Introduction
Before a while, I needed to implement rendering of numbers in one project (C++ MFC), where it wasn't possible to simply use rendering of text using font. So I made the prototype in C# WinForms and maybe someone will find it useful.
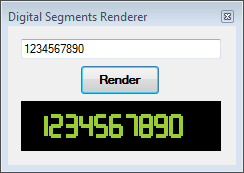
Background
I'm using classical old-school approach -> 7-segments rendering of numbers.
Source Code
I've created SegmentRender
class for rendering of segmented numbers.
At first, I've created Enum
of segment type for better orientation.
private enum Segment
{
Up, LeftUp, RightUp, Middle, LeftDown, RightDown, Down
}
Here is simple 2D array [10 digits, 7 segments] for definition of segments used for each digit:
static int[,] segValues =
{
{1, 1, 1, 0, 1, 1, 1},
{0, 0, 1, 0, 0, 1, 0},
{1, 0, 1, 1, 1, 0, 1},
{1, 0, 1, 1, 0, 1, 1},
{0, 1, 1, 1, 0, 1, 0},
{1, 1, 0, 1, 0, 1, 1},
{1, 1, 0, 1, 1, 1, 1},
{1, 0, 1, 0, 0, 1, 0},
{1, 1, 1, 1, 1, 1, 1},
{1, 1, 1, 1, 0, 1, 1}
};
Then I defined 3 functions:
public void DrawNumber(int n, Graphics g, Point pos)
Here, the maximal order of our Int32
number is found:
while (true)
if (n < Math.Pow(10, ++maxOrder))
break;
Then, each single digit is found and called to be rendered:
for (int ord = 0; ord < maxOrder; ord++)
{
int dig = n / Convert.ToInt32(Math.Pow(10, ord)) % 10;
Point digPos = new Point
(pos.X + (maxOrder - ord - 1) * (size + space), pos.Y);
DrawDigit(dig, g, digPos);
}
The next function:
private void DrawDigit(int n, Graphics g, Point pos)
In this function, all single digits are called to be rendered:
for (int i = 0; i < 7; i++)
if (segValues[n, i] == 1)
DrawSegment((Segment)i, g, pos);
And the last rendering function:
private void DrawSegment(Segment s, Graphics g, Point pos)
Here I've defined all segments points (x
, y
) and rendered these segments, which are for actual digit enabled in variable segValues
.
That's all, simple enough. The rest of the code is only handling of the application form.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.