Unsheathing the Swiss Army Web Part (Almost as Impressive as a "Sure-'Nuff Barlow Knife")
WebParts can be edited by the user to a certain extent. Developers can extend what the user can edit by writing custom code for this purpose. For example, you could, in a section of the WebPart editor, allow the user to assign the (textual) value of a label you have added to the WebPart. Additionally, you can allow the user to determine which elements, or sections, of a WebPart, display. In this way, you can create a WebPart that does double-, triple, or up to million-duty (theoretically), depending on their needs at a particular time/on a particular page.
Here is how that can be done. This assumes you already have a WebPart, and have added a Visual Web Part to it. If not, create a new Empty Sharepoint project in VS 2010, and then Add > New Item... > Visual WebPart.
In the WebPart code-behind (the <whateveryounamedyourwebpart>.cs file), add code like so:
[ToolboxItemAttribute(false)]
public class DPSVisualWebPart : WebPart
{
[WebBrowsable(true),
Category("CustomUCSC"),
Personalizable(PersonalizationScope.Shared),
WebDisplayName("WebPart Title Text")]
public string CustomTitleProp { get; set; }
[WebBrowsable(true),
Category("CustomUCSC"),
Personalizable(PersonalizationScope.Shared),
WebDisplayName("Generate Section1")]
public bool CheckboxGenSection1 { get; set; }
. . . checkboxes 2 through 5 elided in the interests of brevity
[WebBrowsable(true),
Category("CustomUCSC"),
Personalizable(PersonalizationScope.Shared),
WebDisplayName("Generate Section6")]
public bool CheckboxGenSection6 { get; set; }
private const string _ascxPath =
@"~/_CONTROLTEMPLATES/DirectPaymentSectionsWebPart/DPSVisualWebPart/DPSVisualWebPartUserControl.ascx";
public bool genSection1 { get; set; }
public bool genSection2 { get; set; }
public bool genSection3 { get; set; }
public bool genSection4 { get; set; }
public bool genSection5 { get; set; }
public bool genSection6 { get; set; }
protected override void CreateChildControls()
{
DPSVisualWebPartUserControl control = Page.LoadControl(_ascxPath) as DPSVisualWebPartUserControl;
if (control != null)
{
control.dpsvisWebPart = this;
}
Controls.Add(control);
}
}
The example above should be pretty self-explanatory; the code is fairly sparse; it adds controls to the new custom section of the WebPart Editor (CustomUCSC in this case). Here a label in which the user can enter what they want the first label on the WebPart to say, and six Checkboxes, which are checked/unchecked to determine which portions/sections of the WebPart will be dynamically generated and displayed, are cretad. Obviously, which controls you add to the WebPart Editor (as well as what you do in response to them) will vary depending on your particular WebPart, needs, requirements, etc.
Note: If you wanted to show one and only one Section for each instance of the WebPart, you could use a Dropdown List in lieu of a gaggle (which is more than a couple, but fewer than a googleplex) of checkboxes. That may look something like this:
public enum ddlSectionsEnum { section1, section2, section3, section4, section5, section6 }
[WebBrowsable(true),
Category("CustomUCSC"),
Personalizable(PersonalizationScope.Shared),
WebDisplayName("Section to Display")]
public ddlSectionsEnum ddlProp { get; set; }
The pertinent (CustomUCSC) part of the WebPart Editor now looks like this (after deploying):
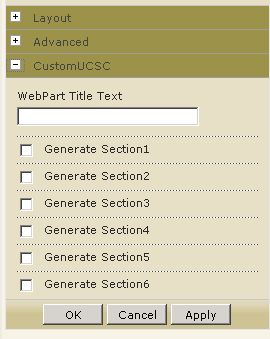
When adding the WebPart to a page it looks like this:

Admittedly, there's not much to it. But now, if you enter a title to use and select a section in the CustomUCSC portion of the Web Part Editor:

...you will then see something more interesting on the WebPart:

