useful for creates movable forms.
Every widget description is characterized by a list of attributes separated by space(s): Type
, Name
, Label
and Extra
parameters:
Type
is the widget type, this is indifferent to the case. Name
is the widget name, the case is significant. Label
is the label of the widget, if omitted it is generated from the Name
with some syntactic sugar, for example name_Surname
becomes Name surname
. Extra
can contain information for the widget, some depends on the type, but other are generic; these can be in the form key value
or parameter
for example:
- Image img images/condor.gif
width 100
- RDB Sex 'images/sex.png' 'M=♂ Male,F=♀ Female,N=Not specified'
vertical
- T mediaFile ''
File
width 50
Filter audio/*,video/*,image/*
Every widget description is separated by line terminator(s).
Among the widgets there are some types of input texts, lists, buttons, slider, radio button, check box, hidden fields..., the list can also contain some controls with a semantic slightly different:
Form
for telling how to manage the form when it is submitted, in particular it can contains the parameters server URL
and call function
; Defaults
for insert initial value on widgets; Control
for executing some checks on the widgets value before submitting the form; Get
for obtaining data, useful for populating the form, update lists or change widgets value or images (also at predefined intervals). Event
for associate an event to a widget: the event can be handled by a local JavaScript function or by the server, for example by a PHP script. The response can be sent to a JavaScript function or entered directly on a DOM component. Dictionary
for manage language traslation.
fGen
inserts the buttons Ok
, Reset
and Cancel
, whose names are respectively fg_Ok
, fg_Cancel
, and fg_Reset
, depending on the widgets present, for example, if there is only one data to insert (text , combo or radio button) no buttons are presents because the choice or the value inserted exits the form (try it yourself):
Form frm 'Select a measure' server echo.php call receive
CMB Unit 'Measure Unit' =Linear,mm=millimeter,cm=centimeter,m=meter,
km=kilometer,=Weight,g=gram,kg=kilogram,t=ton
Two notes on the above example:
- the
Form
pseudo control tells to call the server script echo.php
whose response will be handled by the JavaScript function receive
, - the CMB widget generates a combo box elements starting from a set of items separated by comma where the item can have the form
key[=exposed value]
;
key
is the value returned from the choice, moreover the items can be grouped using the syntax =group
(in the example =Linear
and =Weight
) .
We can insert custom buttons or graphics buttons or replace the standard buttons captions (try it yourself).
function infoPSW(frm) {
alert("Password from 6 to 12 characters.\nand at least one number and
one capital letter and one letter")
}
var pswForm = `
T psw Password width 15 password hint 'Insert password'
B Info ? width 25 event click call infoPSW after psw
B fg_Cancel ✘ width 30 title 'Cancel form'
B fg_Reset \x21B6 width 30 title 'Reset form'
B fg_Ok ✎ width 30 title Go!
Required psw
Control psw is (?=.*\d)(?=.*[A-Z])(?=.*[a-z]).{6,12} 'Password from 6 to 12 characters.\nand at least one number and one capital letter and one letter'
`
var frm = new fGen("",pswForm)
Note the use of Unicode characters for the button's caption, for example the caption of the Ok button is ✎ (✎).
Form Presentation
The data are presented in the order in which they appear in the control_list
, except for the custom buttons that appear together the standard buttons inserted by fGen
at the bottom of the form; therefore, it is possible to insert custom buttons, and others widgets, at the right or below another widget.
With CSS style sheet, we can control the presentation for the form is displayed using a table
tag which has a class name fg_Table
, the buttons have the class name fg_Button
or fg_Gbutton
. The labels have class name fg_Label
. Some styles are embedded in fGen
:
.fg_Buttons {text-align:center}
.fg_Title img,.fg_Comment img,.fg_Label img,.fg_Button img,.fg_GButton img {
padding-left: 4px;
vertical-align:middle;
}
.fg_Erase {cursor:pointer;color:#888;padding-left: 4px}
.fg_Error {color:red}
.fg_Number {text-align:right}
.fg_Slider {width:3em;padding-left: 4px}
.fg_GButton {border: none;background: none}
.fg_Button:hover, .fg_GButton:hover {cursor:pointer}
.fg_Button:disabled, .fg_GButton:disabled{cursor: not-allowed;
Note that those styles can be overriden by setting !important
to the style, for example for change the color of the erase marker the CSS can be: .fg_Erase {color:blue !important;}
Manage Events
We can enter add the management of events using JavasScript addEventListener
see the fragment below (try by yourself).
...
Fgen = new formGen("fg")
$("Agree").addEventListener("click",function()
{$('Start').disabled = !this.checked;},true);
$('Start').disabled = true;
...
var agreeForm = `
Form '' 'Event example Form' server echo.php call receive
CKB Agree 'Consent cookies?' 'I agree'
B Start '' disabled
B fg_Cancel ✘
`
var frm = new fGen("",agreeForm)
Note the Start
button as alternative to the Ok
button, that it is initially disabled .
