Introduction
This article explains how to load data on demand or load data when the user scrolls. Here, we will do this in a simple manner. I hope you enjoy it.
Background
A few days ago, one of my colleagues asked me how to load data into the UL when we do scroll. I could help him at that time itself. But here, I am writing this article for his future reference. In this way, we can do this very easily.
Using the Code
Since we will load the data on a scroll, we need to fire the window events. So I suggest we include a jQuery plug in in your page as in the following:
<script src="jquery-2.1.4.min.js"></script>
Once you are done, you are ready to go!
Our next step is to load some initial or static data.
<div id="myScroll">
<p>
Contents will load here!!!.<br />
</p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
</div>
Now we need some styles, right?
<style>
#myScroll {
border: 1px solid #999;
}
p {
border: 1px solid #ccc;
padding: 5px;
text-align: center;
}
.loading {
color: red;
}
.dynamic {
background-color:#ccc;
color:#000;
}
</style>
What is next? Shall we run it and see our page now?
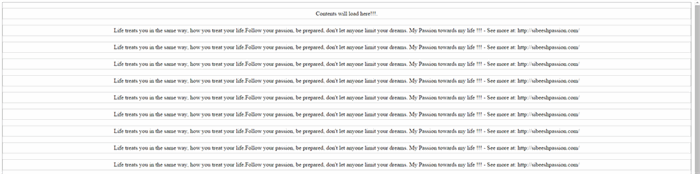
So now we have loaded the static data. Now it is time to fire the scroll
event.
$(window).scroll(function () {
if ($(window).scrollTop() == $(document).height() – $(window).height()) {
appendData();
}
});
In the preceding code, I am calling the scroll
event and when the condition $(window).scrollTop() == $(document).height() – $(window).height()
is satisfied, I am calling a function. You can see the function below:
function appendData() {
var html = ";
for (i = 0; i < 10; i++) {
html += ‘<p class="dynamic">Dynamic Data :
Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, do not let anyone limit your dreams.
My Passion towards my life !!! – See more at:
<a href="http:
</p>’;
}
$(‘#myScroll’).append(html);
}
Here, I am populating 10 records dynamically and appending the created data to our main element. Once you are done, you can see the output as in the following:

Complete Code
<!DOCTYPE html>
<html>
<head>
<title>Load data on demand demo – Sibeesh Passion</title>
<script src="jquery-2.1.4.min.js"></script>
<style>
#myScroll {
border: 1px solid #999;
}
p {
border: 1px solid #ccc;
padding: 5px;
text-align: center;
}
.loading {
color: red;
}
.dynamic {
background-color:#ccc;
color:#000;
}
</style>
<script>
$(window).scroll(function () {
if ($(window).scrollTop() == $(document).height() – $(window).height()) {
appendData();
}
});
function appendData() {
var html = ";
for (i = 0; i < 10; i++) {
html += ‘<p class="dynamic">Dynamic Data :
Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, do not let anyone limit your dreams.
My Passion towards my life !!! – See more at:
<a href="http:
</p>’;
}
$(‘#myScroll’).append(html);
}
</script>
</head>
<body>
<div id="myScroll">
<p>
Contents will load here!!!.<br />
</p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
<p >Life treats you in the same way, how you treat your life.
Follow your passion, be prepared, don’t let anyone limit your dreams.
My Passion towards my life !!! – See more at: http://sibeeshpassion.com/ </p>
</div>
</body>
</html>
Conclusion
Please download the source code for more details. I hope you liked this article. Now please share your thoughts and suggestions. It matters a lot.