Introduction
Model Oriented Development (MOD) is a technique that allows you to utilize a model for development purposes at any point in the development process. You translate model information into source code and/or utilize the model directly during the course of development. This doesn't imply any particular process or technology. How you represent and utilize your model is completely up to you.
Model Oriented Programming (MOP) involves a process of translating the information in your models into code or whatever materials you need. In order to do this in a most effective manner, I believe that you need a good model oriented programming language.
Modeling in terms of the process of creating and maintaining model data through visual constructs such as diagrams (or the tools to do so) will not be covered here. Some modeling will be covered from a purely programatic standpoint.
In this article, I will present what I feel are necessary qualities for a good model oriented programming language that can allow developers to be able to achieve more with MOD. I am hoping to generate discussion, and would love for you to weigh in on the concepts and materials presented here. I would love to see MOD evolve with a good set of supporting programming practices and languages.
Background
Example model oriented language code will be in Mo+, since the Mo+ language implements nearly all of the concepts outlined in this article. The Mo+ model oriented programming language and the Mo+ Solution Builder IDE for model oriented development was introduced in this Code Project article. Follow that article and related resource links for more information if you want to learn how to use Mo+. Note that Mo+ code will not be displayed in code blocks since there is no similar code block syntax recognition for this language in Code Project.
Model structures will be displayed as a UML class diagrams, where:
- Classes represent objects in the model structure
- Members represent properties of objects in the model structure
- Directed associations represent parent-child relationships in the model structure
Example object oriented code will be in C#.
MOP with decoupled models
I believe that the term Model Oriented Programming (MOP) was first coined by Dr. Timothy Lethbridge. MOP as defined by him and implemented in UMPLE implies that your models are highly coupled with your code in a text-diagram duality.
I am going to alter that definition of MOP for this article with the notion that models can be decoupled from your code, and that a model oriented programming language serves to bridge the gap between your models and your code (and other materials) in any way that you need it to. I believe that decoupling your models from your code has some key advantages for model oriented development:
- Scalability in your work - A model that is directly coupled to your code implies that a single element in your model corresponds to a single element in your code (ex. a class in a class diagram corresponding to a business logic layer class). This does not scale. To get more code out of your model, you have to increase the size of your model. With a model that is decoupled from your code, you can produce multiple, even dozens of code elements from a single element in the model. You make the decisions on how many and what kinds of code or other materials to produce with your model oriented programming.
- Flexibility in your modeling - A model that is directly coupled to your code implies that it's a design level model. You can choose to do detailed modeling at a design level, but you shouldn't have to! Personally, I find that you get the most benefit from modeling while ironing out data and workflows to solidify requirements, then it's simply easier to just program from there. You shouldn't have to do any modeling if you have a source for model information such as a database. In any case, I think you should be free to model to a degree that's right for you. With a decoupled model, you are free to make your design decisions with your model oriented programming.
- Power in your programming - A model that is decoupled from your code places more responsibility on the model oriented programming language to bridge the gap. A more powerful and open language is needed to create your code and materials, and having such a language empowers what you can do as a model oriented programmer.
The model oriented language qualities outlined below will be based on the assumption that models are decoupled from your code. I invite your input on MOP and the notion of coupled vs. decoupled models. Read this You Already Are A Model Oriented Developer article for additional explanation on coupled vs. decoupled MOD.
Models in terms of structure and data
Finally, before we discuss the qualities of a model oriented programming language, we should think of models in terms of structure and data:
- Model Structure - The model structure defines the "rules" or schema by which corresponding models can be organized and built. For example, in a generic entity relationship model, the model structure would include constructs such as Entity, Relationship, and Property.
- Model Data - The model data defines particular instances of the model structure, and gives concrete meaning to the model for a particular purpose. For example, in a generic entity relationship model, the model data could include instances of Entity such as Customer or Order, and instances of Property such as First Name (for Customer) and Order Total (for Order).
