Introduction to Angular
So what is Angular, and why would you want to use it? The simplest answer to that question is that Angular is a JavaScript library, but it's so much more than that. It's probably more accurate to categorize it as an MV* framework. Angular isn't just an MV* framework, it's an opinionated MV* framework. What is opinionated software? Well, opinionated software guides you into certain ways of doing things. Opinionated software has a vision. It may limit itself to solving fewer problems, but generally, software with opinions solves those problems with less fuss. That doesn't mean that there isn't more than one way to solve a problem with Angular. For example, take DOM manipulation. Angular wants you to only manipulate your DOM inside of directives, but with Angular, inside of a directive you can use jQuery, Zepto, any other library, or even just raw JavaScript to manipulate your DOM. That doesn't mean that you can't manipulate the DOM from within any other piece of an Angular application, but Angular definitely has an opinion about whether or not you should, and it gently guides you into doing things a certain way.
Let’s make sure that we understand exactly what an MV* framework is.
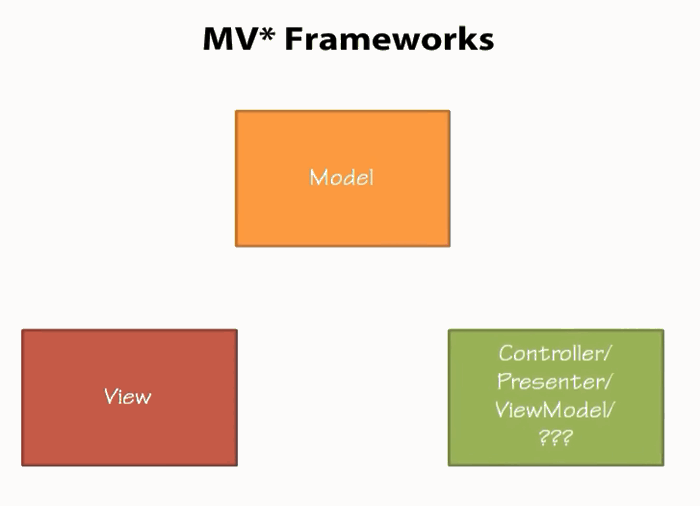
The M in MV* stands for Model. The Model is where you store the data and state of your application. The V in MV* stands for View. The View is where you actually render to the user the information that you want them to see and the View is where you receive input from the user. The * in MV* stands for, well, something else. In many common MV* frameworks, the star is either a Controller or a Presenter or a ViewModel, or even something different besides those three. In fact, some frameworks even allow you to choose one of those three, and don't necessarily prescribe which one. Now you may be asking yourself, which one of those does Angular use? Well, Angular uses a Controller, so some people may call Angular an MVC framework. That would definitely be true, but in the web world there are so many frameworks that fall within this categorization, but don't necessarily fit into just MVC, that this term MV* is commonly used. Angular is an open source library built by the folks at Google.
Angular Architecture
Let's take a look at some of the architectural choices that Angular has made. First, Angular supports two-way binding. This means the user input into form fields is instantly updated in your Angular models. That means that in most cases you don't need to watch for specific events and respond to them and then manually update your HTML. Instead, Angular will handle that for you. Angular also employs a technique called dirty checking. The net result of this is that you don't have to put your data into special structures and call getter and setter methods to read and write to your data. You can simply put your model data into plain old JavaScript objects and Angular will respond whenever your data changes, and automatically update your view. Lastly, Angular is built on Dependency Injection. This lets you encapsulate pieces of your application better and also improves testability.
With Angular, everything starts with the Controller. The Controller is the central player in an Angular application. Controllers contain both logic and state. Next we have the View. Views are made up of bindings and directives. This is how Angular talks to and listens to the user. Controllers can communicate with Views through both one-way and two-way binding. Directives, which are a heavily talked about piece of Angular, are really just part of the View. And the last major piece is services. Services give you a place to contain the real logic and state of your application. If you think about what is the essential tasks of your application, this would likely happen in your services. Complex business logic, important application state, etc., services are the place to house all that. Also, services are the place where you will want to communicate with the server.
Controllers and Scope
We’re going to talk about Controllers, but we can't talk about Controllers without talking about Scope. So, let's look at the relationship between Controllers and Scope. A controller's primary responsibility is to create a scope object. A scope object is how we communicate with the view and the scope is able to communicate with the view through two-way communication. The view can bind to properties and results of functions on the scope and events on the view can call methods on the scope. Data passes in this way from the controller to the scope and from the scope back and forth to the view. The scope is used to expose the model to the view, but the scope is not the model. The model is the data that is put into the scope. If we want to modify the model, we can either use methods that are on the scope to modify the model, perhaps in response to events fired by the view or using two-way bindings we can modify the model. In this way, users, through the view can make modifications to the model or in other words can make modifications to the data.
Let’s Code
Well as common all bloggers who use to create Hello World application, let’s change the trend we are going to build addition application, where two text boxes accept the values and add them and show result in label.
HTML

In the above HTML, I have use ng-app
, ng-controller
, ng-model
and special sign {{ }} which we call delimiter angular use this to evaluate the expression. Now you have some question what is ng-app
and other ng-
? They are build-in directives. So, now let's talk about what directives are. According to the Angular documentation, directives are a way to teach HTML new tricks. Essentially, directives give HTML new functionality. We will talk about directives in the next blog.
Alright, let’s talk about ng-app
directive is used to auto bootstrap the application. Only one AngularJs application can use this per HTML page. Most commonly, this can use at <HTML>
, <BODY>
tag of the page. We use this on <BODY>
tag.
The directive ng-controller
, notifies the angular engine that this part of DOM will control by this controller and local scope will handled.
ng-model
is used for binding the control with the model values, which can show the updated value of the attached property and update at the same time.
In our application, we use ng-app=”calc”
and ng-controller=”calculatorController”
also ng-model="calc.firstNumber"
.
JS

While looking into the above code snippet, you can notice that I have used angular.module
. Module has the ability to isolate different parts of the application like controller, factory, services and directives.
I have created calc
module which contains controller for this application. I use $scope
which holds calc
model for the application.
See this working @ http://codepen.io/anon/pen/eNKjeB.
Summary
In this blog, we started by taking a look at JavaScript MV* frameworks, some of the various options there, and how Angular fits into that world. Then we looked at some of the benefits of AngularJS, such as it being forward thinking and very testable. After that, we took a brief look at the application that we will be building.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.