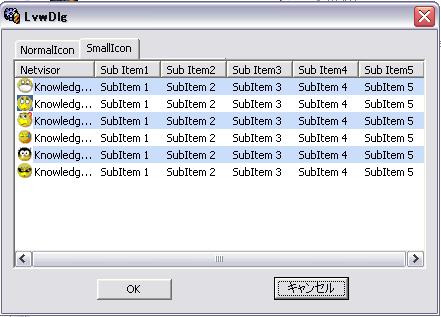
Introduction
This program uses a listview in a dialog, by changing the tab, it can reappear in report style and icon style.
It explains these problems in using listview:
- how can you change the listview's style
- how to get the data that item you clicked;
- how to remove the icon in every item
- how to change bk-color and text color in every item
- how to disable the mouse left and right key
Background
I received a project, and had to code a win32 dialog using listview. But when I searched the web, there are
so few articles and code, which was using listview in win32. Almost of them are in MFC.
Using the code
BOOL CALLBACK TableProc(HWND hDlg, UINT message,
WPARAM wParam, LPARAM lParam)
{
int iIndex;
LPNMLISTVIEW pnm;
TCHAR *pVarName = NULL;
POINT pt;
static RECT lstRect;
switch(message)
{
case WM_INITDIALOG:
SendMessage(hDlg, WM_SETREDRAW, FALSE, 0);
hListTab = GetDlgItem(hDlg, IDC_LISTTAB);
InitListTab(hListTab);
hTableList = GetDlgItem(hDlg, IDC_TABLELIST);
InitTableImageList(hTableList);
InitTableList(hTableList);
InitTableDlg(hDlg);
SetFocus(hTableList);
SendMessage(hTableList, WM_SETREDRAW, TRUE, 0);
GetWindowRect(hTableList, &lstRect);
return TRUE;
case WM_COMMAND:
if(LOWORD(wParam) == IDOK || LOWORD(wParam) == IDCANCEL)
{
PostQuitMessage(0);
EndDialog(hDlg, 0);
return TRUE;
}
break;
case WM_NCHITTEST:
pt.x = LOWORD(lParam);
pt.y = HIWORD(lParam);
if(pt.x >= lstRect.left && pt.x <= lstRect.right &&
pt.y >= lstRect.top && pt.y <= lstRect.right)
{
return (LRESULT)HTERROR;
}
break;
case WM_NOTIFY:
switch(LOWORD(wParam))
{
case IDC_TABLELIST:
pnm = (LPNMLISTVIEW)lParam;
if(pnm->hdr.hwndFrom == hTableList &&pnm->hdr.code == NM_CUSTOMDRAW)
{
SetWindowLong(hDlg, DWL_MSGRESULT, (LONG)TableDraw(lParam));
return TRUE;
}
if(((LPNMHDR)lParam)->code == NM_CLICK)
{
iIndex = (int)SendMessage(hTableList, LVM_GETNEXTITEM, -1, LVNI_FOCUSED);
if(iIndex == -1)
return FALSE;
TCHAR itemTotle[MAX_PATH] = {0};
GetItemText(hTableList, iIndex, itemTotle);
return FALSE;
}
if(((LPNMHDR)lParam)->code == LVN_ITEMCHANGED)
{
iIndex = (int)SendMessage(hTableList, LVM_GETNEXTITEM, -1, LVNI_FOCUSED);
if(iIndex == -1)
return FALSE;
ListView_SetItemState(hTableList, iIndex, 0, LVIS_SELECTED | LVIS_FOCUSED);
return TRUE;
}
break;
case IDC_LISTTAB:
if(((LPNMHDR)lParam)->code == TCN_SELCHANGE)
{
OnSelchangeListCtrlMode(hDlg);
return TRUE;
}
break;
}
break;
}
return FALSE;
}
Updates
I will keep a running update of any changes or improvements to this simple
program.
I think it must be useful to those who want to use listview in Win32.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.