Any type of model (UML, ORM, etc.), whether simple or complex, can be described in terms of model structure and model data. Read this Utilizing Model Structures and Data Effectively for Model Oriented Development article for more details on this subject.
Example model structure
The following diagram represents a simple portion of an entity relationship model structure that we will use in the remainder of the article:
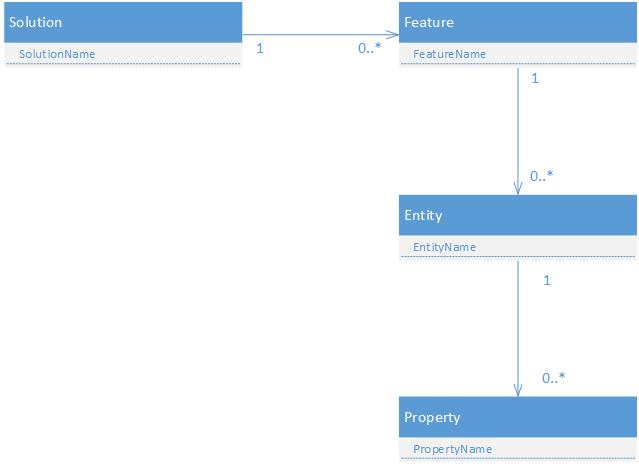
The rules for this model structure are very simple:
- A Solution is the bucket that holds all of the model information, and is described by a SolutionName.
- A Feature is a grouping to organize additional model information, and is described by a FeatureName.
- An Entity is a sort of object or building block, and is described by an EntityName.
- A Property is an attribute used to further describe an Entity, and is described by a PropertyName.
- A Solution can consist of zero or more Features.
- A Feature can consist of zero or more Entity items.
- An Entity can consist of zero or more Property items.
We are also going to utilize this model structure in our object oriented code samples. Here is the model structure represented as simple C# classes:
public class Solution
{
public string SolutionName { get; set; }
public List<Feature> Features { get; set; }
}
public class Feature
{
public string FeatureName { get; set; }
public List<Entity> Entities { get; set; }
}
public class Entity
{
public string EntityName { get; set; }
public List<Property> Properties { get; set; }
}
public class Property
{
public string PropertyName { get; set; }
}
Example model data
We are going to populate this model with just a few items for utilizing the model data:
Solution
: SolutionName
= "My Solution"
Feature
: FeatureName
= "Customers"
-
Entity
: EntityName
= "Customer"
-
Property
: PropertyName
= "FirstName" Property
: PropertyName
= "LastName"
Entity
: EntityName
= "Order"
Property
: PropertyName
= "OrderTotal" Property
: PropertyName
= "ProductName"
Feature
: FeatureName
= "Products"
-
Entity
: EntityName
= "Product"
- Property:
PropertyName
= "ProductName" - Property:
PropertyName
= "Price"
Entity
: EntityName
= "Category"
-
Property
: PropertyName
= "CategoryName"
Qualities of a model oriented programming language
A model oriented programming language is key to letting you employ model oriented programming (MOP) for highly effective model oriented development (MOD). Ultimately, you need to be able to fully utilize your models to create the model based code and materials you want. So, what should such a language look like?
Here are several things I believe are essential qualities of a good model oriented programming language:
- Complete knowledge of model structure
- Complete access to model data
- Ability to utilize model structure to simplify language
- Similarities to an object oriented language
- Ability to update model structure and data
- Ability to effectively produce your code and materials
- Ability to effectively manage your code and materials
Each of these qualities will appear as a separate section below, where we will dig deeper with examples. If you can think of additional qualities you feel a model oriented programming should have, please comment below!
Complete knowledge of model structure
The model oriented language needs to have a complete knowledge of all of the elements of the model structure, otherwise there isn't much you will be able to do with the model. The language should provide keywords with syntax recognition of all of the elements of the model structure.
The example below shows Mo+ keyword syntax recognition of our model elements (in teal text) and other elements that are not in the model (in brown text).
