Introduction
This code works well with a GMail account.
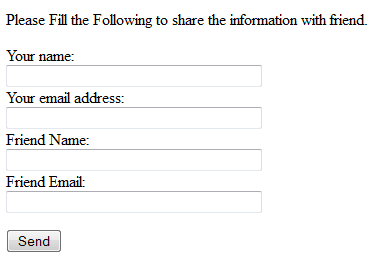
Background
This code is based on the 3.5 and 4.0 frameworks.
Using the code
HTML:
<asp:Panel ID="Panel1" runat="server" DefaultButton="btnSubmit">
<p>
Please Fill the Following to share the information with friend.</p>
<p>
Your name:
<asp:RequiredFieldValidator ID="RequiredFieldValidator11"
runat="server" ErrorMessage="*"
ControlToValidate="YourName" ValidationGroup="save" /><br />
<asp:TextBox ID="YourName" runat="server" Width="250px" /><br />
Your email address:
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="*"
ControlToValidate="YourEmail" ValidationGroup="save" /><br />
<asp:TextBox ID="YourEmail" runat="server" Width="250px" />
<asp:RegularExpressionValidator runat="server" ID="RegularExpressionValidator23"
SetFocusOnError="true" Text="Example: username@gmail.com" ControlToValidate="YourEmail"
ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*" Display="Dynamic"
ValidationGroup="save" /><br />
Friend Name:
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ErrorMessage="*"
ControlToValidate="txtboxFname" ValidationGroup="save" /><br />
<asp:TextBox ID="txtboxFname" runat="server" Width="250px" /><br />
Friend Email:
<asp:RequiredFieldValidator ID="RequiredFieldValidator3" runat="server" ErrorMessage="*"
ControlToValidate="txtboxFriendEmail" ValidationGroup="save" /><br />
<asp:TextBox ID="txtboxFriendEmail" runat="server" Width="250px" />
<asp:RegularExpressionValidator runat="server"
ID="RegularExpressionValidator1" SetFocusOnError="true"
Text="Example: username@gmail.com"
ControlToValidate="txtboxFriendEmail"
ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"
Display="Dynamic" ValidationGroup="save" />
</p>
<p>
<asp:Button ID="btnSubmit" runat="server"
Text="Send" OnClick="Button1_Click" ValidationGroup="save" />
</p>
</asp:Panel>
<p>
<asp:Label ID="lblMsgSend" runat="server" Visible="false" />
</p>
The server side code:
protected void SendMail()
{
var fromAddress = YourEmail.Text.ToString();
var toAddress = txtboxFriendEmail.Text.ToString();
const string toPassword = "Password";
string subject = YourName.Text.ToString() + " want to share the information";
string body = "From: " + YourName.Text + "\n";
body += "Email: " + YourEmail.Text + "\n";
body += "To: " + txtboxFname.Text + "\n";
body += "Message: \n Dear " + txtboxFname.Text +
"\n your friend: " + YourName.Text +
" want to share the information about the visa services " +
"of University of South Asia \n please visit " +
"the website www.usa.edu.pk \n than visa services";
var smtp = new System.Net.Mail.SmtpClient();
{
smtp.Host = "smtp.gmail.com";
smtp.Port = 587;
smtp.EnableSsl = true;
smtp.DeliveryMethod = System.Net.Mail.SmtpDeliveryMethod.Network;
smtp.Credentials = new NetworkCredential("address@gmail.com",
toPassword);
smtp.Timeout = 20000;
}
smtp.Send(fromAddress, toAddress, subject, body);
}
protected void Button1_Click(object sender, EventArgs e)
{
try
{
SendMail();
lblMsgSend.Text = "Your message send successfully to your friend";
lblMsgSend.Visible = true;
txtboxFriendEmail.Text = "";
YourEmail.Text = "";
YourName.Text = "";
txtboxFname.Text = "";
}
catch (Exception) { }
}
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.