In this tip, we are going to talk about Angular2 forms. Forms are really important to collect the data from the users. Each website has forms to collect the data from the user, let’s say our website has registration form to collect the information from the users, we need to validate form data as well before submitting the data.
Angular provides the two different ways to collect and validate the data from the user:
- Template driven forms
- Model driven forms
In this tip, we will learn about how to use and validate template driven forms. I am using bootstrap classes to apply some basic style to form elements, to give visual indication to the user about the controls validity.
Let's open template-form.component.html and paste the following code to render simple form.
HTML Code
<div class="container">
<form class="form-horizontal" #userForm="ngForm" (ngSubmit)="submitForm(userForm.value)" novalidate>
<div class="form-group">
<label class="control-label col-sm-2" for="email">First Name:</label>
<div class="col-sm-10">
<input type="text" #firstName="ngModel" minlength="5"
maxlength="10" class="form-control" required placeholder="Enter first name"
name="firstName" ngModel>
<div *ngIf="firstName.errors && firstName.dirty">
<div class="alert alert-danger"
*ngIf="firstName.errors.required">Name is required</div>
<div class="alert alert-danger"
*ngIf="firstName.errors.minlength">Name should have minimum 5 characters</div>
</div>
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="pwd">Email:</label>
<div class="col-sm-10">
<input type="text" class="form-control"
placeholder="Enter email" name="email" ngModel>
</div>
</div>
<div ngModelGroup="address">
<div class="form-group">
<label class="control-label col-sm-2" for="pwd">Country:</label>
<div class="col-sm-10">
<input type="text" class="form-control"
placeholder="Enter country" name="country" ngModel>
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="pwd">City:</label>
<div class="col-sm-10">
<input type="text" class="form-control"
placeholder="Enter city" name="city" ngModel>
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="pwd">Postal Code:</label>
<div class="col-sm-10">
<input type="text" class="form-control"
#potalCode pattern="^[0-9][1-9]{4}$" placeholder="Enter postal code"
name="postalCode"
ngModel>
<div *ngIf="potalCode.errors && potalCode.dirty">
<div class="alert alert-danger"
*ngIf="potalCode.errors.pattern">Please enter proper postal code</div>
</div>
</div>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<button type="submit" class="btn btn-primary"
[disabled]="!userForm.valid">Submit</button>
</div>
</div>
</form>
</div>
HTML output screenshot:
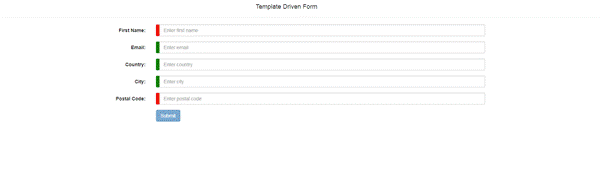
In the HTML code, as you can see that I have used form tag at the top to declare the form, see the below code:
<form class="form-horizontal" #userForm="ngForm" (ngSubmit)="submitForm(userForm.value)" novalidate>
I have created the reference variable userForm
using ngForm
inbuilt directive to refer the form.
Whenever user clicks on the submit button of form, ngSubmit
event gets fired and here I am passing the form values to submitForm()
function using the reference variable. And submitForm()
function is present in .ts file.
And you can see that I am using ngModel
inbuilt directive to register input controls and its value with the form. See below code.
<input type="text" #firstName="ngModel" minlength="5" maxlength="10"
class="form-control" required placeholder="Enter first name" name="firstName" ngModel>
For Adding Validation
In the above form, I have also added some basic validations, i.e., required, minlength. So I am adding validation messages/css classes to the form control based on controls validity. Angular by default adds some classes to controls based on controls state.
State | True | False |
Valid | ng-valid | ng-invalid |
Controls value changed | ng-dirty | ng-pristine |
Control is visited | ng-touched | ng-untoched |
So we can easily use these classes to apply some basic validations/css styling to form element in template driven forms.
See the below code:
<div class="form-group">
<label class="control-label col-sm-2" for="email">First Name:</label>
<div class="col-sm-10">
<input type="text" #firstName="ngModel" minlength="5" maxlength="10"
class="form-control" required placeholder="Enter first name"
name="firstName" ngModel>
<div *ngIf="firstName.errors && firstName.dirty">
<div class="alert alert-danger" *ngIf="firstName.errors.required">Name is required</div>
<div class="alert alert-danger" *ngIf="firstName.errors.minlength">
Name should have minimum 5 characters</div>
</div>
</div>
</div>
I have added firstName
as a reference variable to the control and I’m using errors property to check the errors of the controls and based on the condition I’m showing different error messages.
.ts File Code
import { Component } from '@angular/core'
@Component({
selector: 'my-app',
templateUrl: './templatedrivenforms/template-form.component.html',
styles: [
`input.ng-valid{ border-left:10px solid green }
input.ng-invalid { border-left:10px solid red }`
]
})
export class TemplateFormComponent {
submitForm(values: any) {
console.log(values);
}
}
Now refresh the browser and fill all the required details:

Click submit and check the console log as shown below:

Once we click on submit button, it has called that submitForm
function and console log is as above.
In our next article, we will learn about Model driven forms.
Hope this will help you. Thanks!
You can download the complete code from by github repository using the below link:
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.