SomethingElse
Solution
Solution.SolutionName
Solution.SomethingElse
Feature
Feature.FeatureName
Feature.SomethingElse
Entity
Entity.EntityName
Entity.SomethingElse
Property
Property.PropertyName
Property.SomethingElse
The model oriented language also needs to know where each of the elements in the model structure fit together in parent/child or other relationships with other elements. Using our example model structure, the language would need to know that SolutionName is an element that describes a Solution.
Complete access to model data
The model oriented language needs to be able to allow you to access the model data in many conceivable ways. Here are a few different ways we need to access model data to be able to do something with it.
General browsing
The model oriented language should be able to iterate and browse through model data in a general way for every kind of element in the model structure. The following Mo+ example shows browsing through all features in a solution, then through all entities in a solution using a foreach
statement (additional features seen in the Mo+ syntax will be explained later).
foreach (Feature)
{
trace(FeatureName)
}
foreach (Entity in Solution)
{
trace(EntityName)
}
Accessing child data
The model oriented language should be able to get to all child model data for a given parent instance. The following Mo+ example shows browsing through all the features, and for each feature browsing through that feature's child entities.
foreach (Feature)
{
foreach (Entity)
{
trace(EntityName)
}
}
Accessing parent data
The model oriented language should be able to get all parent data for a given child instance (all the way up the parent tree). The following Mo+ example shows browsing though all the entities and getting access to the parent feature name for each entity.
foreach (Entity in Solution)
{
trace(FeatureName)
}
Searching for data
The model oriented language should allow you to perform general searches on any element in the model for single or multiple matches based on criteria. Mo+ has rudimentary Find
and FindAll
methods for performing such searches. Below, a Find
single (first) match search is done to do something with a feature called "Customers".
with (Feature from Solution.Find(FeatureName, "Customers"))
{
trace(FeatureName)
}
Filtering data
A nice feature to have is a mechanism to simply filtering through model data while you browse or search. The following Mo+ example shows iterating through entities and using a where
clause to only do something with entities starting with "C".
foreach (Entity in Solution where EntityName.StartsWith("C") == true)
{
trace(EntityName)
}
Ability to utilize model structure to simplify language
I believe that if we look at the general qualities of model structures themselves, we can make use of these qualities to simplify our model oriented language. Consider the following C# code to access some model data:
Solution solution = Helper.GetSolution();
foreach (Feature feature in solution.Features)
{
foreach (Entity entity in feature.Entities)
{
Console.WriteLine(feature.FeatureName + "." + entity.EntityName);
}
}
Now consider the equivalent Mo+ code:
foreach (Feature)
{
foreach (Entity)
{
trace(FeatureName + "." + EntityName)
}
}
Notice that there is no need to define and use variables to track current elements in the foreach
loops.
Model structure has a root
I believe that a model structure has (or should have) a top level root or bucket into which all of the model data can be placed. In our example model structure, the root is Solution
.
I don't think having a real or placeholder root in the model structure places any real constraints on potential model structures. You could have a whole bunch of tailored models in your overall structure (ER models, domain models, user models, hardware models, etc.) that are just tied to your root.
If the assumption holds true that a model structure has a root, then we do not have to explicitly load our model data, we can let the interpreter do it. So, a statement to load the solution such as Helper.GetSolution()
in the C# example is unnecessary, all of the model data should be implicitly available.
Elements in model structure are part of parent-child relationships
I believe that all elements in a model structure (other than the root element) are a child of some other element. Thus, all elements in the model structure are part of parent-child relationships. The parent-child relationships in a model structure can be very shallow (directly to the root) or very deep or otherwise more complex.
To further illustrate this belief, consider the following very simplified portion of the structure for a UML Class Diagram (I am not trying to reproduce the UML metamodel here!):

Models often need to appear in a diagram form to serve as a visual and communication aid. If the model elements were not in parent-child relationships, how could we diagram them? We can put classes, members, associations, and annotations, etc. onto a class diagram because all of these elements are directly or indirectly a child of ClassDiagram.
