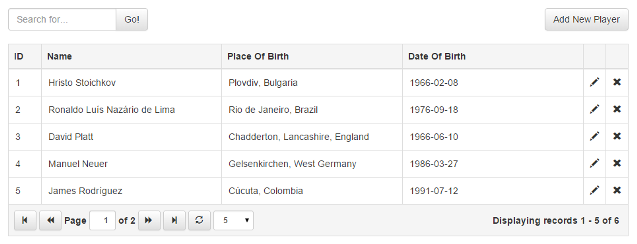
Introduction
This tip is going to show how you can easily implement paging, sorting, filtering and CRUD operations with jQuery Grid Plugin in ASP.NET MVC with bootstrap.
Background
In the sample project that you can download from this tip, I'm using jQuery Grid 0.4.3 by gijgo.com, jQuery 2.1.3, Bootstrap 3.3.4 and AspNet.Mvc 5.2.3.
A Few Words about jQuery Grid by gijgo.com
Since the other libraries that are in use are pretty popular compared to the grid plugin. I'm going to give you some information about this plugin.
- Stylish and Featured Tabular data presentation control
- JavaScript control for representing and manipulating tabular data on the web
- Ajax enabled
- Can be integrated with any of the server-side technologies like ASP, JavaServlets, JSP, PHP, etc
- Very simple to integrate with ASP.NET
- Support pagination, JavaScript and server side data sources
- Support jQuery UI and Bootstrap
- Free open source tool distributed under MIT License
You can find the documentation about the version of the plugin that is in use in this tip at http://gijgo.com/version_0_4/Documentation.
Integrating jQuery Grid with ASP.NET MVC - Step By Step
- Create a new ASP.NET MVC project in Visual Studio.
- I assume that jquery and bootstrap would be added to your ASP.NET MVC project by default. If they are not added, you can find and add them to your project via nuget.
- Add jQuery Grid by gijgo.com via nuget. You can find more information at https://www.nuget.org/packages/jQuery.Grid/.
- Make sure that you have a reference to jquery.js, bootstrap.css, grid.css and grid.js files in the pages where you are planning to use the jquery grid.

In order to use the grid plugin, you will need a HTML table tag for a base element of the grid. I recommend to use the "data-source
" attribute of the table as identification for the location of source url on the server side.
<table id="grid" data-source="@Url.Action("GetPlayers")"></table>
After that, we have to initialize the table as jquery grid with the fields which we are planning to display in the grid
.
grid = $("#grid").grid({
dataKey: "ID",
uiLibrary: "bootstrap",
columns: [
{ field: "ID", width: 50, sortable: true },
{ field: "Name", sortable: true },
{ field: "PlaceOfBirth", title: "Place Of Birth", sortable: true },
{ field: "DateOfBirth", title: "Date Of Birth", sortable: true },
{ field: "Edit", title: "", width: 34, type: "icon",
icon: "glyphicon-pencil", tooltip: "Edit", events: { "click": Edit } },
{ field: "Delete", title: "", width: 34, type: "icon",
icon: "glyphicon-remove", tooltip: "Delete", events: { "click": Remove } }
],
pager: { enable: true, limit: 5, sizes: [2, 5, 10, 20] }
});
If you want to be able to sort by particular column, you need to set the sortable option of the column to true
. When you do that, the grid plugin is going to send information to the server about the field name that needs to be sorted. In order to configure paging, you have to use the pager option from where you can control the paging.
In the sample project, I use the following code to implement simple CRUD operations over the data inside the grid
.
function Add() {
$("#playerId").val("");
$("#name").val("");
$("#placeOfBirth").val("");
$("#dateOfBirth").val("");
$("#playerModal").modal("show");
}
function Edit(e) {
$("#playerId").val(e.data.id);
$("#name").val(e.data.record.Name);
$("#placeOfBirth").val(e.data.record.PlaceOfBirth);
$("#dateOfBirth").val(e.data.record.DateOfBirth);
$("#playerModal").modal("show");
}
function Save() {
var player = {
ID: $("#playerId").val(),
Name: $("#name").val(),
PlaceOfBirth: $("#placeOfBirth").val(),
DateOfBirth: $("#dateOfBirth").val()
};
$.ajax({ url: "Home/Save", type: "POST", data: { player: player } })
.done(function () {
grid.reload();
$("#playerModal").modal("hide");
})
.fail(function () {
alert("Unable to save.");
$("#playerModal").modal("hide");
});
}
function Remove(e) {
$.ajax({ url: "Home/Remove", type: "POST", data: { id: e.data.id } })
.done(function () {
grid.reload();
})
.fail(function () {
alert("Unable to remove.");
});
}
function Search() {
grid.reload({ searchString: $("#search").val() });
}
Server Side
In the Controller, we need only 4 methods, Index
, GetPlayers
, Save
and Remove
.
[NoCache]
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpGet]
public JsonResult GetPlayers(int? page, int? limit,
string sortBy, string direction, string searchString = null)
{
int total;
var records = new GridModel().GetPlayers
(page, limit, sortBy, direction, searchString, out total);
return Json(new { records, total }, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public JsonResult Save(Player player)
{
new GridModel().Save(player);
return Json(true);
}
[HttpPost]
public JsonResult Remove(int id)
{
new GridModel().Remove(id);
return Json(true);
}
}
Please note that I'm using custom "[NoCache]
" attribute for the controller, that is going to resolve some issues with the caching. I recommend the usage of such attribute or similar mechanism for prevention of bugs related to caching.
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method)]
public sealed class NoCacheAttribute : ActionFilterAttribute
{
public override void OnResultExecuting(ResultExecutingContext filterContext)
{
filterContext.HttpContext.Response.Cache.SetExpires(DateTime.UtcNow.AddDays(-1));
filterContext.HttpContext.Response.Cache.SetValidUntilExpires(false);
filterContext.HttpContext.Response.Cache.SetRevalidation(HttpCacheRevalidation.AllCaches);
filterContext.HttpContext.Response.Cache.SetCacheability(HttpCacheability.NoCache);
filterContext.HttpContext.Response.Cache.SetNoStore();
base.OnResultExecuting(filterContext);
}
}
In the data model of this example, I use XML as data store in order to simplify the logic in the model. You can customize the data model as you want and replace my implementation with code that is using relational databases like Microsoft SQL Server, My SQL or other services that are specific for your project.
I hope that this tip is going to be useful for your project. Happy coding!
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.