Introduction
This demo illustrates how the DevExpress ASP.NET MVC Grid View can be bound to a Model passed to a View from the Controller. The Bind method is used to bind the ASP.NET MVC Grid View to the Model. As a Model for this demo. If you dont have the dev express download the latest version and intall it.
Background
The method's delegate is used to change the text within the text column cells. The method is utilized to create a template for the grid's status bar and to display a refresh message within it.
Using the code
A brief description of how to use this code. The class names, the methods and properties, any tricks or tips.
Add a new project using DevExpress 14.1 all those creating steps are given below:
First you need to add a Quotes.cs class in your Model folder and add some code which is given below:
using System;
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Web;
using System.Web.SessionState;
using System.Xml;
using System.Xml.Linq;
using System.Xml.Serialization;
namespace LiveGrid.Models
{
public class Quotes
{
static readonly Random random = new Random();
public string Symbol { get; set; }
public Decimal Price { get; set; }
public Decimal Change { get; set; }
public Decimal DayMax { get; set; }
public Decimal DayMin { get; set; }
public DateTime LastUpdated { get; set; }
public class Feeds
{
public string Symbol { get; set; }
public string Name { get; set; }
}
public void Update()
{
if (LastUpdated.Day != DateTime.Now.Day)
{
DayMax = 0;
DayMin = 0;
}
Change = (Decimal)((0.5 - random.NextDouble()) / 5.0);
Decimal newPrice = Price + Price * Change;
if (newPrice < 0) newPrice = 0;
if (Price > 0)
Change = (newPrice - Price) / Price;
else
Change = 0;
Price = newPrice;
LastUpdated = DateTime.Now;
if (Price > DayMax || DayMax == 0)
DayMax = Price;
if (Price < DayMin || DayMin == 0)
DayMin = Price;
}
}
public class QuotesProvider
{
static string[] symbolsList = new string[] { "MSFT", "INTC", "CSCO", "SIRI", "AAPL", "HOKU", "ORCL", "AMAT", "YHOO", "LVLT", "DELL", "GOOG" };
static string yahooUrl = "http://finance.yahoo.com/d/quotes.csv?s={0}&f=s0l1h0g0v0d1";
static readonly Random random = new Random();
static HttpSessionState Session
{
get { return HttpContext.Current.Session; }
}
public static List<quotes> GetQuotes()
{
if (Session["Quotes"] == null)
Session["Quotes"] = LoadQuotes();
var quotes = (List<quotes>)Session["Quotes"];
UpdateQuotes(quotes);
return quotes;
}
public static List<quotes> LoadQuotes()
{
try
{
return LoadQuotesFromYahoo();
}
catch
{
return null;
}
}
static List<quotes> LoadQuotesFromYahoo()
{
var quotes = new List<quotes>();
var url = string.Format(yahooUrl, string.Join("+", symbolsList));
var request = HttpWebRequest.Create(url);
using (var stream = request.GetResponse().GetResponseStream())
{
using (var reader = new StreamReader(stream, Encoding.UTF8))
{
while (!reader.EndOfStream)
{
var values = reader.ReadLine().Replace("\"", "").Split(new char[] { ',' });
Quotes quote = new Quotes();
quote.Symbol = values[0].Trim();
Decimal value;
if (Decimal.TryParse(values[1], out value))
quote.Price = value;
else
quote.Price = 0;
if (Decimal.TryParse(values[2], out value))
quote.DayMax = value;
else
quote.DayMax = 0;
if (Decimal.TryParse(values[3], out value))
quote.DayMin = value;
else
quote.DayMin = 0;
DateTime date;
if (DateTime.TryParse(values[4], out date))
quote.LastUpdated = date;
else
quote.LastUpdated = DateTime.Now;
quotes.Add(quote);
}
}
}
return quotes;
}
private static void UpdateQuotes(List<quotes> quotes)
{
foreach(Quotes quote in quotes)
{
if (random.NextDouble() >= 0.7)
quote.Update();
}
}
}
}
After that you have to go to your Index.cshml page and in the source code add a partial view for the grid:
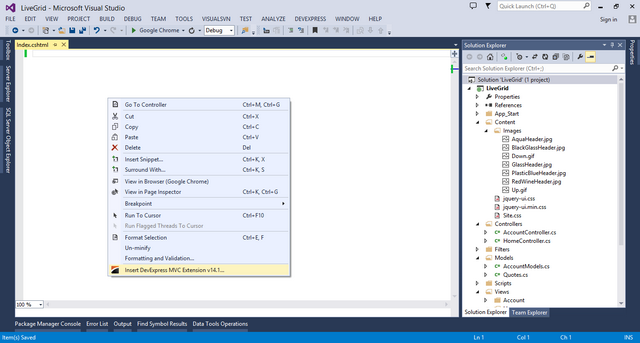
After opening DevExpress MVC Extension go to the Data manu and select GridView from the left pane as you can see i have made an example for you select . Add ExtentionName GridView and PartialViewName QuotesPartial In the data binding section add model class Quotes.cs don't panic with the LiveGrid.Qoutes.cs beacuse Tester is my project name after that check those columns which you want to see in the grid and then select any one column to a keyField.