Certainly, a model element can have multiple child elements (ClassDiagram has child Class and Annotation), and an element can have multiple parent associations to the same or different elements (Association has a parent source Class and a parent destination Class).
If this assumption that model elements are part of parent-child relationships hold true, then we can make some simplifications to the model oriented language:
- Implicit access to children - We can implicitly access child element data for a given instance of a parent element. Going back to the example code above, instead of needing to iterate through solution features explicitly as in C# with variables and members (
foreach (Feature feature in solution.Features)
), we can implicitly iterate through features in our model oriented language (foreach (Feature)
). - Implicit access to parent - Similar to child element data, we can implicitly access parent data for a given child element. Instead of needing to explicitly get the parent feature name with a variable as in C#(
feature.FeatureName
), we can implicitly get our parent feature name in our model oriented language (Feature.FeatureName
or just FeatureName
).
Similarities to an object oriented language
I believe that a model oriented programming language should be analogous to an object oriented language, yet be suited specifically to support MOD. If an object oriented language works with object structures and instances, a model oriented language should work with model structures and instances in a similar manner. Object oriented languages are known for effectively encapsulating data and rules, and having a greater ability to divide and conquer complex problems than procedural languages.
Model oriented properties
The model oriented language should have properties analogous to object oriented properties. Consider the updated Entity
class in our C# representation of the model, with a property called FullName
:
public class Entity
{
public string EntityName { get; set; }
public List<Property> Properties { get; set; }
public Feature Feature { get; set; }
public string FullName
{
get
{
return Feature.FeatureName + "." + EntityName;
}
}
}
We should be able to define and use properties such as this in our model oriented language. In Mo+, properties are essentially blocks of code that are stored as templates (all templates in Mo+, whether used as properties or not, are essentially public). Templates have a name and are associated with a particular type of element in the model. The equivalent code in Mo+ for the FullName
property is an Entity
template called FullName
with the following content code:
<%%=FeatureName + "." + EntityName%%>
The special <%%=%%>
tags are used to emit content from model data. This FullName Entity
template can then be used just like any built in property, including inside expressions:
foreach (Entity in Solution where FullName.Contains("Customer"))
{
trace(FullName)
}
Model oriented methods
Similar to properties, the model oriented language should have methods analogous to object oriented methods. Consider the updated Entity class in our C# representation of the model, with a method called GetExtendedFullName
:
public class Entity
{
public string EntityName { get; set; }
public List<Property> Properties { get; set; }
public Feature Feature { get; set; }
public string FullName
{
get
{
return Feature.FeatureName + "." + EntityName;
}
}
public string GetExtendedFullName(string tag)
{
return tag + "." + FullName;
}
}
We should be able to define and use methods such as this in our model oriented language as well. Mo+ has a rudimentary support for method like calls. Methods are also blocks of code that are stored as templates, but also have additional parameters. Following is the code for the GetExtendedFullName Entity
template:
<%%:
param tag
<%%=tag + "." + FullName%%>
%%>
The GetExtendedFullName Entity
template can also be used just like any built in property, with the addition of passing in the parameter values for a method like call:
foreach (Entity in Solution where GetExtendedFullName(tag="ModelOriented").Contains("Customer"))
{
trace(GetExtendedFullName(tag="ModelOriented"))
}
Model context
Let's take a look at our Entity
class C# example again:
public class Entity
{
public string EntityName { get; set; }
public List<Property> Properties { get; set; }
public Feature Feature { get; set; }
public string FullName
{
get
{
return Feature.FeatureName + "." + EntityName;
}
}
public string GetExtendedFullName(string tag)
{
return tag + "." + FullName;
}
}
When we are inside the body of a non static member, we have direct access to other non static members, and data is based on the context of a given object instance. For example, if our Entity
object instance is "Customer", we can utilize the FullName
property anywhere inside a non static member, and we would get a value of "Customers.Customer".
