Introduction
In this tip, we will guide you through creating, running and debugging a simple application on Windows 10 IoT Core.
Background
This post is one of a series of posts which I wrote about development applications for Windows 10 IoT Core. To see all my posts, please visit my introduction article:
When you are about to start, you should be on Windows 10 OS (can be even on virtual machine) and you should have Visual Studio 2015 installed. (You can download the community edition for free from here.)
First Steps
First, you open up Visual Studio, and create a new project (File -> New -> Project). Then you choose blank App (Universal Windows).
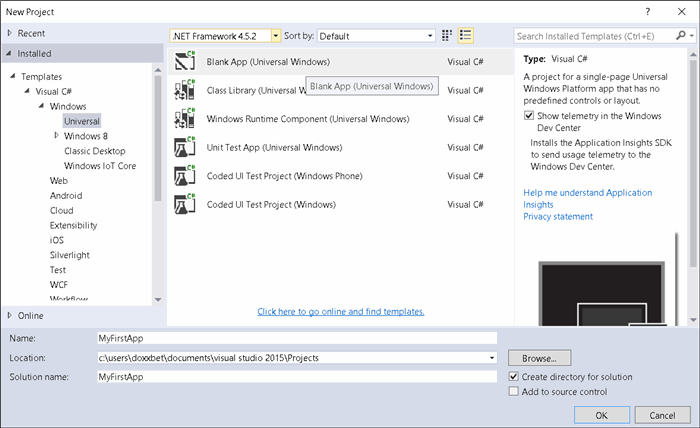
I chose the name "MyFirstApp
". After you click OK, Visual Studio will create a new project for you.

Here, you can see what your new project consists of. So let's start from the top.
- App.xaml - This is the main entry point of the application. Even if it is an xaml file, it has no designer.
- App.xaml.cs - This is the code part from app.xaml, this file is more interesting, it contains code that is executed when the app is started.
- ApplicationInsights.config - Configuration of applicationinsights, which is kind of telemetry for apps from Microsoft.
- MainPage.xaml - This is the main xaml file, this you will see when you will run your application (if you double click on it, visual designer will open)
- MainPage.xaml.cs - This is code file for MainPage.xaml. Here, you will write all code that will be associated to your main screen.
- MyFirstApp_TemporaryKey.pfx - This is the key file used to sign your app package (Universal app package must be signed) usually you don't need to do any modifications on this.
- Package.appxmanifest - Here, you can define basic properties of your application.
- project.json - In this file are described basic app properties, usually you don't need to modify this.
You should understand that Universal windows app is not much like desktop application, it is more like Windows phone application (does not matter if it runs on raspberry or on your local machine). It still has very limited access to system where it is running. Because it runs in sandbox.
After you have looked around the project, you can just click run, first on the local machine to see if everything is OK and if it will compile. (After a while, the program should open in new window, and you can close it.)
To run application on your Raspberry pi, you will need to do some setup.
First, open run options in Visual Studio (as seen in the picture).

Choose Remote Machine and you will see Remote connections window.

Usually your device gets Auto Detected, and you need just to click on its name (in my case miniwinpc) and then you click select. If your device did not get auto detected, you can find its IP address using IOT dashboard and type it in Manual section, important is Authentication Mode, it must be set to "Universal (Unencrypted Protocol)".
Please note that architecture of Raspberry pi processor is ARM, so change it in settings also. After you have set up everything successfully, your run bar should look like this.

Now, you can click green arrow to run your app. In output window, you will see process of deployment of your application.

First build takes quite a while, but you have to be patient. When deployment finishes, your app should be displayed on screen attached to Raspberry pi. Surely at the moment, it is nothing special, but it is something. (You should see a blank white screen with some numbers in corners - performance counters.)
Adding Modifications
Now that you have built and tested your first project on Windows 10 IoT core, it is time to change the application a little bit so you can get hands on it.
First, open MainPage.xaml designer file by double clicking on it. Then set up designer properties to be more accurate to your device. I am using normal fullHD TV so I have it like this:

We will put some image in the background, and then we will add button and text field.
To put image in background, I will first add an image to my project. I select Assets folder and I will add existing object there.

Then in file browser, I will choose some .jpg image to be imported into project. When import is finished, I should be able to see this file in Assets folder.

When I have file ready, I can set it as background of my application. First, I open MainPage.xaml designer by double clicking, and then I select main element and open properties. Go to category Brush
and choose an image, then open dropdown Imagesource
, and choose your new file.

If you do everything correctly in designer your background will change. Next step is to add a button. You can just open toolbox (usually on left) and drag and drop to your application designer button object. I have modified button by changing background color and text in properties window. (to change text on button, you have to modify field "Content
"). Your designer should now look like this:

Ok, now we have a nice background and a button which says, "Click me !". In the next step, we will create some place where we could see result of action performed by button. After clicking on a button, the device will display its IP address on the centre of the screen. So, we will need to add a label, to the centre of the screen. In WPF, you can use TextBox as label, so just find this component in toolbox and drag and drop it into on your main screen. In properties, set text size and fort to bigger I used size 80pt.
When you have this initial design, we can make it work, First, we will add action to a button.
This is very simple, you can just double click on the button and action will be created and you will be redirected to code where you can write classic C# code.
Private void Button_Click(object sender, RoutedEventArgs e)
{
}
Now, we will go back to designer and set name for textBlock
, so we can use it from code. So select your textBlock
and set its name to "textBlock1
".

Now, we can go back to the code and get IP address from system, and then display it in textBlock
, to do it, use this code:
Private void Button_Click(object sender, RoutedEventArgs e)
{
List<string> IPaddr = new List<string>();
var HostNames = Windows.Networking.Connectivity.NetworkInformation.GetHostNames().ToList();
foreach (var Host in HostNames)
{
string IP = Host.DisplayName;
IPaddr.Add(IP);
}
textBlock1.Text = IPaddr.Last();
}
when you finish it, you can run it on your device. If you don't see your background and button same as in designer, probably you have not set correct display resolution on device, to set it please modify config.txt on your SD card and add these values:
hdmi_drive=2
hdmi_mode=82
I use these to get full HD resolution on my TV. You can try another setting, maybe you will get better results.
Then you need to set on your tv aspect ratio to "scan only" and you should see the whole picture.
When your program runs on device successfully, after clicking on button, you should see the IP address of device below.
Please feel free to download the source codes of project and try it on your own. Also, I will be happy if you post any comments or questions.
After study on University of Zilina, I started to work as software developer in this town in Slovakia, I have worked with various technologies, a bit of mobile apps, for android and iOS In latest time I specialize in ASP .NET and .NET C# applications. I like to learn and explore new technologies. Also I like photography and traveling.
I hope with my articles here I could help another developers solve similar problems that I was facing.