Introduction
Partial views are just Views which you can reuse across your ASP.NET MVC application. If you are from ASP.NET Web Forms background, you can think of Partial View as User Control. Partial views can contain anything – HTML elements for displaying the data or getting the input from the users. In this tip, we are going to see the different ways to pass data to partial view for displaying data to the partial view.
As views are like partial views, you can pass data to partial view as though passing data to the view. Below are few of the methods which you can pass data to Partial View.
- Pass data from enclosing View to Partial View
- Pass data to Partial View using
ViewBag
/ViewData
- Pass data to Partial View using
TempData
- Pass data to Partial View using strongly typed model
Before discussing about each of the methods in detail, let us talk about creating the application.
Create ASP.NET MVC project using Microsoft Visual Studio Express 2013 for the web – choosing Empty template and checking MVC as type of project. Create a Controller by name HomeController
and Index
action method would be added by default as below.
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
}
Right click inside the Index
action method and select “Add View” from the contextual menu – This will add Index.cshtml with the following content:
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
Create a Partial View by right clicking the Views\Shared folder and select Add -> MVC 5 Partial Page (Razor) from the contextual menu.
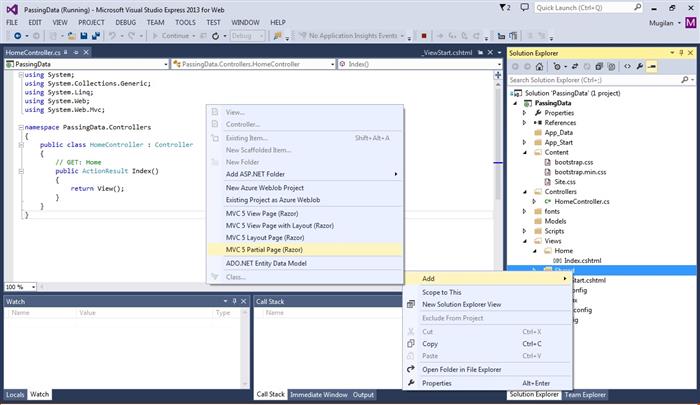
I name this partial view as _MyPartial.cshtml as by convention, name of the partial view would start with underscore(_)
.
We are done with the setup. Let us discuss each one of the above mentioned methods for passing data to partial view in detail now.
1. Pass Data from Enclosing View to Partial View
You can initiate a variable in View using Razor and pass that variable to Partial View. I’ve made couple of changes in Index.cshtml.
- Creating a variable
piValue
of type double
- Calling
PartialView
by passing the newly created variable piValue
to @Html.Partial
method. @Html.Partial
is the helper method for displaying the partial View
@{
ViewBag.Title = "Index";
double piValue = 3.14;
}
<h2>Index</h2>
@Html.Partial("_MyPartial", piValue)
In the partial view (_MyPartial.cshtml), I can consume the passed variable value by accessing @Model
variable.
Data received is: @Model
When you run the application, you’ll get the following result:
Data received is : 3.14
2. Pass Data to Partial View using ViewBag/ViewData
You can use ViewData
/ViewBag
to pass the information from the controller’s action method to the View. ViewData
uses ViewDataDictionary
and ViewBag
is just a wrapper around ViewData
using dynamic feature.
In the below Index
action method, I am passing the piValue
value from action method to the View.
public ActionResult Index()
{
ViewBag.piValue = 3.14;
return View();
}
In the View(Index.cshtml)
, I am passing the piValue
to the partial view. The important point to note here is that @Html.Partial
method does not accept the dynamic value – so we need to cast it to the actual type.
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@Html.Partial("_MyPartial", (double) @ViewBag.piValue)
There is no change in the way the Partial View consumes your passed data. As far as Partial view is concerned, it is receiving a data which you can access through @Model
.
Data received is : @Model
When you run the application, you’ll get the same result:
Data received is : 3.14
3. Pass Data to Partial View using TempData
TempData
is another way to pass the data from controller’s action method to View. Tempdata
persists the data even in the case of redirection whereas ViewBag
/ViewData
would not be able to. You can use TempData
just like you use ViewData
– even though the underlying behavior is different.
Below is the Index
action method- where I’ve used TempData
to pass data.
public ActionResult Index()
{
TempData["piValue"] = 3.14;
return View();
}
We are passing the data to the Partial View from the TempData
. There is no need to cast the data as it’s not using dynamic feature like ViewBag
.
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@Html.Partial("_MyPartial", (double) TempData["piValue"])
As usual, there is no change in the way the Partial view consumes your data.
Data received is : @Model
4. Pass Data to Partial View using Strongly Typed Model
To pass the strongly typed model, we need to have the model first. Right click Model folder and Add class file – I have named it as Employee.cs.
It’s a simple class with couple of properties – Name
and Location
.
public class Employee
{
public string Name { get; set; }
public string Location { get; set; }
}
I have created instance of this class and pass it to the View. In the real world, we would be getting the value from database.
public ActionResult Index()
{
var model = new Employee { Name = "John", Location = "New York" };
return View(model);
}
We need to make couple of changes here – first, we need to hint the View what type of data is passed to the View so that we can take advantage of intellisense and strong typing and secondly, we can just use Model
keyword to pass the data to the Partial View.
@model PassingData.Models.Employee
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
@Html.Partial("_MyPartial",Model)
In Partial view as well, we need to inform what type of data we are passing to it. Then, we can access the properties of the class using @Model
.
@model PassingData.Models.Employee
Name : @Model.Name<br/>
Location : @Model.Location
Thanks for your reading the tip. If anything is not clear, please let me know the in the comments section. I’ll reply and update the tip as needed.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.