I believe that a model oriented language should similarly have this kind of context based access to properties and methods, while also making use of the qualities of model structures to perhaps simplify or empower the way it is done. I call this feature model context.
Mo+ implements model context as a stack with the following characteristics:
- Template based - Blocks of Mo+ code are stored as templates, where a particular template is of a certain model element type (such as
Solution
, Entity
, Property
with this example). When the interpreter calls a template, it passes an instance of model data as the single element in the stack. A model context stack's scope is within a template call. - Items on stack are instances of model data - Each item on the stack is an instance of model data. There can be (and usually are) instances of different model element types on the stack.
- Current context - The current context or item is the one on the bottom of the stack.
- Pushing and popping model data - Certain statements such as
foreach
and with
push an instance of model data onto the stack within the scope of their statements. At the end of the statement block, the instance is automatically popped off. - Access up the stack - You can access instances on the stack above the current context with the
../
notation (../../
, etc.).
Now consider the following block of Mo+ code:
foreach (Entity)
{
trace(FullName)
foreach (Feature in Solution)
{
trace(FeatureName)
trace(../FeatureName)
}
with (Feature from Solution.Find(FeatureName, "Customers"))
{
trace(SolutionName + "." + FeatureName)
}
}
Let's examine what happens with model context and how we can make use of it on every step:
- To begin, let's assume that this block of code is within a
Solution
level template. If that's the case, initially the stack contains one item, our Solution
called "My Solution". Our current context starts out with this solution. - At the
foreach (Entity)
statement, which iterates through all entities in our current context (solution), the following happens:
- The current
Entity
in the iteration (such as "Customer") is pushed onto the stack and becomes the current context. By doing this, we don't need a temporary variable to store the current entity. - At the end of the
foreach
statement block, the current Entity
is popped off of the stack.
- At the
trace(FullName)
statement, the following happens:
- The current context is an
Entity
(such as "Customer"), so we can directly access anything Entity
related. We choose to call the Entity
level template called FullName
. - When calling
FullName
, the interpreter passes the current context (Entity
) to start the stack for processing that template call. That template can do whatever manipulations of its context stack and not affect this template's stack.
- At the
foreach (Feature in Solution)
statement, we are iterating through each feature in the solution, pushing (and popping) a Feature
on the stack within the scope of that foreach
statement block. Note that if we didn't add in Solution
here, we would look for all features in the current context (Entity
), of which there would be none since Feature
is not a child of Entity
. - At the
trace(FeatureName)
statement, the current context is a Feature
(such as "Customers"), and we have access to anything Feature
related. - At the
trace(../FeatureName)
statement, we are accessing the item one up the stack, which is an Entity
from our first foreach
statement. We choose to get the feature name for that entity which may be different than the current context feature's name. - At the
with (Feature from Solution.Find(FeatureName, "Customers"))
statement, the following happens:
- A search is performed to match a single
Feature
called "Customers", and this Feature
is pushed onto the stack and becomes the current context. Again, no temporary variables are needed to store the current feature. - At the end of the with statement block, the current
Feature
is popped off of the stack.
- At the
trace(SolutionName + "." + FeatureName)
statement, the current context is the Feature
called "Customers", and we have access to anything Feature
related. - Finally, at the end of these statements, the stack should only have one item, the
Solution
("My Solution") that the template was originally called with. The stack should never be empty within a block of code.
Following is similar code to do this in C#. Notable differences include explicitly needing variables to track context within loops, explicitly needing to manage separate lists at higher levels in the model (need an explicit list of entities at the solution level), and explicitly needing to manage references to parent information (need an explicit reference to Feature
from an Entity
):
foreach (Entity entity in solution.Entities)
{
Console.WriteLine(entity.FullName);
foreach (Feature feature in solution.Features)
{
Console.WriteLine(feature.FeatureName);
Console.WriteLine(entity.Feature.FeatureName);
}
foreach (Feature feature in solution.Features.Where(i => i.FeatureName == "Customers"))
{
Console.WriteLine(solution.SolutionName + "." + feature.FeatureName);
}
}
Additional similarities
What other similarities do you think a model oriented language should have with an object oriented language?