How did this happen? It's either magic, or I haven't shown you some of the code yet.
In the WebPart User Control code-behind (the <whateveryounamedyourwebpart>UserControl.ascx.cs file), I added the following code for that "magic" to take place. I have added pertinent comments (encase in brackets "// [like this]") to show the purpose of the various pieces of code.
public partial class DPSVisualWebPartUserControl : UserControl
{
public DPSVisualWebPart dpsvisWebPart { get; set; }
CheckBox ckbx204Submitted = null;
TextBox boxRequestDate = null;
TextBox boxPaymentAmount = null;
TextBox boxPayeeName = null;
TextBox boxRemitAddress = null;
TextBox boxMailStop = null;
TextBox boxSSNOrITIN = null;
TextBox boxRequesterName = null;
TextBox boxDeptDivName = null;
TextBox boxPhone = null;
TextBox boxEmail = null;
protected void Page_Load(object sender, EventArgs e)
{
}
protected override void OnPreRender(EventArgs e)
{
base.OnPreRender(e);
if (this.dpsvisWebPart != null && this.dpsvisWebPart.CustomTitleProp != null)
{
lbl_Title.Text = String.Format("<h1>{0}</h1>", this.dpsvisWebPart.CustomTitleProp.ToString());
if (this.dpsvisWebPart.CheckboxGenSection1)
{
GenerateSection1();
}
if (this.dpsvisWebPart.CheckboxGenSection2)
{
GenerateSection2();
}
}
}
private void GenerateSection1()
{
var section1Hdr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "<h2>Section 1: Payment Information</h2>"
};
this.Controls.Add(section1Hdr);
HtmlTable tblSection1 = null;
tblSection1 = GetSection1Table();
this.Controls.Add(tblSection1);
String checkboxText = "<span class=\"finaff-webform-field-label\">204 submitted or on file.
<strong>NOTE:</strong> If not on file, complete a <a href=
\"https://financial.ucsc.edu/Financial_Affairs_Forms/Payee_Setup_204.pdf\" target=\"_blank
\">Payee_Setup_204</a></span>";
ckbx204Submitted = new CheckBox();
ckbx204Submitted.Text = checkboxText;
this.Controls.Add(ckbx204Submitted);
}
private HtmlTable GetSection1Table()
{
HtmlTable dynamicTable = new HtmlTable();
var row1 = new HtmlTableRow();
var cellLitCtrl_1 = new HtmlTableCell();
var cellTxtbx_1 = new HtmlTableCell();
row1.Cells.Add(cellLitCtrl_1);
row1.Cells.Add(cellTxtbx_1);
var reqDateStr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "Requester Date:"
};
cellLitCtrl_1.Controls.Add(reqDateStr);
boxRequestDate = new TextBox
{
CssClass = "finaff-webform-field-input",
Text = DateTime.Today.ToShortDateString()
};
cellTxtbx_1.Controls.Add(boxRequestDate);
dynamicTable.Rows.Add(row1);
var row7 = new HtmlTableRow();
var cellLitCtrl_7 = new HtmlTableCell();
var cellTxtbx_7 = new HtmlTableCell();
row7.Cells.Add(cellLitCtrl_7);
row7.Cells.Add(cellTxtbx_7);
var SSNOrITINStr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "Last 4 Digits SSN or ITIN:"
};
cellLitCtrl_7.Controls.Add(SSNOrITINStr);
boxSSNOrITIN = new TextBox
{
CssClass = "finaff-webform-field-input"
};
cellTxtbx_7.Controls.Add(boxSSNOrITIN);
dynamicTable.Rows.Add(row7);
return dynamicTable;
}
private void GenerateSection2()
{
HtmlTable tblSection2 = null;
var section2Hdr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "<h2>Section 2: Requester Information</h2>"
};
this.Controls.Add(section2Hdr);
tblSection2 = GetSection2Table();
this.Controls.Add(tblSection2);
}
private HtmlTable GetSection2Table()
{
HtmlTable dynamicTable = new HtmlTable();
var row1 = new HtmlTableRow();
var cellLitCtrl_1 = new HtmlTableCell();
var cellTxtbx_1 = new HtmlTableCell();
row1.Cells.Add(cellLitCtrl_1);
row1.Cells.Add(cellTxtbx_1);
var reqNameStr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "Requester Name:"
};
cellLitCtrl_1.Controls.Add(reqNameStr);
boxRequesterName = new TextBox
{
CssClass = "finaff-webform-field-input"
};
cellTxtbx_1.Controls.Add(boxRequesterName);
dynamicTable.Rows.Add(row1);
var row4 = new HtmlTableRow();
var cellLitCtrl_4 = new HtmlTableCell();
var cellTxtbx_4 = new HtmlTableCell();
row4.Cells.Add(cellLitCtrl_4);
row4.Cells.Add(cellTxtbx_4);
var emailStr = new Label
{
CssClass = "finaff-webform-field-label",
Text = "Email:"
};
cellLitCtrl_4.Controls.Add(emailStr);
boxEmail = new TextBox
{
CssClass = "finaff-webform-field-input"
};
cellTxtbx_4.Controls.Add(boxEmail);
dynamicTable.Rows.Add(row4);
return dynamicTable;
}
}
Show Some Exuberance
If you find this tip helpful, jump up and click your heels together, as if you had just quit the tobacco habit, or bounce around your neighborhood on a pogo stick while alternately grinning and laughing, or run around your house thinking of the phrase "Siberian Tiger Milk Dudley Do-right the Wrongs!" or skip down the corridor at work whistling "Zippe-Dee-Doo-Dah!"
I am in the process of morphing from a software developer into a portrayer of Mark Twain. My monologue (or one-man play, entitled "The Adventures of Mark Twain: As Told By Himself" and set in 1896) features Twain giving an overview of his life up till then. The performance includes the relating of interesting experiences and humorous anecdotes from Twain's boyhood and youth, his time as a riverboat pilot, his wild and woolly adventures in the Territory of Nevada and California, and experiences as a writer and world traveler, including recollections of meetings with many of the famous and powerful of the 19th century - royalty, business magnates, fellow authors, as well as intimate glimpses into his home life (his parents, siblings, wife, and children).
Peripatetic and picaresque, I have lived in eight states; specifically, besides my native California (where I was born and where I now again reside) in chronological order: New York, Montana, Alaska, Oklahoma, Wisconsin, Idaho, and Missouri.
I am also a writer of both fiction (for which I use a nom de plume, "Blackbird Crow Raven", as a nod to my Native American heritage - I am "½ Cowboy, ½ Indian") and nonfiction, including a two-volume social and cultural history of the U.S. which covers important events from 1620-2006: http://www.lulu.com/spotlight/blackbirdcraven