This example shows you how to use VB.NET to mimic the Excel Slope (known_ys, known_xs) and Intercept (known_ys, known_xs) functions in code. It has a simplified snippet of code that has the bare basics, then a more robust version which contains the DataGridView form.
Introduction
When it is needed to programatically calculate Linear Regression Slope and Intercept statistical values, you need to do it manually since VB.NET doesn't have many math features built in.
Program form
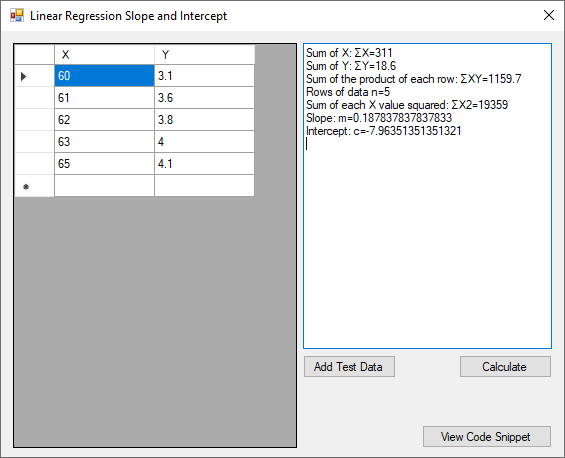
Background
I went looking for how to calculate linear regression slope and intercept in a program I am writing and since I have many years between me and math formulas, it was a bit of a challenge. I looked around for an example that would break down the problem into chunks for me to understand and implement into code but didn't find much.
Using the Code
Here is the basic function. The resultant variables, m
and c
, will contain the slope and the intercept.
To use code this as it is here:
- Create a new "Windows Forms App (.NET Framework)" Visual Basic project in Visual Studio.
- Add one text box to the form.
- Set the text box to Multiline by clicking the ">" in the upper right corner and checking Multiline.
- Make the text box bigger by dragging its edges.
- Double click the title bar of the form to open the "
FormX_load
" function. - Paste this in and run it.
Dim X As New List(Of Double)
Dim Y As New List(Of Double)
Dim c As Double
Dim N As Integer
Dim ΣXY As Double
Dim m As Double
Dim ΣX As Double
Dim ΣY As Double
Dim ΣX2 As Double
X.Add(60)
X.Add(61)
X.Add(62)
X.Add(63)
X.Add(65)
Y.Add(3.1)
Y.Add(3.6)
Y.Add(3.8)
Y.Add(4)
Y.Add(4.1)
N = X.Count
ΣX = X.Sum
ΣY = Y.Sum
ΣXY = 0
ΣX2 = 0
For i = 0 To N - 1
ΣXY += X(i) * Y(i)
Next
For i = 0 To N - 1
ΣX2 += X(i) ^ 2
Next
m = ((N * ΣXY) - (ΣX * ΣY)) / ((N * ΣX2) - (ΣX ^ 2))
c = (ΣY - m * ΣX) / N
TextBox1.Text &= "Sum of X: ΣX=" & ΣX & vbCrLf
TextBox1.Text &= "Sum of Y: ΣY=" & ΣY & vbCrLf
TextBox1.Text &= "Sum of the product of each row: ΣXY=" & ΣXY & vbCrLf
TextBox1.Text &= "Rows of data n=" & N & vbCrLf
TextBox1.Text &= "Sum of each X value squared: ΣX2=" & ΣX2 & vbCrLf
TextBox1.Text &= "Slope: m=" & m & vbCrLf
TextBox1.Text &= "Intercept: c=" & c & vbCrLf
The code sample in the zip contains a working program which uses a DataGridView
to populate the values. It also contains a second form with this exact snippet of code.
Here is what this snippet should look like when done:

Here is what the code of the snippet looks like in Visual Studio:

Points of Interest
I used this site to get a working model of this formula going.
You will see the same dataset
represented in my downloadable code so you can test the results.
I also included an Excel spreadsheet with the formulas and same data.
History
- 24th January, 2022: Initial version
- 24th January, 2022: Applying editor suggestions
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.