After adding successfully there is a new View has been added to you Home folder _QuotesPartial.cshtml remove "_" from it like QuotesPartial.cshtml

Now go to QuotesPartial.cshtml and replace all of its code with this:
@Html.DevExpress().GridView(
settings =>
{
settings.Name = "GridView";
settings.CallbackRouteValues = new { Controller = "Home", Action = "QuotesPartial" };
settings.Width = Unit.Percentage(100);
settings.ClientSideEvents.Init = "grid_Init";
settings.ClientSideEvents.BeginCallback = "grid_BeginCallback";
settings.ClientSideEvents.EndCallback = "grid_EndCallback";
settings.Styles.Header.HorizontalAlign = HorizontalAlign.Center;
settings.Settings.GridLines = System.Web.UI.WebControls.GridLines.Vertical;
settings.SettingsBehavior.AllowDragDrop = false;
settings.SettingsPager.Mode = GridViewPagerMode.ShowAllRecords;
settings.SettingsLoadingPanel.Mode = GridViewLoadingPanelMode.ShowOnStatusBar;
settings.Columns.Add("Symbol", "Symbol", MVCxGridViewColumnType.TextBox);
settings.Columns.Add(column =>
{
column.FieldName = "Price";
column.Caption = "Price";
column.Width = 120;
column.ColumnType = MVCxGridViewColumnType.TextBox;
column.PropertiesEdit.DisplayFormatString = "0.00";
});
settings.Columns.Add(column =>
{
column.FieldName = "Change";
column.Caption = "Change";
column.Width = 120;
column.ColumnType = MVCxGridViewColumnType.TextBox;
column.SetDataItemTemplateContent(c =>
{
var text = ((Decimal)DataBinder.Eval(c.DataItem, "Change")).ToString("P");
var imageUrl = Url.Content(string.Format("~/Content/Images/{0}.gif", ((Decimal)DataBinder.Eval(c.DataItem, "Change")) >= 0 ? "Up" : "Down"));
ViewContext.Writer.Write("<img alt='' src='" + imageUrl + "' style='border: 0; margin: 2px 4px;' />"+ text);
});
});
settings.Columns.Add(column =>
{
column.FieldName = "DayMax";
column.Caption = "Day Max";
column.Width = 120;
column.ColumnType = MVCxGridViewColumnType.TextBox;
column.PropertiesEdit.DisplayFormatString = "0.00";
});
settings.Columns.Add(column =>
{
column.FieldName = "DayMin";
column.Caption = "Day Min";
column.Width = 120;
column.ColumnType = MVCxGridViewColumnType.TextBox;
column.PropertiesEdit.DisplayFormatString = "0.00";
});
settings.Columns.Add(column =>
{
column.FieldName = "LastUpdated";
column.Caption = "Last Updated";
column.Width = 120;
column.ColumnType = MVCxGridViewColumnType.DateEdit;
column.CellStyle.HorizontalAlign = HorizontalAlign.Right;
column.PropertiesEdit.DisplayFormatString = "HH:mm:ss";
});
}).Bind(Model).GetHtml()
After replacing that, add two images in your project Content/Images (
),(
) path and now the Final work goto the Index page and add a script on it which is given below:
<script type="text/javascript">
var timeout;
function scheduleGridUpdate(grid) {
window.clearTimeout(timeout);
timeout = window.setTimeout(
function () { grid.Refresh(); },
2000
);
}
function grid_Init(s, e) {
scheduleGridUpdate(s);
}
function grid_BeginCallback(s, e) {
window.clearTimeout(timeout);
}
function grid_EndCallback(s, e) {
scheduleGridUpdate(s);
}
</script>
It will look like this:

One more thing you need to do you have to change in HomeController go to the QuotesPartial()
and remove the model and replace the PartialView model to QuotesProvider.GetQuotes()
:

Now Build you project and run you can see the grid:

This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.