Ability to update model structures and data
The model oriented language should also give you the ability to modify your model structures and data.
Updating model data
First, we will talk about model data. Up to now, we have only talked about reading model data. But, I think that it's important for a model oriented language to be able to also write to and update your model data. This is epecially important if you want to import model data from somewhere else, where you will need your model oriented language to significantly reduce your modeling effort.
Mo+ has a rudimentary syntax, but powerful method for adding and updating model data. Writing to model data is only allowed in the output area of specification templates. With a current instance of a given element in the model structure, you can create a new instance (with New()
method), add it to the model (with add
statement), and/or merely update properties of that instance.
CurrentProperty = New()
CurrentProperty.PropertyName = "My Property"
add(CurrentProperty)
CurrentProperty.PropertyName = "My Updated Property"
Updating model structures
What about model structures? Most model oriented programmers may only need to work with standard structures such as UML or ER structures, but the model oriented language should give you the power to create and use your own structures or add to existing structures if you ever need to do so.
Let's say for example that we want to add an object to our model structure called Block
, with a property called BlockName
. The model oriented language should be able to allow you to add any additional structure you want, and should have keywords with syntax recognition for each of these elements that you create.
Mo+ has a rudimentary (and perhaps convoluted) method for creating any model structures of your choosing. As with updating model data, this is done in the output area of specification templates. The following Mo+ code shows some of the steps for adding Block
and BlockName
.
CurrentModelObject = New()
CurrentModelObject.ModelObjectName = "Block"
add(CurrentModelObject)
var blockObjectID = CurrentModelObject.ModelObjectID
CurrentModelProperty = New()
CurrentModelProperty.ModelPropertyName = "BlockName"
CurrentModelProperty.ModelObjectID = blockObjectID
add(CurrentModelProperty)
Then, Block
and BlockName
become recognized pieces of your model structure and can be used in the same way as other model elements:
foreach (Block)
{
trace(BlockName)
}
Read this Utilizing Model Structures and Data Effectively for Model Oriented Development article for more details on utilizing custom model structures.
Ability to effectively produce your code and materials
I am assuming at this point that a primary purpose of MOD and a model oriented language supporting it is that you need to produce source code and other materials from your model.
For generating code and other materials, you generally need to merge model data with other information to translate your model into something else. So I believe that a model oriented language should have some sort of templating mechanism to allow you to merge this data.
Mo+ templates support tags that allow you to delineate between processing, raw content, and model oriented content. Mo+ tags begin with <%%:
(for processing), <%%-
(for raw content), and <%%=
for model oriented content, and end with %%>
. See the following example (which we will call ExampleReport
, a Solution
level template):
<%%:
foreach (Entity)
{
<%%-
The full name for this entity is %%><%%=FullName%%><%%-.%%>
}
%%>
In addition to syntax recognition for statements (in blue), model elements (in teal), and templates (in brown), Mo+ recognizes raw content as orange text. The content for this template when called is:
The full name for this entity is Customers.Customer.
The full name for this entity is Customers.Order.
The full name for this entity is Products.Category.
The full name for this entity is Products.Product.
Ability to effectively manage your code and materials
Actually managing your code and materials is more that just merely producing it. Most model oriented approaches that generate code do so blindly, regenerating everything on every update pass, and give you little control on where stuff goes. This isn't very useful, especially in large team environments. A model oriented language should give you full control in determining when, where, and how to update your code and materials.