However, the simplest way to handle events is via the control Event or by the parameter Event of a widget, in both case the syntax is similar:
Event eventType [on fieldName] [Server URL] [call function|set field]
When is present Server URL the JavaScript function will process the response or the data is inserted into the field indicated by set , see the example below (try it yourself).
Form fHBook 'Static Form' server echo.php call receive static
B Clock images/clock.png inline 'Get Time'
T Text ' ' disabled after Clock
B xExcel images/excel.png Event click alert 'Create Excel file' inline 'Excel file'
B ShowImage images/faro.ico inline 'Show image'
I Img '' height 200 comment
B ShowCite images/new.png inline 'Show IT quote'
Event click on ShowImage server getImage.php?NoTitle set Img
Event click on Clock server getSample.php?Type=Time set Text
Event click on ShowCite server getITCite.php set result2
Note that the parameter set of the control Event refers to the widget via the widget name, while in the last control Event the reference is to an ID external to the form
|  |
When the Form is Submitted
Data controls
The data are controlled as indicated by the pseudo type Control
or Check
, in case of errors the form is not submitted and the fields in error are bordered in red and it is generated an alert.
The controls that the pseudo type Check
allows to perform:
- compare values with constants or with other fields,
- formally correct mail address,
- not empty field,
- match a regular expression,
- control by a custom function
In the example below controls on a numeric floating field (try it yourself).
Form '' 'Example of control on data' server echo.php call receive
T Number '' Float 'Insert Floating number'
Check Number >= -200 'Not allowed lesser -200'
Check Number <= 200 'Not allowed greater 200'
Check Number is ^[-+]?\d{1,3}(\.\d{1,2})?$ 'incorrect format'
C c1 'Floating point number\nbetween -200.00 and 200.00 (two decimals)'
Data submission
The submission depends on parameters URL
and function
of pseudo type Form
:
- only
URL
: the response generates a new page. URL
and function
: function
receives the answer from the server, via a built-in Ajax module. - Only
function
: the function
is called with form as argument, obviously it needs a local elaboration. - If no
URL
nor function
fGen
shows the data replacing the form.
Advanced Use
Compile the form with data from server
The pseudo type Get
can be used to retrieve data from INTERNET via the internal Ajax function for populate (or change) values on Combo box or Lists or to populate the form with default values, for example with data from database.
Get
needs a URI
, i.e. a script on an Internet site that provides the data that can be a value to assign to a widget or the items for populate a combo boxes or, in case of defaults is expected a set of values acceptable by control Defaults
(try it yourself):
Form frmg2 'Get example' server echo.php call receive
T Widget '' disabled
CMB WidgetType '' '' link Widget group
CMB Hellas 'Greek letters' '' multiple
List Town
CMB Languages
Hidden HiddenField
Get * getSample.php?Type=Defaults
Get WidgetType getSample.php?Type=Type
Get Town getSample.php?Type=Towns
Get Hellas getSample.php?Type=Hellas
GET Languages getSample.php?Type=Lang
Here is the PHP script that handles the requests:
<?PHP
$type = $_REQUEST["Type"];
if ($type == "Type") {
echo "=Buttons,B=Button,R=Radio button,RV=Vertical Radio button,"
."=Lists,CMB=Combo box,L=List,"
."=Texts,C=Comment,F=File,H=Hidden field,N=Numeric,NS=Numeric signed,"
."NF=Numeric with decimals,P=Password,T=Text,U=Unmodifiable text,"
."=Others,CKB=Check box,S=Slider";
}
if ($type == "Hellas") {echo "Alfa,Beta,Delta,Gamma,Epsilon";}
else if ($type == "Towns") {echo "London,Paris,Rome,Toulon,Toulouse,Turin,Zurich";}
else if ($type == "Defaults") {echo "Town=Turin Hellas=Alfa 'WidgetType=T file' Languages=Pascal 'HiddenField=El Condor'";}
else if ($type == "Lang") {echo "Algol,Cobol,Fortran,JavaScript,Pascal,PHP";}
?>
Furthermore, the pseudo-type GET can have a timeout parameter to allow periodic updating of comments, texts or images (try it yourself):
Form frm '' server echo.php call receive
C Time Clock
T wField 'IT Cite' width 250 disabled
Image Image '' '' height 200 comment
GET Time getSample.php?Type=Time every 60000
GET wField getITCite.php?CR every 9000
Get Image getImage.php every 11000
B fg_Cancel ✘ width 45
|  |
Movable Forms
In the SandBox, there is an example of movable form (and internationalization).
