Factory Method belongs to Creational Patterns and it is useful when you have a set of classes performing a similar operation, but the exact class to be used to get the job done is dependent on some logic or condition.
As the name suggests, the factory method pattern defines a way to create an object. And the subclasses decide which class to instantiate. How do these subclasses decide which class to instantiate?
This is quite application specific, and may involve some programmatic logic or configuration setting.
Problem
Suppose you design an application to render a specific type of chart and your library has to receive a set of records to render, but you don't know what type of chart you are going to receive, as a consequence, you don´t know what type of chart you are going to render, how do you deal with it ?
Design
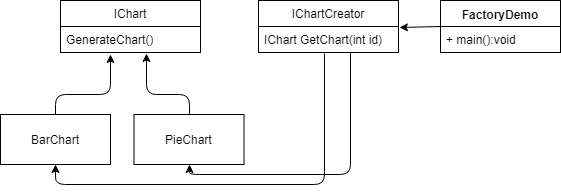
Solution
Factory Method comes to the rescue with this type of requirement.
Steps
The first that you must create is an interface IChart
that defines GenerateChart()
method.
The second is creating the class that will implement this Interface IChart
: BarChart
and PieChart
.
After that, create an Interface called IChartCreator
which is responsible for returning the proper Chart
according to a specific TypeOfChart
.
Code in Console
class Program
{
static void Main(string[] args)
{
ChartCreator chartCreator = new ChartCreator();
for (int i = 1; i <= 2; i++)
{
var chart = chartCreator.GetChart(i);
Console.WriteLine("Where id = {0}, chart = {1} ", i, chart.GenerateChart());
}
Console.ReadKey();
}
}
public interface IChart
{
string GenerateChart();
}
public class BarChart : IChart
{
public string GenerateChart()
{
return "Generating BarChart.";
}
}
public class PieChart : IChart
{
public string GenerateChart()
{
return "Generating PieChart.";
}
}
public interface IChartCreator
{
IChart GetChart(int id);
}
public class ChartCreator : IChartCreator
{
public IChart GetChart(int id)
{
IChart chart = null;
switch (id)
{
case (int)ChartType.BarChart:
chart = new BarChart();
break;
case (int)ChartType.PieChart:
chart = new PieChart();
break;
}
return chart;
}
}
public enum ChartType
{
BarChart = 1,
PieChart = 2
}
Conclusion
Factory classes are often implemented because they allow the project to follow the SOLID principles more closely. In particular, the interface segregation and dependency inversion principles.
Factory method is often used in the next situations:
- A class cannot anticipate the type of objects it needs to create beforehand.
- A class requires its subclasses to specify the objects it creates.
- You want to localize the logic to instantiate a complex object.
Code Running in .NET Fiddle
Find this code running here.
I am Software Developer with a passion for technology with strong backend (PHP, MySQL, PostgreSQL and SQL Server) and frontend (HTML5, CSS3, AngularJs and KnockOutJs) experience. I combine my education with expertise in CSS3, HTML5, Javascript to build creative and effective websites and applications.
If you wish to contact me then please do so on ricardojavister@gmail.com or feel free to skype me on
ricardo-torres-torres.