Mo+ templates have an output section that not only gives you this full control, but allows you to do so in a model oriented way. Going back to our ExampleReport
template above, consider the following code to determine the output of this template:
<%%:
var path = "c:\\temp\\ExampleReport.txt"
if (File(path) == null)
{
update(path)
}
%%>
Basically, this says that if there is no file at the path
location, create it (with the update
statement), with the content of this template (as shown above). If the file already exists, then it is not regenerated. Obviously, your real conditions for updating a generated file will usually be more than this.
What makes this model oriented is that this template is in control of its own destiny. Another template could choose to induce ExampleReport
to output something with a statement such as:
<%%>ExampleReport%%>
Even so, ExampleReport
won't update its report file unless the conditions are met. Managing output in this manner becomes very effective and maintainable on a large scale.
Additional language features
Here are some additional features that I think a good model oriented language should have (most of these features are supported at least in part by Mo+):
- Ability to handle any model structure from the most simple to the most complex, including any constraints or rules such as whether the structure allows duplicate names for elements
- Ability to handle any amount of model data from small to large, including any constraints or rules such as naming constraints
- Ability to decouple network architectural details and allowing compilers/interpreters to map to those details (this one requires much more thought and evolution than I have considered so far)
- Ability to configure customization points so custom code can be directly maintained with generated code in the same files
- Ability to pull and filter existing files for help on decisions to update generated content
- Ability to generate updates to additional resources other than files, such as databases
- Ability to process directories to remove outdated generated files
- Ability to import model information from XML and various popular databases
- Ability to import model information from other popular modeling tools
- Ability to export model information to other sources
- Ability to read information from database records as well as schema
- A full suite of string testing and manipulation methods
- Ability to configure basic formatting for output such as tabs and spaces
- Of course, ability to add comments!
Do you think a model oriented language should be type safe or not? Mo+ is not type safe (with var being your only variable data type), freely letting you use data between string and non string formats, which I think is more useful for code generation purposes.
What other features and qualities in general do you think a model oriented language should have?
Sample code
The attached download contains a Visual Studio solution with some of the sample object oriented code in it, and a Mo+ solution (xml) file with a few associated code templates. The focus of this article was not on doing something specific with the code, but these samples may be of use to you while following the article.
In Summary
Hopefully this article has provided you with some food for thought on what a good model oriented programming language should look like to allow you as a programmer to get the most out of model oriented development. Again, I hope you weigh in with your thoughts on the subject in the comments section below.
I have one parting thought for you to ponder. In 1969 we landed and stepped on the moon. I would have thought by now that we would have similarly landed on Mars, but somehow we didn't have enough dedication and drive to do so. Similary, by the 1960s the software industry developed procedural languages, allowing us to solve problems decoupled from the details of CPU architectures. By the 1980s, the industry developed object oriented languages, giving us more power to solve problems and in a way that can be more closely expressive to business needs. I would have thought by now that the industry would have developed languages that would allow us to solve problems in even more business oriented ways and decoupled from platform and network architectural details. I would think that such languages would be model oriented ones. Why are we still programming to network architectural details such as choice of cloud platform (AWS, Azure), choice of DBMS (SQL Server, PostgreSQL), etc., when such decisions could be made and changed at deployment time and interpreted/compiled from higher order languages? Why hasn't the industry made more headway on such languages? We currently are solving some of these problems with frameworks, but frameworks come and go and we have to keep updating our programming to new and better ones.
In addition, I hope you try Mo+ to fully utilize incorporating a model oriented approach to your development, using an initial attempt at a good model oriented programming language. Mo+ has a built in flexible generic entity-relationship type of model structure, but it also allows you to create and use your own model structures (or recreate other standardized structures if you choose). The free, open source product is available at moplus.codeplex.com or github.com/moplus/modelorientedplus. In addition to the product, this site contains sample packs for building more complete models and generating complete working applications. Video tutorials and other materials are also available on the codeplex site, as well as online help in the IDE. The Mo+ community gets additional support, and contributes to the evolution of Mo+ via the web site at https://modelorientedplus.com.