This is achieved through a form generated without indicating the creation tag , so fGen
generates a tag with class fg_PopUp
; the form must have the form
control in order to be generate a title row which reacts to the move event and where the cursor style is set to move
.
At this point the user must include some JavaScript for move the form (the SandBox
includes the script moveItem.js) (try it yourself).
Widget
List |
Form rdb 'Radio buttons example' server echo.php call receive
R Status '' M=Married,S=Single,W=Widow
R Sex 'images/sex.png' 'M=♂ Male,F=♀ Female,N=Not specified'
R Output '' E=images/excel.png,None
R Nations '' 'It=images/its.png Italia,Fr=images/frs.png France,Es=images/ess.png España,Us=images/uss.png United States,El=images/els.png Ελλάδα' vertical
Defaults Nations=El Sex=M
|
JS |
function movableForm(widgetList) {
if($("fg_PopUp")) $("fg_PopUp").remove();
Fgen = new formGen("",widgetList)
var link = $("fg_PopUp")
link.style.top = 0.5 * (window.innerHeight - link.offsetHeight);
link.style.left = 0.5 * (window.innerWidth - link.offsetWidth);
$("ftfg_Title").classList.add("fg_Movable")
$("ftfg_Title").addEventListener("mousedown", dragStart.bind(null, event, "fg_PopUp"))
}
|
CSS |
.fg_PopUp {
background:#E0E0E0;
box-shadow:10px 10px #BFBFBF;
max-width: fit-content;
position: absolute;
}
.fg_PopUp .fg_Title {cursor:move}
|
Internationalization
The Dictionary pseudo type is intended for form internationalization.
Dict[ionary] dictionaryObject|From function
 | The dictionaryObject global JavaScript variable contains a set of key value items where the key is the word or phrase contained in the widgets list and the value is the translation.
Form ft 'images/faro.ico "Demo internationalization" images/els.png' server echo.php call receive
Dict dict
T Mail Mail address '' hint 'Minimum 6 characters'"
T Protect 'Protected text' value 'Not modifiable Field' disabled
CKB CheckBox 'Send info' 'Check for consent'
T Time '' disabled"
C Comment Comment center"
B Save images/update.png 'inline=In line button' Event click alert 'Not saved, only for demo'"
GET Time getSample.php?Type=Time"
CMB Hellas 'Greek letters' Alfa,Beta,Delta,Epsilon,Gamma
|
(try it yourself) |
Below is a fragment of dictionary used in the form on the left above:
Cancel: "Να κλείσω"
"Check for consent": "Σημειώστε για συγκατάθεση"
Comment: "Σχόλιο"
"Demo internationalization": "Επίδειξη διεθνοποίησης"
"Greek letters": "Ελληνικά γράμματα"
"In line button": "Κουμπί στη γραμμή"
Mail: "ταχυδρομείο"
"Not modifiable Field": "Μη τροποποιήσιμο πεδίο"
Ok: "Εντάξει"
"Protected text": "Προστατευμένο κείμενο"
Reset: "Επαναφορά"
"Send info": "Στείλτε πληροφορίες"
Time: "Τώρα"
Note
- ^This is one of some form generators (for Autoit, Powershell, B4A,B4J, Julia, OCTAVE/MATLAB and TCL/TK) which can be found in my site.
History
- 28th April, 2024: Initial version
Computer literacy (software) : Languages: PHP, Javascript, SQL Autoit,Basic4Android; Frameworks: JOOMLA!
Teaching/Training skills on Office, WEB site development and programming languages.
Others : WEB site development.
UNDP Missions
feb – may 2003 Congo DR Bukavu: ground IT computer course
nov 2003 Burundi Bujumbura: Oracle Data Base course
feb 2005 Burundi Bujumbura: JAVA course
mar 2005 Mali Kati: MS Office course
oct 2006 Mali Kati: MS Office course
jun 2006 Burkina Faso Bobo Dioulasso: MS Office course
jun 2007 Burkina Faso Bobo Dioulasso: MS Office course
may 2007 Argentina Olavarria hospital: Internet application for access to medical records
apr 2008 Burkina Faso Ouagadougou: MS ACCESS and dynamic Internet applications
jun 2008 Niger Niamey: analysis of the computing needs of the Niamey hospital
may 2009 Burkina Faso Ouagadougou: MS ACCESS and dynamic Internet applications
oct 2010 Niger Niamey: analysis of the computing needs of the Niamey hospital (following)
Region Piedmont project Evaluation
mar 2006 Burkina Faso, Niger
mar 2007 Benin, Burkina Faso, Niger
sep 2008 Benin, Burkina Faso, Niger
Others
feb 2010 Burundi Kiremba hospital: MS Office course
feb 2011 Congo DR Kampene hospital: MS Office course